对servlet支持,cookie
<body>
<form action="user/login.do" method="post">
<table>
<tr>
<td>用户名:</td>
<td><input type="text" name="userName"/></td>
</tr>
<tr>
<td>密码:</td>
<td><input type="password" name="password"/></td>
</tr>
<tr>
<td>
<input type="submit" value="登录"/>
</td>
</tr>
</table>
</form>
</body>
<body>
Main.jsp ${currentUser.userName }
</body>
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import com.java1234.model.User;
@Controller
@RequestMapping("/user")
public class UserController {
@RequestMapping("/login")
public String login(HttpServletRequest request,HttpServletResponse response){
System.out.println("----登录验证---");
String userName=request.getParameter("userName");
String password=request.getParameter("password");
Cookie cookie=new Cookie("user",userName+"-"+password);
cookie.setMaxAge(1*60*60*24*7);
User currentUser=new User(userName,password);
response.addCookie(cookie);
HttpSession session=request.getSession();
session.setAttribute("currentUser", currentUser);
return "redirect:/main.jsp";
}
}
使用request或sesion
@RequestMapping("/login2")
public String login2(HttpServletRequest request){
return "redirect:/main.jsp";
}
@RequestMapping("/login3")
public String login3(HttpSession session){
return "redirect:/main.jsp";
}
springMvc对json的支持
配置文件bean.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<!-- 使用注解的包,包括子集 -->
<context:component-scan base-package="com.java"/>
<!-- 支持对象与json的转换。 -->
<mvc:annotation-driven/>
<!-- 视图解析器 -->
<bean id="viewResolver"
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/jsp/" />
<property name="suffix" value=".jsp"></property>
</bean>
</beans>
@ResponseBody返回user的json对象
@RequestMapping("/ajax")
public @ResponseBody User ajax(){
User user=new User("zhangsan","123");
return user;
}
util
object o为传入的json对象
import java.io.PrintWriter;
import javax.servlet.http.HttpServletResponse;
public class ResponseUtil {
public static void write(HttpServletResponse response,Object o)throws Exception{
response.setContentType("text/html;charset=utf-8");
PrintWriter out=response.getWriter();
out.println(o.toString());
out.flush();
out.close();
}
}
扫描二维码关注公众号,回复:
2886047 查看本文章
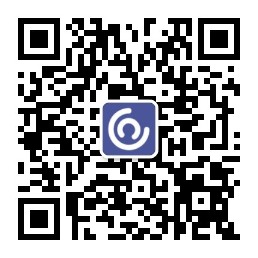