Hbase提供了JavaApi来进行操作,以下是笔者以JavaApi的集中方式对Hbase进行操作:
条件查询:Scan
public static void main(String[] args) {
try(Connection conn = ConnectionFactory.createConnection()){ //通过Hbase中Connection
//这种写法是JDK7以后的新特性
Scan scan = new Scan();//使用Scan
scan.setFilter(new SingleColumnValueFilter(Bytes.toBytes("info"),Bytes.toBytes("sex"),
CompareFilter.CompareOp.EQUAL,Bytes.toBytes("m")));
//条件查询 查询列族是info中列sex中值为m的一条数据
Table table = conn.getTable(TableName.valueOf("student"));//通过一个表根据Scan去查
ResultScanner rs =table.getScanner(scan);
rs.forEach( t->{
System.out.println(t);
System.out.println(Bytes.toString(t.getRow()));
//获取RowKey 转为String
System.out.print(Bytes.toString(t.getValue(Bytes.toBytes("info"),Bytes.toBytes("name"))));
//获取哪个列族下的哪个列:name
System.out.print(Bytes.toString(t.getValue(Bytes.toBytes("info"),Bytes.toBytes("sex"))));
//获取哪个列族下的哪个列:sex
});
}catch (Exception e){
e.printStackTrace();
}finally {
}
}
查看运行结果:
keyvalues={1001/info:name/1537522165984/Put/vlen=4/seqid=0, 1001/info:sex/1537522172814/Put/vlen=1/seqid=0}1001
创建表和列族:
public static void main(String[] args) {
try(Connection conn = ConnectionFactory.createConnection()){
// Table table = conn.getTable(TableName.valueOf("student3"));
//获取表,要创建的表
TableName tableName = TableName.valueOf("student3");
Table table = conn.getTable(tableName);
HTableDescriptor desc = new HTableDescriptor(tableName);
//创建列族
HColumnDescriptor infos = new HColumnDescriptor("info");
desc.addFamily(infos);
HColumnDescriptor adds = new HColumnDescriptor("address");
desc.addFamily(adds);
//创建表
HBaseAdmin admin =(HBaseAdmin) conn.getAdmin();//表的管理类
admin.createTable(desc);
}catch (Exception e){
e.printStackTrace();
}finally {
}
}
查看结果:
hbase(main):016:0> desc 'student3'
Table student3 is ENABLED
student3
COLUMN FAMILIES DESCRIPTION
{NAME => 'address', VERSIONS => '1', EVICT_BLOCKS_ON_CLOSE => 'false', NEW_VERSION_BEHAVIOR => 'false', KEEP_DELETED_CELLS => 'FALSE', CACHE_DATA_ON_WRITE => 'false', DATA_BLOCK_ENCODING => 'NONE', TTL => 'FORE
VER', MIN_VERSIONS => '0', REPLICATION_SCOPE => '0', BLOOMFILTER => 'ROW', CACHE_INDEX_ON_WRITE => 'false', IN_MEMORY => 'false', CACHE_BLOOMS_ON_WRITE => 'false', PREFETCH_BLOCKS_ON_OPEN => 'false', COMPRESSIO
N => 'NONE', BLOCKCACHE => 'true', BLOCKSIZE => '65536'}
{NAME => 'info', VERSIONS => '1', EVICT_BLOCKS_ON_CLOSE => 'false', NEW_VERSION_BEHAVIOR => 'false', KEEP_DELETED_CELLS => 'FALSE', CACHE_DATA_ON_WRITE => 'false', DATA_BLOCK_ENCODING => 'NONE', TTL => 'FOREVER
', MIN_VERSIONS => '0', REPLICATION_SCOPE => '0', BLOOMFILTER => 'ROW', CACHE_INDEX_ON_WRITE => 'false', IN_MEMORY => 'false', CACHE_BLOOMS_ON_WRITE => 'false', PREFETCH_BLOCKS_ON_OPEN => 'false', COMPRESSION =
> 'NONE', BLOCKCACHE => 'true', BLOCKSIZE => '65536'}
2 row(s)
添加数据:
public static void main(String[] args) {
try(Connection conn = ConnectionFactory.createConnection()){
// Table table = conn.getTable(TableName.valueOf("student3"));
//获取表
TableName tableName = TableName.valueOf("student3");
Table table = conn.getTable(tableName);
Put put = new Put(Bytes.toBytes("1001"));//添加rowkey
put.addColumn(Bytes.toBytes("info"),Bytes.toBytes("name"),Bytes.toBytes("zhangsan"));//给列族中某列添加值
put.addColumn(Bytes.toBytes("address"),Bytes.toBytes("address"),Bytes.toBytes("1111111111"));//给列族中某列添加值
table.put(put);
}catch (Exception e){
e.printStackTrace();
}finally {
}
}
查看结果:
hbase(main):017:0> scan 'student3'
ROW COLUMN+CELL
1001 column=address:address, timestamp=1537539582788, value=1111111111
1001 column=info:name, timestamp=1537539582788, value=zhangsan
1 row(s)
Took 0.0428 seconds
添加多条记录:
public static void main(String[] args) {
try(Connection conn = ConnectionFactory.createConnection()){
//获取表
TableName tableName = TableName.valueOf("student3");
Table table = conn.getTable(tableName);
List<Put> putList = new ArrayList<Put>();
Put put1 = new Put(Bytes.toBytes("1002"));
put1.addColumn(Bytes.toBytes("info"),Bytes.toBytes("name"),Bytes.toBytes("lisi"));
put1.addColumn(Bytes.toBytes("info"),Bytes.toBytes("sex"),Bytes.toBytes("nan"));
Put put2 = new Put(Bytes.toBytes("1003"));
put2.addColumn(Bytes.toBytes("info"),Bytes.toBytes("name"),Bytes.toBytes("wangwu"));
put2.addColumn(Bytes.toBytes("address"),Bytes.toBytes("dizhi"),Bytes.toBytes("haidian"));
putList.add(put1);
putList.add(put2);
table.put(putList);
}catch (Exception e){
e.printStackTrace();
}finally {
}
}
查看结果:
hbase(main):019:0> scan 'student3'
ROW COLUMN+CELL
1001 column=address:address, timestamp=1537539582788, value=1111111111
1001 column=info:name, timestamp=1537539582788, value=zhangsan
1002 column=info:name, timestamp=1537540094023, value=lisi
1002 column=info:sex, timestamp=1537540094023, value=nan
1003 column=address:dizhi, timestamp=1537540094023, value=haidian
1003 column=info:name, timestamp=1537540094023, value=wangwu
3 row(s)
Took 0.0223 seconds
删除:
public static void main(String[] args) {
try(Connection conn = ConnectionFactory.createConnection()){
// Table table = conn.getTable(TableName.valueOf("student3"));
TableName tableName = TableName.valueOf("student3");
Table table = conn.getTable(tableName);
//删除rowkey为1002内数据
Delete delete1 = new Delete(Bytes.toBytes("1002"));
table.delete(delete1);
//删除rowkey为1003下列族为address, 以此类推
Delete delete2 = new Delete(Bytes.toBytes("1003"));
delete2.addFamily(Bytes.toBytes("address"));
table.delete(delete2);
}catch (Exception e){
e.printStackTrace();
}finally {
}
}
扫描二维码关注公众号,回复:
3274070 查看本文章
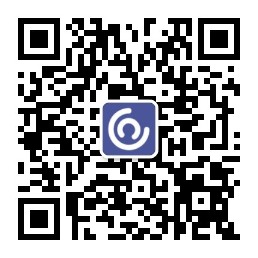