版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/Dan_2017/article/details/79128243
1.首先,你应该准备以下东西
(1)一个android.apk包
(2)应用签名(用android apk包名生成签名)
http://blog.csdn.net/dan_2017/article/details/79138635
(3)通过各个开放平台,填写应用信息,申请appid
QQ:腾讯开放平台http://open.qq.com/
微信:微信开放平台https://open.weixin.qq.com/
微博:微博开放平台http://open.weibo.com/
2.安装各个插件(appid填写自己申请的)
微信插件安装:
ionic cordova plugin add cordova-plugin-wechat --variable wechatappid=wx7b22a8079fcd2f45
QQ插件安装:
ionic cordova plugin add cordova-plugin-qqsdk --variable QQ_APP_ID=1105786989 --save
微博插件安装:
ionic cordova plugin add cordova-plugin-weibosdk --variable WEIBO_APP_ID=2690083339
微信插件卸载:
ionic cordova plugin rm cordova-plugin-wechat --variable wechatappid=wx7b22a8079fcd2f45
QQ插件卸载:
ionic cordova plugin rm cordova-plugin-qqsdk --variable QQ_APP_ID=1105786989
微博插件卸载:
ionic cordova plugin rm cordova-plugin-weibosdk --variable WEIBO_APP_ID=2690083339
扫描二维码关注公众号,回复:
3552307 查看本文章
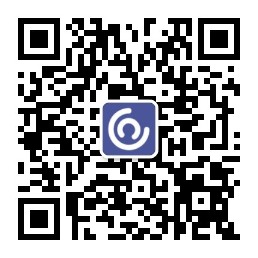
微信API:https://github.com/xu-li/cordova-plugin-wechat
QQ API:https://github.com/iVanPan/Cordova_QQ/blob/master/README_ZH.md
微博API:https://github.com/iVanPan/cordova_weibo
3.下面我用QQ举个例子:
html
<ion-content >
<button ion-button block (click)="textShare()">文字分享</button>
<button ion-button block (click)="newsShare()">链接分享</button>
<button ion-button block (click)="imgShare()">图片分享</button>
<button ion-button block (click)="flashShare()">视频分享</button>
</ion-content>
/**
* 文字分享
*/
textShare(){
let actionSheet=this.actionSheetCtrl.create({
title:'分享',
buttons:[
{
text: 'QQ好友',
handler: () => {
this.shareProvider.qqTextShare(0);
}
},
{
text: 'QQ空间',
handler: () => {
this.shareProvider.qqTextShare(1);
}
},
{
text: '微信好友',
handler: () => {
this.shareProvider.wxTextShare(0)
}
},
{
text: '朋友圈',
handler: () => {
this.shareProvider.wxTextShare(1)
}
},
{
text: '微博',
handler: () => {
this.shareProvider.wbTextShare()
}
},
{
text: '取消',
role: 'cancel',
handler: () => {
}
}
]
});
actionSheet.present();
}
/**
* 链接分享
*/
newsShare(){
let actionSheet=this.actionSheetCtrl.create({
title:'分享',
buttons:[
{
text: 'QQ好友',
handler: () => {
this.shareProvider.qqNewsShare(0);
}
},
{
text: 'QQ空间',
handler: () => {
this.shareProvider.qqNewsShare(1);
}
},
{
text: '微信好友',
handler: () => {
this.shareProvider.wxNewsShare(0);
}
},
{
text: '朋友圈',
handler: () => {
this.shareProvider.wxNewsShare(1);
}
},
{
text: '微博',
handler: () => {
this.shareProvider.wbNewsShare();
}
},
{
text: '取消',
role: 'cancel',
handler: () => {
}
}
]
});
actionSheet.present();
}
/**
* 图片分享
*/
imgShare(){
let actionSheet=this.actionSheetCtrl.create({
title:'分享',
buttons:[
{
text: 'QQ好友',
handler: () => {
this.shareProvider.qqImgShare(0);
}
},
{
text: 'QQ空间',
handler: () => {
this.shareProvider.qqImgShare(1);
}
},
{
text: '微信好友',
handler: () => {
this.shareProvider.wxImgShare(0);
}
},
{
text: '朋友圈',
handler: () => {
this.shareProvider.wxImgShare(1);
}
},
{
text: '微博',
handler: () => {
this.shareProvider.wbImgShare()
}
},
{
text: '取消',
role: 'cancel',
handler: () => {
}
}
]
});
actionSheet.present();
}
/**
* 视频分享
*/
flashShare(){
let actionSheet=this.actionSheetCtrl.create({
title:'分享',
buttons:[
{
text: 'QQ好友',
handler: () => {
this.shareProvider.qqFlashShare(0);
}
},
{
text: 'QQ空间',
handler: () => {
this.shareProvider.qqFlashShare(1);
}
},
{
text: '微信好友',
handler: () => {
this.shareProvider.wxFlashShare(0);
}
},
{
text: '朋友圈',
handler: () => {
this.shareProvider.wxFlashShare(1);
}
},
{
text: '微博',
handler: () => {
this.shareProvider.wbFlashShare()
}
},
{
text: '取消',
role: 'cancel',
handler: () => {
}
}
]
});
actionSheet.present();
import { Injectable } from '@angular/core';
import { LoadingController } from 'ionic-angular';
declare var Wechat;
declare var QQSDK;
declare var WeiboSDK;
/*
Generated class for the ShareProvider provider.
See https://angular.io/guide/dependency-injection for more info on providers
and Angular DI.
*/
@Injectable()
export class ShareProvider {
//文字
text: string = "博客";
description: string = "2017_Dan的博客";
//分享链接
link: string = "http://blog.csdn.net/Dan_2017";
//分享图片
image: string = "assets/imgs/1.jpg"
constructor() {
console.log('Hello ShareProvider Provider');
}
/**
* 微信文字分享
* @param index
*/
wxTextShare(index) {
alert(index);
Wechat.share({
text: "这是分享的一段文字",
scene: index == 0 ? Wechat.Scene.SESSION : Wechat.Scene.Timeline
}, function () {
alert("分享成功!");
}, function (reason) {
alert("失败:" + reason)
});
}
/**
* 微信链接分享
* @param index
*/
wxNewsShare(index) {
Wechat.share({
message: {
title: this.text,
thumb: this.image,
description: this.description,
mediaTagName: 'TEST-TAG-001',
messageExt: "这是第三方带的测试字段",
messageAction: "<action>dotalist</action>",
media: {
type: Wechat.Type.WEBPAGE,
webpageUrl: "https://www.csdn.net/"
}
},
scene: index == 0 ? Wechat.Scene.SESSION : Wechat.Scene.Timeline
}, function () {
alert("分享成功!");
}, function (reason) {
alert("失败:" + reason)
});
}
/**
* 微信图片分享
* @param index
*/
wxImgShare(index) {
Wechat.share({
message: {
title: '我的头像',
thumb: 'https://cordova.apache.org/static/img/cordova_bot.png',
description: '樱桃小丸子',
mediaTagName: 'TEST-TAG-001',
messageExt: "这是第三方带的测试字段",
messageAction: "<action>dotalist</action>",
media: {
type: Wechat.Type.WEBPAGE,
webpageUrl: "http://tech.qq.com/zt2012/tmtdecode/252.htm"
}
},
scene: index == 0 ? Wechat.Scene.SESSION : Wechat.Scene.Timeline
}, function () {
alert("分享成功!");
}, function (reason) {
alert("失败:" + reason)
});
}
/**
* 微信视频分享
* @param index
*/
wxFlashShare(index) {
Wechat.share({
message: {
title: "岁月神偷",
thumb: this.image,
description: '周笔畅',
mediaTagName: 'TEST-TAG-001',
messageExt: "这是第三方带的测试字段",
messageAction: "<action>dotalist</action>",
media: {
type: Wechat.Type.WEBPAGE,
webpageUrl: "http://tech.qq.com/zt2012/tmtdecode/252.htm"
}
},
scene: index == 0 ? Wechat.Scene.SESSION : Wechat.Scene.Timeline
}, function () {
alert("分享成功!");
}, function (reason) {
alert("失败:" + reason)
});
}
/**
*
* @param index QQ分享文字
*/
qqTextShare(index) {
var args: any = {};
if (index == 0) {
args.index = QQSDK.Scene.QQ;
} else {
args.index = QQSDK.Scene.QQZone;
}
args.text = "这是被分享的文字";
QQSDK.shareText(function () {
alert('分享成功')
}, function (reason) {
alert('失败:'+reason)
}, args);
}
/**
*
* @param index QQ分享链接
*/
qqNewsShare(index) {
var args: any = {};
if (index == 0) {
args.index = QQSDK.Scene.QQ;
} else {
args.index = QQSDK.Scene.QQZone;
}
args.url = this.link;
args.title = this.text;
args.image = 'https://cordova.apache.org/static/img/cordova_bot.png';
args.description = this.description;
QQSDK.shareNews(function () {
alert('分享成功')
}, function (reason) {
alert('失败:'+reason)
}, args);
}
/**
* QQ分享图片
* @param index
*/
qqImgShare(index) {
var args: any = {};
if (index == 0) {
args.index = QQSDK.Scene.QQ;
} else {
args.index = QQSDK.Scene.QQZone;
}
args.title = "cordova";
args.image = "https://cordova.apache.org/static/img/cordova_bot.png";
args.description = "cordova";
QQSDK.shareImage(function () {
alert('分享成功')
}, function (reason) {
alert('失败:'+reason)
}, args);
}
/**
*
* @param index QQ分享flash
*/
qqFlashShare(index) {
var args: any = {};
if (index == 0) {
args.index = QQSDK.Scene.QQ;
} else {
args.index = QQSDK.Scene.QQZone;
}
args.title = '岁月神偷';
args.image = 'https://cordova.apache.org/static/img/cordova_bot.png';
args.description = '周笔畅';
args.url = 'https://y.qq.com/n/yqq/song/003K45Q02b0JVS.html';
args.flashUrl = 'https://y.qq.com/portal/player.html';
QQSDK.shareAudio(function () {
alert('分享成功')
}, function (reason) {
alert('失败:'+reason)
}, args);
}
/**
* 微博文本分享
*/
wbTextShare() {
var args: any = {};
args.text = '这是我要分享的一段文字!';
WeiboSDK.shareTextToWeibo(function () {
alert('分享成功!');
}, function (reason) {
alert('分享失败' + reason);
})
}
/**
* 微博图片分享
*/
wbImgShare() {
var args: any = {};
args.image = "https://cordova.apache.org/static/img/cordova_bot.png";
WeiboSDK.shareImgToWeibo(function () {
alert('分享成功!');
}, function (reason) {
alert('分享失败' + reason);
})
}
/**
* 微博链接分享
*/
wbNewsShare() {
var args: any = {};
args.url = this.link;
args.title = this.text;
args.image = 'https://cordova.apache.org/static/img/cordova_bot.png';
args.description = this.description;
WeiboSDK.shareToWeibo(function () {
alert('分享成功!');
}, function (reason) {
alert('分享失败' + reason);
})
}
/**
* 微博视频分享
*/
wbFlashShare() {
var args: any = {};
args.title = '岁月神偷';
args.image = 'https://cordova.apache.org/static/img/cordova_bot.png';
args.description = '周笔畅';
args.url = 'https://y.qq.com/n/yqq/song/003K45Q02b0JVS.html';
args.flashUrl = 'https://y.qq.com/portal/player.html';
WeiboSDK.shareToWeibo(function () {
alert('分享成功!');
}, function (reason) {
alert('分享失败' + reason);
})
}
}
这个可以完成基本的微信微博QQ分享。
但注意如果你在申请的时候签名和包名不一致就会出现微信分享闪退,微博分享不成功等。
推荐博客:http://www.qdfuns.com/notes/15182/372db311ca31a4d868bd8fcb5de0dffa.html