6-1 输出月份英文名
1.设计思路
(1)主要描述题目算法
第一步:先定义一个指针数组。
第二步:根据for循环判断月份并返还月份字数。
2.实验代码
int getindex( char *s )
{
int i=0,t=-1;
char *p[7]={"Sunday","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday"};
for(i=0;i<7;i++)
{
if(*p[i]==*s&&strlen(*p[i])==strlen(*s))
{
return i;
}
}
return t;
}
3.本题调试过程碰到问题及解决办法
无问题.
6-2 查找星期
1.设计思路
(1)主要描述题目算法
第一步:定义一个字符指针数组。
第二步:用循环和条件语句判断这个数是否等于0。等于就返回i。
第三步:否则返回t。
(2)流程图
2.实验代码
int getindex( char *s )
{
int i=0,t=-1;
char *p[7]={"Sunday","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday"};
for(i=0;i<7;i++)
{
if(strcmp(p[i],s)==0)
{
return i;
}
}
return t;
}
3.本题调试过程碰到问题及解决办法
无问题.
6-3 计算最长的字符串长度
1.设计思路
(1)主要描述题目算法
第一步:定义初值。
第二步:根据循环和判断来判断大小。
第三步:返回最长字符串的字符返回字数。
2.实验代码
int max_len( char *s[], int n )
{
int i,t=0;
for(i=0;i<n;i++)
{
if(strlen(s[i])>strlen(s[t]))
{
t=i;
}
}
return strlen(s[t]);
}```
###3.本题调试过程碰到问题及解决办法
无。
###**6-4 指定位置输出字符串**
###1.设计思路
(1)主要描述题目算法
第一步:定义初值t,g,mark,flag。
第二步:根据判断结果不同标记成不同的数值。
第三步:根据不同条件了来返回其中的字符串。
###2.实验代码
char match( char s, char ch1, char ch2 )
{
int i=0,j=0,t=1,g=1,mark,flag;
for(i=0;;i++)
{ if((s+i)=='\0')
{break;
}
if((s+i)==ch1&&t!=0)
{t=0;
mark=i;}
if((s+i)==ch2)
{g=0;
flag=i;
}
}
if(t==1)
{
printf("\n");
s='\0';
return s;
}
if(t!=1&&g==0)
{
for(j=mark;;j++)
{
if((s+j)=='\0')
{break;
}
printf("%c",(s+j));
if(*(s+j)==ch2)
{ printf("\n");
return (s+mark);
}
}
}
if(t!=1&&g==1)
{
printf("%s\n",(s+mark));
return (s+mark);
}
}
###3.本题调试过程碰到问题及解决办法
无。
###**6-1 奇数值结点链表**
###1.设计思路
(1)主要描述题目算法
第一步:创建2个新的链表。
第二步:根据所给链表的奇偶性不同将其给不同的链表。
第三步:返回2个链表的头结点。
###2.实验代码
struct ListNode readlist()
{
int data;
struct ListNode head=NULL;
struct ListNode p;
while(scanf("%d",&data)&&data!=-1)
{
struct ListNode q=(struct ListNode)malloc(sizeof(struct ListNode));
if(q!=NULL)
{
q->data=data;
q->next=NULL;
}
else exit(1);
if(head!=NULL)
{
p->next=q;
}
else head=q;
p=q;
}
return head;
}
struct ListNode getodd( struct ListNode **L )
{
struct ListNode head0=NULL,head1=NULL,p0,p1;
while((L)!=NULL)
{
int data=(L)->data;
struct ListNode q=(struct ListNode)malloc(sizeof(struct ListNode));
if(data%2)
{
if(q!=NULL)
{
q->data=data;
q->next=NULL;
}
else exit(1);
if(head1!=NULL)
{
p1->next=q;
}
else head1=q;
p1=q;
}
else
{
if(q!=NULL)
{
q->data=data;
q->next=NULL;
}
else exit(1);
if(head0!=NULL)
{
p0->next=q;
}
else head0=q;
p0=q;
}
L=(L)->next;
}
*L=head0;
return head1;
}
###3.本题调试过程碰到问题及解决办法
无
###**6-2 学生成绩链表处理**
###1.设计思路
(1)主要描述题目算法
第一步:建立一个链表利用循环来进行赋值。
第二步:输入条件值历链表,对每个低于条件值的节点进行删除。
第三步:返回删除操作后的链表头结点。
(2)流程图
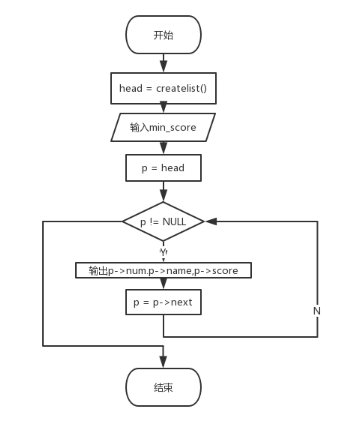
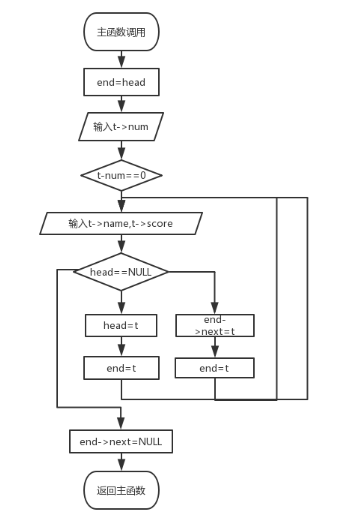
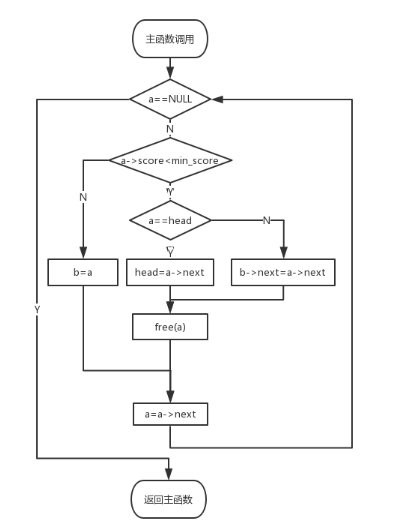
###2.实验代码
struct stud_node createlist()
{
struct stud_node head, tail, q;
head = tail = NULL;
int num;
scanf ("%d", &num);
while (num != 0)
{
q = (struct stud_node )malloc (sizeof (struct stud_node));
scanf ("%s %d", q->name, &q->score);
q->num = num;
q->next = NULL;
if (head == NULL)
head = q;
else
tail->next = q;
tail = q;
scanf ("%d", &num);
}
return head;
}
struct stud_node deletelist( struct stud_node head, int min_score )
{
struct stud_node ptr1, *ptr2;
while (head != NULL && head->score < min_score)
{
ptr2 = head;
head = head->next;
free(ptr2);
}
if (head == NULL)
return NULL;
ptr1 = head;
ptr2 = head->next;
while (ptr2 != NULL)
{
if (ptr2->score < min_score) {
ptr1->next = ptr2->next;
free(ptr2);
}
else
ptr1 = ptr2;
ptr2 = ptr1->next;
}
return head;
}
###3.本题调试过程碰到问题及解决办法
(无)
###**6-3 链表拼接**
###1.设计思路
(1)主要描述题目算法
第一步:将两个数组的数值赋值在一个数组里。
第二步:将数组进行排序从而得到有序数组。
第三步:把数组各元素赋值回一个新链表,返回链表头结点。
###2.实验代码
struct ListNode mergelists(struct ListNode list1, struct ListNode list2)
{
int num = 0;
int temp[100];
struct ListNode p = list1;
while(p != NULL)
{
temp[num] = p->data;
num++;
p = p->next;
}
p = list2;
while(p != NULL)
{
temp[num] = p->data;
num++;
p = p->next;
}
int i,j;
for(i = 0; i < num; i++)
for(j = i + 1; j < num; j++)
{
if(temp[i] > temp[j])
{
int t;
t = temp[i];
temp[i] = temp[j];
temp[j] = t;
}
}
struct ListNode newlist = NULL;
struct ListNode endlist = NULL;
struct ListNode q;
for(i = 0; i < num; i++)
{
q = (struct ListNode )malloc(sizeof(struct ListNode));
q->data = temp[i];
if(newlist == NULL)
{
newlist = q;
newlist->next = NULL;
}
if(endlist != NULL)
{
endlist->next = q;
}
endlist = q;
endlist->next = NULL;
}
return newlist;
}```
3.本题调试过程碰到问题及解决办法
无
1总结两周里所学的知识点有哪些学会了?哪些还没有学会?
这两周我学会了指针数组,指向指针的指针 ,用指针数组处理多个字符串,命令行参数,指针作为函数的返回值,链表 ,链表的概念。
链表的常用操作###2、将PTA作业的源代码使用git提交到托管平台上,要求给出上传成功截图和你的git地址。请注意git地址应是类似
https://git.coding.net/yangzhiqun/cfsafa.git