记一记:
加入相关依赖后。。。
【简单的】
相关的spring配置文件:T3.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:rabbit="http://www.springframework.org/schema/rabbit"
xsi:schemaLocation="http://www.springframework.org/schema/rabbit
http://www.springframework.org/schema/rabbit/spring-rabbit.xsd
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<rabbit:connection-factory id="connectionFactory" host="localhost" virtual-host="/ywj" username="ywj" password="0"/>
<rabbit:template id="rabbitTemplate" connection-factory="connectionFactory" message-converter="jackson2JsonMessageConverter"/>
<rabbit:admin connection-factory="connectionFactory"/>
<!--一个队列-->
<rabbit:queue name="testQueue" auto-delete="true" durable="false"/>
<!--json序列化-->
<bean id="jackson2JsonMessageConverter" class="org.springframework.amqp.support.converter.Jackson2JsonMessageConverter"/>
</beans>
相关的java代码:
package com.example.java.t0;
import com.rabbitmq.client.AMQP;
import com.rabbitmq.client.impl.AMQImpl;
import org.springframework.amqp.core.Queue;
import org.springframework.amqp.rabbit.connection.ConnectionFactory;
import org.springframework.amqp.rabbit.core.RabbitAdmin;
import org.springframework.amqp.rabbit.core.RabbitTemplate;
import org.springframework.context.support.AbstractApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class T3 {
public static void main(String[] args) throws InterruptedException {
AbstractApplicationContext ctx =
new ClassPathXmlApplicationContext("T3.xml");
RabbitTemplate template = ctx.getBean(RabbitTemplate.class);
template.convertAndSend("testQueue", new Order(1L,"order0"));
System.out.println("发送成功");
Thread.sleep(1000);
Object o = template.receiveAndConvert("testQueue");
Order order = (Order) o;
System.out.println("接收:"+order.getName());
ctx.destroy();
}
}
【work模式】:
spring配置文件如上的T3.xml.
发送者:
package com.example.java.t0;
import com.rabbitmq.client.AMQP;
import com.rabbitmq.client.impl.AMQImpl;
import org.springframework.amqp.core.Queue;
import org.springframework.amqp.rabbit.connection.ConnectionFactory;
import org.springframework.amqp.rabbit.core.RabbitAdmin;
import org.springframework.amqp.rabbit.core.RabbitTemplate;
import org.springframework.context.support.AbstractApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class T3 {
public static void main(String[] args) throws InterruptedException {
AbstractApplicationContext ctx =
new ClassPathXmlApplicationContext("T3.xml");
RabbitTemplate template = ctx.getBean(RabbitTemplate.class);
for(int i = 0; i < 20; i ++){
template.convertAndSend("testQueue", "msg "+i+" "+System.currentTimeMillis());
}
System.out.println("发送成功");
ctx.destroy();
}
}
接收者1:
package com.example.java.t0;
import org.springframework.amqp.rabbit.core.RabbitTemplate;
import org.springframework.context.support.AbstractApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class T3_1 {
public static void main(String[] args) throws InterruptedException {
AbstractApplicationContext ctx =
new ClassPathXmlApplicationContext("T3.xml");
RabbitTemplate template = ctx.getBean(RabbitTemplate.class);
while (true){
Thread.sleep(1000);
System.out.println("1接收:"+template.receiveAndConvert("testQueue"));
}
}
}
接收者2同上
【topic模式】:
扫描二维码关注公众号,回复:
3213703 查看本文章
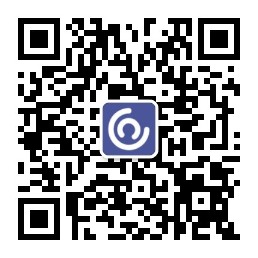
spring.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:rabbit="http://www.springframework.org/schema/rabbit"
xsi:schemaLocation="http://www.springframework.org/schema/rabbit
http://www.springframework.org/schema/rabbit/spring-rabbit.xsd
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<rabbit:connection-factory id="connectionFactory" host="localhost" virtual-host="/ywj" username="ywj" password="0"/>
<rabbit:template id="amqpTemplate" connection-factory="connectionFactory" exchange="topicExchange" routing-key="nokia.8plus"/>
<rabbit:admin connection-factory="connectionFactory" />
<rabbit:queue name="appleQueue" auto-delete="true" durable="false"/>
<rabbit:queue name="nokiaQueue" auto-delete="true" durable="false"/>
<rabbit:topic-exchange name="topicExchange" auto-delete="true" durable="false">
<rabbit:bindings>
<rabbit:binding queue="appleQueue" pattern="apple.*" />
<rabbit:binding queue="nokiaQueue" pattern="nokia.*" />
</rabbit:bindings>
</rabbit:topic-exchange>
<rabbit:listener-container connection-factory="connectionFactory">
<rabbit:listener ref="apple" method="listen" queue-names="appleQueue" />
<rabbit:listener ref="nokia" method="listen" queue-names="nokiaQueue" />
</rabbit:listener-container>
<bean id="apple" class="com.example.java.t0.Apple" />
<bean id="nokia" class="com.example.java.t0.Nokia" />
</beans>
发送者:
package com.example.java.t0;
import org.springframework.amqp.rabbit.core.RabbitTemplate;
import org.springframework.context.support.AbstractApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Test {
public static void main(String[] args) throws InterruptedException {
AbstractApplicationContext ctx =
new ClassPathXmlApplicationContext("context.xml");
RabbitTemplate template = ctx.getBean(RabbitTemplate.class);
template.convertAndSend("买买买!");
Thread.sleep(1000);
ctx.destroy();
}
}
接收者apple:
package com.example.java.t0;
public class Apple {
public void listen(String msg)
{
System.out.println("Apple接收到的信息:"+msg);
}
}
接收者nokia:
package com.example.java.t0;
public class Nokia {
public void listen(String msg)
{
System.out.println("Nokia接收到的信息:"+msg);
}
}
spring.xml中可以去掉:<rabbit:template/>中的routing-key,在发送中设置:template.setRoutingKey("apple.8plus");
注意:spring rabbit消息接收后手动确认回复的方法:
配置文件:加上 acknowledge="manual"
<rabbit:listener-container connection-factory="connectionFactory" acknowledge="manual">
<rabbit:listener ref="apple" queue-names="appleQueue" />
<rabbit:listener ref="nokia" queue-names="nokiaQueue" />
</rabbit:listener-container>
然后接收者:
public class Nokia implements ChannelAwareMessageListener {
public void onMessage(Message message, Channel channel) throws Exception {
System.out.println(new String(message.getBody())+"===============================Nokia");
channel.basicAck(message.getMessageProperties().getDeliveryTag(), false);// 手动确认回复
}
}
先记这么多先。。。