The little cat is so famous, that many couples tramp over hill and dale to Byteland, and asked the little cat to give names to their newly-born babies. They seek the name, and at the same time seek the fame. In order to escape from such boring job, the innovative little cat works out an easy but fantastic algorithm:
Step1. Connect the father's name and the mother's name, to a new string S.
Step2. Find a proper prefix-suffix string of S (which is not only the prefix, but also the suffix of S).
Example: Father='ala', Mother='la', we have S = 'ala'+'la' = 'alala'. Potential prefix-suffix strings of S are {'a', 'ala', 'alala'}. Given the string S, could you help the little cat to write a program to calculate the length of possible prefix-suffix strings of S? (He might thank you by giving your baby a name:)
Input
The input contains a number of test cases. Each test case occupies a single line that contains the string S described above.
Restrictions: Only lowercase letters may appear in the input. 1 <= Length of S <= 400000.
Output
For each test case, output a single line with integer numbers in increasing order, denoting the possible length of the new baby's name.
Sample Input
ababcababababcabab aaaaa
Sample Output
2 4 9 18 1 2 3 4 5
题目大意:给你一组字符串,当这组字符串的前缀和后缀相同时, 输出其前缀的长度。
大致思路:首先我们要明白KMP算法中next数组的作用,next[i]表示在字符串A中,以i为结尾的非前缀子串与A的前缀能够匹配的最长长度。
假设字符串的长度为len,那么next[len]就表示字符串的后缀与A的前缀的匹配的最长长度。我们可以从这一步得到A的前缀串长度,然后在在next[len]中去查找下一个前缀串,因为下一个前缀串一定落在next[len]中,按找上一步的方法继续去求解与之相匹配的后缀串的长度。这个时候我们要知道上一步求的前缀串一定与后缀串想等,所以在next[len]中求解的的后缀串在原字符串中一定能查找到。然后我们就按找这个思路递归查找。知道找到头为止。同时注意字符串A的前缀和后缀的最大匹配为它自己。
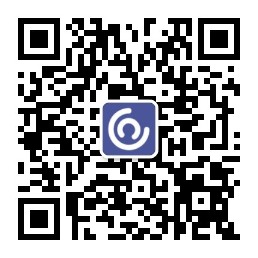
如果无法理解的话我们可以结合这幅图片理解:
图中黑色部分表示原字符串,红色部分表示next[len],蓝色部分表示next[next[len]]。
Talk is cheap,show me the code!
#include <algorithm>
#include <iostream>
#include <cstdio>
#include <cstring>
using namespace std;
const int MAXN=1e6+10;
int next[MAXN],sum[MAXN];
char a[MAXN];
int n;
void getNext(){
next[1]=0;
for(int i =2, j = 0;i <= n; i++){
while(j > 0 && a[i] != a[j+1]) j = next[j];
if(a[i] == a[j+1]) j++;
next[i]=j;
}
}
int main(){
while(~scanf("%s",a+1)){
n = strlen(a+1);
int k = 0;
getNext();
for(int i = next[n];i != 0; i = next[i]){
sum[k++] = i;
}
for(int i = k-1; i >= 0;i--){
printf("%d ",sum[i]);
}
printf("%d\n", n);
}
return 0;
}