Vuex的理解和应用
移动开发
2018-04-16 14:39:50
阅读次数: 6
1.Vuex概念
- Vuex 是一个专为 Vue.js 应用程序开发的状态管理模式。
- 通俗的讲,Vue组件中有各种data变量,它就是一个状态,当应用程序足够庞大时,如果将这些状态集中管理就会非常方便,于是Vuex便应运而生。
2. Vuex五大核心
- State(必须)
- State是Vuex中唯一的数据源。在Vuex中定义,可以全局使用,一般在computed计算属性中获取,在页面中渲染。
- 定义:
// store.js
store: {
count: 2
}
// 方法1:正常获取
computed: {
count () {
return this.$store.state.count;
}
}
// 方法2: mapState辅助函数
import { mapState } from 'vuex'
computed: mapSate({
count: state=>state.count, // 箭头函数
count: 'count', // 字符串传参
count (state) { // 使用常规函数
return state.count;
}
})
computed: mapState(['count']) // 也可以传递字符串数组
// 方法3:mapState对象展开式
import { mapState } from 'vuex'
computed: {
...mapState({
count: 'count'
})
}
- Mutations(必须)
- 更改Store中状态的唯一方法是提交Mutaion。
- 定义:
mutations: {
increment (state, n) {
state.count += n;
}
}
// 方法一:直接提交
this.$store.commit('increment', 10);
// 方法二:使用mapState引入
import { mapMutations } from 'vuex'
methods: {
...mapMutations([
'increment', 'add'
])
}
...mapMutations({
'add': 'increment' // 将 `this.add()` 映射为 `this.$store.commit('increment')`
})
- Getters(非必须)
- 通过Getters可以派生出新的状态。
- 通俗的讲,Getters和State在Vuex中扮演着类似的功能,都是状态的集合。假如某一个状态本身被很多组件使用,但是有的地方又要改变这个数据的形式,这是就要使用Getters,比如购物车中的数量(当大于100时显示99+)。Getters在组件中的获取方式和State相同。
- 定义:
getters: {
doneTodos: state=>{
return state.todos.filter(todo => todo.done)
}
}
// 方法一:同Sate类似
return this.$store.getters.doneTodosCount
// 方法二:同Sate类似
import { mapGetters } from 'vuex'
computed: {
...mapGetters(['doneTodosCount', 'anotherGetter'])
}
- Actions(非必须)
- Action提交的是Mutation,而不是直接变更状态,Action可以包含任意异步操作。
- 定义:
// 方式一
actions: {
add (context) {
context.commit('increment');
}
}
// 方式二
actions: {
add ({commit}) {
commit('increment');
}
}
// 方式一:直接分发
this.$store.dispatch('add');
// 方式二:在组件中分发
import { mapActions } from 'vuex'
methods: {
...mapActions(['add', 'other']),
...mapActions({
add: 'increment' // 将 `this.add()` 映射为 `this.$store.dispatch('increment')`
})
}
- Modules(非必须)
- 当应用变得非常复杂时,store 对象就有可能变得相当臃肿。为了解决以上问题,Vuex 允许我们将 store 分割成模块(module)。每个模块都有自己的State、Getters、Mutations、Actions。
- 定义
const moduleA = {
state: { ... },
mutations: { ... },
actions: { ... },
getters: { ... }
}
const moduleB = {
state: { ... },
mutations: { ... },
actions: { ... }
}
const store = new Vuex.Store({
modules: {
a: moduleA,
b: moduleB
}
})
store.state.a // -> moduleA 的状态
store.state.b // -> moduleB 的状态
3.功能和应用

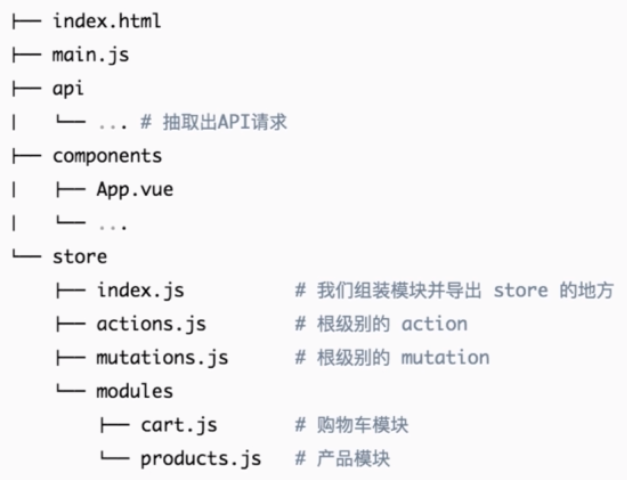
4.优势和缺点
5.应用场景区分
- 如果项目是列表等静态数据较多的小应用,那就用不到状态管理。
- 如果有少量几个状态需要管理的中型应用,可以尝试使用eventBus,这样更简洁。
- 如果有几个以上的状态需要随时修改和改变状态,比如购物车数量、登录状态等,可以使用Vuex,这样会更方便。
转载自my.oschina.net/chinahufei/blog/1630972