学习如何遍历整个列表,包括包含数千个乃至数百万个元素的列表。
一、遍历整个列表
对列表中的元素都执行相同的操作,在python中使用for循环,在下面的程序中,是将列表magicians中取出一个名字,并将其储存在变量magician中,然后让python打印前面储存在变量magician中的名字,重复执行上述操作。for后面没有其他的代码,程序结束。在for循环中,在命名中,常用单数和复数来区别代码段处理的是单个列表元素韩式整个列表元素。
在for循环中,没有缩进的代码只执行一次,而不会重复操作。
magicians=['alice','david','carolina']
for magician in magicians:
print(magician.title()+",that was a great trick!")
print("I can't wait to see you next trick,"+magician.title()+".\n")
print("Thank you,everyone.That was a great magic show!")
二、避免缩进错误
前面讲缩进的将重复操作,没缩进的只执行一次,但在编写的工程中会犯一些错误,如将不需要的代码块缩进,而将必须缩进的代码块却忘记了。
1、忘记缩进
位于for语句后面且属于循环组成部分的代码行一定要缩进,如果忘记了,python会提醒你:
magicians=['alice','david','carolina']
for magician in magicians:
print(magician)
2、忘记缩进额外的代码行
这种情况循环会进行但不会报错,而结果出乎意料。从语法上python代码是合法的,但存在逻辑错误。
magicians=['alice','david','carolina']
for magician in magicians:
print(magician.title()+",that was a great trick!")
print("I can't wait to see you next trick,"+magician.title()+".\n")
3、不必要的缩进
如果你进行了不必要的缩进,pyhton会指出。
message="Hello python world!"
print(message)
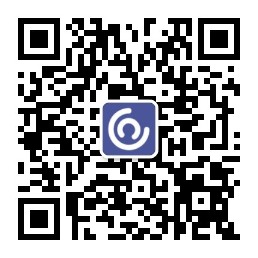
4、循环后不必要的缩进
这些代码将针对每个列表元素重复执行,可能导致python报告语法错误,大多数情况会导致逻辑错误。
magicians=['alice','david','carolina']
for magician in magicians:
print(magician.title()+",that was a great trick!")
print("I can't wait to see you next trick,"+magician.title()+".\n")
print("Thank you,everyone.That was a great magic show!")
5、遗漏了冒号
for语句后面的冒号虽容易消除但难以发现,python不知道你意欲何为。
magicians=['alice','david','carolina']
for magician in magicians
print(magician)
三、创建数值列表
1、使用range()创建数字列表
range()函数经常有差一行的行为,即输出到计数的倒数第二个结束。
for value in range(1,5):
print(value)
要创建数字列表,可使用函数list()将range()的结果直接装换为列表。在使用range()时,可指定步长,range()函数几乎能创建任何需要的数字集。
numbers=list(range(1,7))
print(numbers)
even_numbers=list(range(1,22,3))
print(even_numbers)
squares=[]
for value in range(1,11):
squares.append(value**2)
print(squares)
2、对数字列表进行简单的统计计算
比如找出数字列表中的最小值、最大值以及值得和。
3、列表解析
前面介绍生成列表squares()需要三四行代码,而列表解析只需一行代码就行,列表解析是将for循环和创建新元素的代码合并了,并自动附加新元素。
首先指定一个列表名,如squares,然后指定一个左方括号并定义一个表达式,用于生成一个你要储存到列表中的值,接下来编写一个for循环,用于给表达式提供值,再加上右方括号。
squares=[value**2 for value in range(1,11)]
print(squares)
与前面产生的列表一样,注意这里的for语句不加冒号。
四、使用列表的一部分
1、遍历切片
在python中处理列表的一部分称为切片,在创建切片时,可指定要使用的第一个元素和最后一个元素的索引,和range()函数一样,python将在你指定的第二个索引前面的元素后停止。使用切片可以产生列表的任何子集。
如果你没指定第一个索引,python将自动从列表开头开始,如果你没指定第二个索引,将终止于列表末尾。如果要遍历列表的所有的元素,可在for循环中使用切片。
players=['charles','martina','michael','florence','eli']
print(players[1:3])
print(players[:4])
print(players[2:])
print("\nHere are the first three players on my team:")
for player in players[:3]:
print(player.title())
2、复制列表
复制列表,可创建一个包含整个列表的切片,方法是省略起始索引和终止索引。
my_foods=['pizze','falafel','carrot cake']
friend_foods=my_foods[:]
my_foods.append('cannoli')
friend_foods.append('ice cream')
print('My favorite foods are:')
print(my_foods)
print("\nMy friend's favorite foods are:")
print(friend_foods)
在不使用切片的情况下复制列表得到两个列表是一样的。
五、元组
列表中的元素是可以修改的,有时候需要创建一系列不可修改的元素,python将不能修改的值称为不可变的,而不可变的列表称为元组。元组使用圆括号。遍历元组中所用的值可以使用for循环。
虽然不能修改元组的元素,但可以给储存元组的变量赋值,可重新定义整个元组。
dimensions=(200,80)
print(dimensions[0])
print(dimensions[1])
dimensions=(12,45)
for dimension in dimensions:
print(dimension)
print("Original dimensions:")
for dimension in dimensions:
print(dimension)
dimensions=(65,25)
print("\nModified dimensions:")
for dimension in dimensions:
print(dimension)
六、设置代码格式
提出python语言修改建议,需要编写python改进方案(PEP),PEP 8是最古老的PEP之一,PEP 8建议每级缩进都使用四个空格,每行不超过80个字符(可设置一个视觉标志,通常是一条竖线)。将程序的不同部分隔开可使用空格。