#1.主页面
package com.bw.ymy.imageloader;
import android.content.Intent;
import android.database.Cursor;
import android.graphics.Bitmap;
import android.net.Uri;
import android.provider.MediaStore;
import android.support.annotation.Nullable;
import android.support.v4.content.CursorLoader;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private EditText width;
private EditText height;
private Button getImage;
private Button show;
private Uri uri;
private ImageView image;
private String w;
private String h;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
width = findViewById(R.id.width);
height = findViewById(R.id.height);
getImage = findViewById(R.id.get_image);
show = findViewById(R.id.show);
image = findViewById(R.id.image);
//点击选择图片
getImage.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//打开系统相册
Intent intent = new Intent(Intent.ACTION_PICK);
//设置类型
intent.setType("image/*");
//执行
startActivityForResult(intent, 100);
}
});
//点击生成
show.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//获取输入框的值
w = width.getText().toString();
h = height.getText().toString();
if (w.equals("") || h.equals("")) {
Toast.makeText(MainActivity.this, "请输入宽,高", Toast.LENGTH_SHORT).show();
} else {
if (uri != null) {
String filePath = getUri(uri);
NetUtils.getIntance().scaleBitmap(filePath, Integer.parseInt(w),
Integer.parseInt(h), new NetUtils.CallBack() {
@Override
public void onSuccess(Bitmap bitmap) {
image.setImageBitmap(bitmap);
}
}
);
} else {
Toast.makeText(MainActivity.this, "请选择图片", Toast.LENGTH_SHORT).show();
}
}
}
});
}
//传入图片路径
private String getUri(Uri contentUri) {
String[] pr = {MediaStore.Images.Media.DATA};
CursorLoader loader = new CursorLoader(this, contentUri, pr, null, null, null);
Cursor cursor = loader.loadInBackground();
int index = cursor.getColumnIndexOrThrow(MediaStore.Images.Media.DATA);
cursor.moveToFirst();
return cursor.getString(index);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == 100 && resultCode == RESULT_OK) {
//图片的uri
uri = data.getData();
return;
}
}
}
#2.工具类
package com.bw.ymy.imageloader;
import android.annotation.SuppressLint;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.AsyncTask;
public class NetUtils {
private static NetUtils intance;
public NetUtils() {
}
public static NetUtils getIntance() {
if(intance == null){
intance = new NetUtils();
}
return intance;
}
//图片二次采样
public Bitmap scaleBitmap(String filePath,int width,int height){
//第一次直解析bitmap的宽高信息
BitmapFactory.Options op = new BitmapFactory.Options();
//指定只解析bitmap的边界信息,不加载bitmap到内存中
op.inJustDecodeBounds = true;
BitmapFactory.decodeFile(filePath,op);
//通过图片的真实宽高信息和需要的宽高产生比例
op.inSampleSize = Math.max(op.outHeight/height,op.outWidth/width);
//第二次真正加载图片
op.inJustDecodeBounds = false;
Bitmap bitmap = BitmapFactory.decodeFile(filePath,op);
return bitmap;
}
//定义接口
public interface CallBack{
void onSuccess(Bitmap bitmap);
}
@SuppressLint("StaticFieldLeak")
public void scaleBitmap(String filePath, final int width, final int height, final CallBack callBack){
new AsyncTask<String,Void,Bitmap>(){
@Override
protected Bitmap doInBackground(String... strings) {
return scaleBitmap(strings[0],width,height);
}
@Override
protected void onPostExecute(Bitmap bitmap) {
callBack.onSuccess(bitmap);
}
}.execute(filePath);
}
}
//#3.布局
<?xml version="1.0" encoding="utf-8"?><android.support.constraint.ConstraintLayout xmlns:android=“http://schemas.android.com/apk/res/android”
xmlns:app=“http://schemas.android.com/apk/res-auto”
xmlns:tools=“http://schemas.android.com/tools”
android:layout_width=“match_parent”
android:layout_height=“match_parent”
tools:context=".MainActivity">
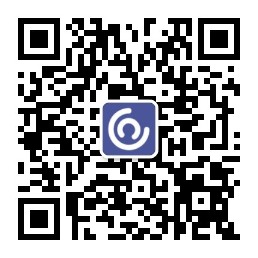
<EditText
android:id="@+id/width"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintTop_toBottomOf="@id/get_image"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toLeftOf="@id/height"
android:hint="输入宽度"/>
<EditText
android:id="@+id/height"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintTop_toBottomOf="@id/get_image"
app:layout_constraintLeft_toRightOf="@id/width"
app:layout_constraintRight_toRightOf="parent"
android:hint="输入高度"/>
<Button
android:id="@+id/show"
android:layout_width="0dp"
android:layout_height="wrap_content"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toBottomOf="@id/height"
android:text="生成"/>
<ImageView
android:id="@+id/image"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toBottomOf="@id/show"
app:layout_constraintBottom_toBottomOf="parent"/>
</android.support.constraint.ConstraintLayout>
//加权限