HBase(2)JAVA Client
First of all, how to run jar based on maven plugin.
pom.xml
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<configuration>
<archive>
<manifest>
<mainClass>com.sillycat.easyhbase.ExecutorApp</mainClass>
</manifest>
</archive>
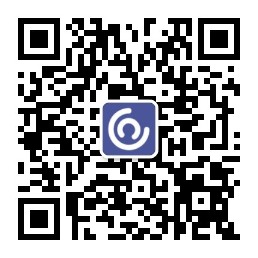
</configuration>
</plugin>
</plugins>
</build>
And the ExecutorApp is just a simplest Java Application.
package com.sillycat.easyhbase;
public class ExecutorApp {
public static void main(String[] args) {
System.out.println("Nice try.");
}
}
Fire and build the jar
>mvn clean install
Run the jar
>java -jar target/easyhbase-1.0.jar
Nice try.
Error Message
08-05 17:15:46 [WARN] org.apache.hadoop.hbase.util.DynamicClassLoader.<init>(DynamicClassLoader.java:106) - Failed to identify the fs of dir hdfs://ubuntu-master:9000/hbase/lib, ignored java.io.IOException: No FileSystem for scheme: hdfs at org.apache.hadoop.fs.FileSystem.getFileSystemClass(FileSystem.java:2385) at org.apache.hadoop.fs.FileSystem.createFileSystem(FileSystem.java:2392) at org.apache.hadoop.fs.FileSystem.access$200(FileSystem.java:89) at org.apache.hadoop.fs.FileSystem$Cache.getInternal(FileSystem.java:2431) at org.apache.hadoop.fs.FileSystem$Cache.get(FileSystem.java:2413) at org.apache.hadoop.fs.FileSystem.get(FileSystem.java:368) at org.apache.hadoop.fs.Path.getFileSystem(Path.java:296) at org.apache.hadoop.hbase.util.DynamicClassLoader.<init>(DynamicClassLoader.java:104) at org.apache.hadoop.hbase.protobuf.ProtobufUtil.<clinit>(ProtobufUtil.java:202)
Solution:
After add this jar, the problem solved.
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-hdfs</artifactId>
<version>${hadoop.version}</version>
</dependency>
Error Message
An internal error occurred during: "Updating Maven Project".
Unsupported IClasspathEntry kind=4
Solution:
Because I am using eclipse, so I use command mvn eclipse:eclipse to generate the jar dependencies.
Right click on the project [Properties] —> [Java Build Path] —>[Library]
Remove all the blue entries starting with “M2_REPO”, then I can use Maven—>Update Project again. Even I can download the source codes.
All the codes are in project easyhbase.
The dependencies are as follow:
<properties>
<hadoop.version>2.4.1</hadoop.version>
<hbase.version>0.98.4-hadoop2</hbase.version>
</properties>
<dependencies>
<dependency>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
<version>1.1.3</version>
</dependency>
<dependency>
<groupId>org.apache.hbase</groupId>
<artifactId>hbase-hadoop2-compat</artifactId>
<version>${hbase.version}</version>
</dependency>
<dependency>
<groupId>org.apache.hbase</groupId>
<artifactId>hbase-common</artifactId>
<version>${hbase.version}</version>
</dependency>
<dependency>
<groupId>org.apache.hbase</groupId>
<artifactId>hbase-client</artifactId>
<version>${hbase.version}</version>
</dependency>
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-common</artifactId>
<version>${hadoop.version}</version>
</dependency>
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-hdfs</artifactId>
<version>${hadoop.version}</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
</dependencies>
The JAVA Client Sample Codes
package com.sillycat.easyhbase;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.Cell;
import org.apache.hadoop.hbase.CellUtil;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.HColumnDescriptor;
import org.apache.hadoop.hbase.HTableDescriptor;
import org.apache.hadoop.hbase.MasterNotRunningException;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.ZooKeeperConnectionException;
import org.apache.hadoop.hbase.client.Delete;
import org.apache.hadoop.hbase.client.Get;
import org.apache.hadoop.hbase.client.HBaseAdmin;
import org.apache.hadoop.hbase.client.HTable;
import org.apache.hadoop.hbase.client.Put;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.ResultScanner;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.util.Bytes;
public class HBaseMain {
private static Configuration conf = null;
// setting
static {
conf = HBaseConfiguration.create();
}
// create table
public static void creatTable(String tableName, String[] familys) throws Exception {
HBaseAdmin admin = new HBaseAdmin(conf);
if (admin.tableExists(tableName)) {
System.out.println("table already exists!");
} else {
TableName tableNameObject = TableName.valueOf(tableName);
HTableDescriptor tableDesc = new HTableDescriptor(tableNameObject);
for (inti = 0; i < familys.length; i++) {
tableDesc.addFamily(new HColumnDescriptor(familys[i]));
}
admin.createTable(tableDesc);
System.out.println("create table " + tableName + " ok.");
}
}
// delete table
public static void deleteTable(String tableName) throws Exception {
try {
HBaseAdmin admin = new HBaseAdmin(conf);
admin.disableTable(tableName);
admin.deleteTable(tableName);
System.out.println("delete table " + tableName + " ok.");
} catch (MasterNotRunningException e) {
e.printStackTrace();
} catch (ZooKeeperConnectionException e) {
e.printStackTrace();
}
}
// insert one line
public static void addRecord(String tableName, String rowKey, String family, String qualifier, String value) throws Exception {
try {
HTable table = new HTable(conf, tableName);
Put put = new Put(Bytes.toBytes(rowKey));
put.add(Bytes.toBytes(family), Bytes.toBytes(qualifier), Bytes.toBytes(value));
table.put(put);
System.out.println("insert recored " + rowKey + " to table " + tableName + " ok.");
} catch (IOException e) {
e.printStackTrace();
}
}
// delete one line
public static void delRecord(String tableName, String rowKey) throws IOException {
HTable table = new HTable(conf, tableName);
List list = new ArrayList();
Delete del = new Delete(rowKey.getBytes());
list.add(del);
table.delete(list);
System.out.println("del recored " + rowKey + " ok.");
}
// query for one line
public static void getOneRecord(String tableName, String rowKey) throws IOException {
HTable table = new HTable(conf, tableName);
Get get = new Get(rowKey.getBytes());
Result rs = table.get(get);
for (Cell cell : rs.rawCells()) {
System.out.print(new String(CellUtil.cloneRow(cell)) + " ");
System.out.print(new String(CellUtil.cloneFamily(cell)) + ":");
System.out.print(new String(CellUtil.cloneQualifier(cell)) + " ");
System.out.print(cell.getTimestamp() + " ");
System.out.println(new String(CellUtil.cloneValue(cell))); }
}
// list all data
public static void getAllRecord(String tableName) {
try {
HTable table = new HTable(conf, tableName);
Scan s = new Scan();
ResultScanner ss = table.getScanner(s);
for (Result r : ss) {
for (Cell cell : r.rawCells()) {
System.out.print(new String(CellUtil.cloneRow(cell)) + " ");
System.out.print(new String(CellUtil.cloneFamily(cell)) + ":");
System.out.print(new String(CellUtil.cloneQualifier(cell)) + " ");
System.out.print(cell.getTimestamp() + " ");
System.out.println(new String(CellUtil.cloneValue(cell)));
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
public static void getRangeRecord(String tableName,String startRowKey,String endRowKey){
try {
HTable table = new HTable(conf, tableName);
Scan s = new Scan(startRowKey.getBytes(),endRowKey.getBytes());
ResultScanner ss = table.getScanner(s);
for (Result r : ss) {
for (Cell cell : r.rawCells()) {
System.out.print(new String(CellUtil.cloneRow(cell)) + " ");
System.out.print(new String(CellUtil.cloneFamily(cell)) + ":");
System.out.print(new String(CellUtil.cloneQualifier(cell)) + " ");
System.out.print(cell.getTimestamp() + " ");
System.out.println(new String(CellUtil.cloneValue(cell)));
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
try {
String tablename = "scores"; String[] familys = { "grade", "course" };
HBaseMain.creatTable(tablename, familys);
// add record sillycat
HBaseMain.addRecord(tablename, "sillycat-20140723", "grade", "", "5");
HBaseMain.addRecord(tablename, "sillycat-20140723", "course", "math", "97");
HBaseMain.addRecord(tablename, "sillycat-20140723", "course", "art", "87");
HBaseMain.addRecord(tablename, "sillycat-20130723", "grade", "", "5");
HBaseMain.addRecord(tablename, "sillycat-20130723", "course", "math", "97");
HBaseMain.addRecord(tablename, "sillycat-20130723", "course", "art", "87");
HBaseMain.addRecord(tablename, "sillycat-20120723", "grade", "", "5");
HBaseMain.addRecord(tablename, "sillycat-20120723", "course", "math", "97");
HBaseMain.addRecord(tablename, "sillycat-20120723", "course", "art", "87");
// add record kiko
HBaseMain.addRecord(tablename, "kiko-20140723", "grade", "", "4");
HBaseMain.addRecord(tablename, "kiko-20140723", "course", "math", "89");
System.out.println("===========get one record========");
HBaseMain.getOneRecord(tablename, "sillycat");
System.out.println("===========show all record========");
HBaseMain.getAllRecord(tablename);
System.out.println("===========del one record========");
HBaseMain.delRecord(tablename, "kiko");
HBaseMain.getAllRecord(tablename);
System.out.println("===========show all record========");
HBaseMain.getAllRecord(tablename);
System.out.print("=============show range record=======");
HBaseMain.getRangeRecord(tablename, "sillycat-20130101", "sillycat-20141231");
} catch (Exception e) {
e.printStackTrace();
}
}
}
References:
http://www.cnblogs.com/panfeng412/archive/2011/08/14/hbase-java-client-programming.html
http://lirenjuan.iteye.com/blog/1470645
http://hbase.apache.org/book/hbase_apis.html
http://blog.linezing.com/?tag=hbase
http://f.dataguru.cn/thread-226503-1-1.html
http://www.cnblogs.com/ggjucheng/p/3379459.html
http://blog.sina.com.cn/s/blog_ae33b83901016azb.html
http://blog.nosqlfan.com/html/3694.html
http://www.infoq.com/cn/news/2011/07/taobao-linhao-hbase
http://blog.csdn.net/xunianchong/article/details/8995019
client performance
http://blog.linezing.com/?p=1378
design row key
http://san-yun.iteye.com/blog/1995829