In this problem, a rooted tree is a directed graph such that, there is exactly one node (the root) for which all other nodes are descendants of this node, plus every node has exactly one parent, except for the root node which has no parents.
The given input is a directed graph that started as a rooted tree with N nodes (with distinct values 1, 2, …, N), with one additional directed edge added. The added edge has two different vertices chosen from 1 to N, and was not an edge that already existed.
The resulting graph is given as a 2D-array of edges
. Each element of edges
is a pair [u, v]
that represents a directed edge connecting nodes u
and v
, where u
is a parent of child v
.
Return an edge that can be removed so that the resulting graph is a rooted tree of N nodes. If there are multiple answers, return the answer that occurs last in the given 2D-array.
Example 1:
Input: [[1,2], [1,3], [2,3]]
Output: [2,3]
Explanation: The given directed graph will be like this:
1
/ \
v v
2-->3
Example 2:
Input: [[1,2], [2,3], [3,4], [4,1], [1,5]]
Output: [4,1]
Explanation: The given directed graph will be like this:
5 <- 1 -> 2
^ |
| v
4 <- 3
Note:
The size of the input 2D-array will be between 3 and 1000.
Every integer represented in the 2D-array will be between 1 and N, where N is the size of the input array.
解法1
从问题可以得知,要想构成一颗有向树,必须满足:
- 每个节点只有一个父节点
- 树中没有环(与无向图做法一致)
特殊样例
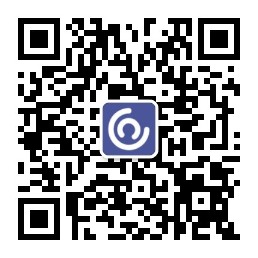
- [[1,2], [2,3], [3,1]] 每个节点有一个父节点但是有环
- [[1,2], [2,3], [1,3]] 节点3有两个父节点,故删除边从[2,3]和[1,3]中选择。
- [[4,2], [2,1], [1,4], [3, 1]] 节点1有两个父节点。删除[2,1]或者[3,1],默认情况下删除[3,1],但由于[2,1]可以构成环,故需要删除[2,1]
class Solution {
int fa[1002];
public:
vector<int> findRedundantDirectedConnection(vector<vector<int>>& edges) {
vector<int> e1, e2; // 如果有某个节点有两个父节点,则将这两条边存起来,删除的话只会在这里面删除。
memset(fa, -1, sizeof(fa)); // 只记录节点的父节点,不做路径压缩
for(auto e: edges) {
int u = e[0], v = e[1];
if(fa[v] != -1) {
e1 = {fa[v], v};
e2 = {u, v};
break;
}
else {
fa[v] = u; //v的父节点记录为u
}
}
memset(fa, -1, sizeof(fa));
if(e1.empty()) { // 找不到某个结点有两个父节点,必然存在环。
for(auto e: edges) {
int u=e[0], v= e[1];
int fu=find(u), fv=find(v);
if(fu!=fv) {
fa[fu]=fv;
}
else {
return {u, v}; // 跟有向图的判断类似
}
}
}
else {
for(auto e: edges) {
int u=e[0], v=e[1];
if(u==e2[0]&&v==e2[1]) continue;
int fu=find(u), fv=find(v);
if(fu!=fv) {
fa[fu]=fv;
}
else
return e1;
}
}
return e2;
}
int find(int x) {
return fa[x]==-1?x:fa[x]=find(fa[x]);
}
};