**最大公约数和最小公倍数
Write a program which computes the greatest common divisor (GCD) and the least common multiple (LCM) of given a and b.
Input
Input consists of several data sets. Each data set contains a and b separated by a single space in a line. The input terminates with EOF.
Constraints
0 < a, b ≤ 2,000,000,000
LCM(a, b) ≤ 2,000,000,000
The number of data sets ≤ 50
Output
For each data set, print GCD and LCM separated by a single space in a line.
Sample Input
8 6
50000000 30000000
Output for the Sample Input
2 24
10000000 150000000**
#include<stdio.h>
#include<math.h>
int main()
{
int a, b, x, y, t;
int GCD, LCM;
while (scanf("%d%d", &a, &b) != EOF)
{
x = a;
y = b;
if (a < b)
{
t = a;
a = b;
b = t;
}
while (b != 0)
{
t = b;
b = a % b;
a = t;
}
GCD = a;
LCM = x / a * y;
printf("%d %d\n", GCD, LCM);
}
return 0;
}
**素数
Write a program which reads an integer n and prints the number of prime numbers which are less than or equal to n. A prime number is a natural number which has exactly two distinct natural number divisors: 1 and itself. For example, the first four prime numbers are: 2, 3, 5 and 7.
Input
Input consists of several datasets. Each dataset has an integer n (1 ≤ n ≤ 999,999) in a line.
The number of datasets is less than or equal to 30.
Output
For each dataset, prints the number of prime numbers.
Sample Input
10
3
11
Output for the Sample Input
4
2
5
**
#include<stdio.h>
#include<string.h>
#include<iostream>
const int maxn = 1000005;
const int _maxn = 78499 + 5;
int prime[_maxn];
bool vis[maxn];
int main()
{
int n;
while(scanf("%d",&n)!=EOF)
{
int count=0;
memset(vis,0,sizeof(vis));
for(int i=2;i<=n;i++)
{
if (!vis[i]) *vis[i]!=0时执行,也就是他是素数的时候执行*
prime[count++] = i;
for (int j = i+i; j<=n; j=j+i)
{
vis[j] = 1;*将不是素数的标记为1*
}
}
printf("%d\n",count);
}
return 0;
}
**超级楼梯
有一楼梯共M级,刚开始时你在第一级,若每次只能跨上一级或二级,要走上第M级,共有多少种走法?
Input
输入数据首先包含一个整数N,表示测试实例的个数,然后是N行数据,每行包含一个整数M(1<=M<=40),表示楼梯的级数。
Output
对于每个测试实例,请输出不同走法的数量
Sample Input
2
2
3
Sample Output
1
2**
#include<stdio.h>
int main()
{
int n, m;
int f[41];
scanf("%d",&n);
f[1] = 0;
f[2] = 1;
f[3] = 2;
for(m=4;m<41;m++)
{
f[m] = f[m - 1] + f[m - 2]; *走一步或走两步*
}
while(n--)
{
scanf("%d",&m);
printf("%d\n",f[m]);
}
return 0;
}
**计算球体积
根据输入的半径值,计算球的体积。
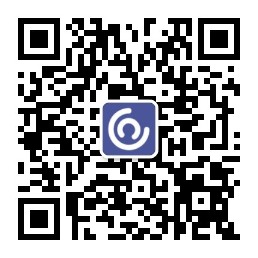
Input
输入数据有多组,每组占一行,每行包括一个实数,表示球的半径。
Output
输出对应的球的体积,对于每组输入数据,输出一行,计算结果保留三位小数。
Sample Input
1
1.5
Sample Output
4.189
14.137
Hint
#define PI 3.1415927
**
#include<stdio.h>
#include<math.h>
#define PI 3.1415927
int main()
{
double r, v;
while (scanf("%lf", &r) != EOF)
{
v = 4 * PI*r*r*r / 3;
printf("%.3lf\n", v);
}
return 0;
}
**求实数的绝对值。
Input
输入数据有多组,每组占一行,每行包含一个实数。
Output
对于每组输入数据,输出它的绝对值,要求每组数据输出一行,结果保留两位小数。
Sample Input
123
-234.00
Sample Output
123.00
234.00**
#include<stdio.h>
int main()
{
double a;
while (scanf("%lf",&a)!=EOF)
{
if (a>=0)
{
printf("%.2lf\n", a);
}
else
{
printf("%.2lf\n", -a);
}
}
return 0;
}