题目:
在给定单词列表 wordlist
的情况下,我们希望实现一个拼写检查器,将查询单词转换为正确的单词。
对于给定的查询单词 query
,拼写检查器将会处理两类拼写错误:
- 大小写:如果查询匹配单词列表中的某个单词(不区分大小写),则返回的正确单词与单词列表中的大小写相同。
- 例如:
wordlist = ["yellow"]
,query = "YellOw"
:correct = "yellow"
- 例如:
wordlist = ["Yellow"]
,query = "yellow"
:correct = "Yellow"
- 例如:
wordlist = ["yellow"]
,query = "yellow"
:correct = "yellow"
- 例如:
- 元音错误:如果在将查询单词中的元音(‘a’、‘e’、‘i’、‘o’、‘u’)分别替换为任何元音后,能与单词列表中的单词匹配(不区分大小写),则返回的正确单词与单词列表中的匹配项大小写相同。
- 例如:
wordlist = ["YellOw"]
,query = "yollow"
:correct = "YellOw"
- 例如:
wordlist = ["YellOw"]
,query = "yeellow"
:correct = ""
(无匹配项) - 例如:
wordlist = ["YellOw"]
,query = "yllw"
:correct = ""
(无匹配项)
- 例如:
此外,拼写检查器还按照以下优先级规则操作:
- 当查询完全匹配单词列表中的某个单词(区分大小写)时,应返回相同的单词。
- 当查询匹配到大小写问题的单词时,您应该返回单词列表中的第一个这样的匹配项。
- 当查询匹配到元音错误的单词时,您应该返回单词列表中的第一个这样的匹配项。
- 如果该查询在单词列表中没有匹配项,则应返回空字符串。
给出一些查询 queries
,返回一个单词答案列表 answer
,其中 answer[i]
是由查询 query = queries[i]
得到的正确单词。
思路:5000个原字符串,5000个带查询字符串,分为三种查询模式。
1.都是原串查询
2,把它们都变成大写的字符串之后查询
3.把它们之中的元音字母都变成A之后查询
思路很简单,根据这三种不同的字符串建三颗字典树就可以愉快的开始查询了,这是我的SB做法,写了估计有一个多小时。
实际上,你不需要自己手动建树,用三颗map就可以很愉快的哈希了,想哭QAQ,窝手撸了一个小时,人家一个map轻松过关。
扫描二维码关注公众号,回复:
4710047 查看本文章
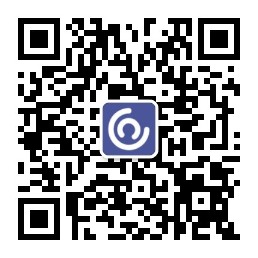
白写了三颗字典树,好气啊!!!
我的代码:
int ch[30000][3][60];
int tot1,tot2,tot3;
int root;
class Solution {
public:
void init(){
memset(ch[0],-1,sizeof(ch[0]));
tot1 = tot2 = tot3 = 0;
root = 0;
}
void insert(string s,int num){
int len = s.length();
int u = root;
int tot = 0;
if(num==0)tot = tot1;
if(num==1)tot = tot2;
if(num==2)tot = tot3;
for(int i=0;i<len;i++){
int c = s[i]-'A';
if(-1==ch[u][num][c]){
tot++;
memset(ch[tot][num],-1,sizeof(ch[tot][num]));
ch[u][num][c] = tot;
}
u = ch[u][num][c];
}
if(num==0)tot1 = tot;
if(num==1)tot2 = tot;
if(num==2)tot3 = tot;
}
bool query_s(string s,int num){
int len = s.length();
int u = root;
for(int i=0;i<len;i++){
int c = s[i]-'A';
if(-1==ch[u][num][c])return false;
u = ch[u][num][c];
}
return true;
}
string to_upp(string s){ //将字符串转化为大写
int len = s.length();
for(int i=0;i<len;i++){
if(s[i]>='a'&&s[i]<='z')s[i]-= 32;
}
return s;
}
string change_word(string a){//改造为第三种字符串
int len = a.length();
string s = to_upp(a);
for(int i=0;i<len;i++){
if(s[i]=='A'||s[i]=='E'||s[i]=='I'||s[i]=='O'||s[i]=='U')s[i] = 'A';
}
return s;
}
vector<string> spellchecker(vector<string>& wordlist, vector<string>& queries) {
map<string,int>ma1,ma2,ma3;
int word_len = wordlist.size(),qu_len = queries.size();
init();
for(int i=0;i<word_len;i++){
insert(wordlist[i],0);
if(ma1.find(wordlist[i])==ma1.end())ma1[wordlist[i]] = i;
string s = to_upp(wordlist[i]);
insert(s,1);
if(ma2.find(s)==ma2.end())ma2[s] = i;
string ss = change_word(wordlist[i]);
insert(ss,2);
if(ma3.find(ss)==ma3.end())ma3[ss] = i;
}
vector<string>ans;
for(int i=0;i<qu_len;i++){//分别插入与查询
string s = to_upp(queries[i]);
string ss = change_word(queries[i]);
// cout<<s<<" "<<ss<<endl;
// cout<<query_s(queries[i],0)<<" "<<query_s(s,1)<<endl;
if(query_s(queries[i],0)){
ans.push_back(wordlist[ma1[queries[i]]]);
}
else if(query_s(s,1)){
ans.push_back(wordlist[ma2[s]]);
}
else if(query_s(ss,2)){
ans.push_back(wordlist[ma3[ss]]);
}
else {
ans.push_back("");
}
}
return ans;
}
};
用map的做法:
class Solution {
public:
string to_upp(string s){
int len = s.length();
for(int i=0;i<len;i++){
if(s[i]>='a'&&s[i]<='z')s[i]-= 32;
}
return s;
}
string change_word(string a){
int len = a.length();
string s = to_upp(a);
for(int i=0;i<len;i++){
if(s[i]=='A'||s[i]=='E'||s[i]=='I'||s[i]=='O'||s[i]=='U')s[i] = 'A';
}
return s;
}
vector<string> spellchecker(vector<string>& wordlist, vector<string>& queries) {
map<string,int>ma1,ma2,ma3;
int word_len = wordlist.size(),qu_len = queries.size();
for(int i=0;i<word_len;i++){
if(ma1.find(wordlist[i])==ma1.end())ma1[wordlist[i]] = i;
string s = to_upp(wordlist[i]);
if(ma2.find(s)==ma2.end())ma2[s] = i;
string ss = change_word(wordlist[i]);
if(ma3.find(ss)==ma3.end())ma3[ss] = i;
}
vector<string>ans;
for(int i=0;i<qu_len;i++){
string s = to_upp(queries[i]);
string ss = change_word(queries[i]);
// cout<<s<<" "<<ss<<endl;
// cout<<query_s(queries[i],0)<<" "<<query_s(s,1)<<endl;
if(ma1.find(queries[i])!=ma1.end()){
ans.push_back(wordlist[ma1[queries[i]]]);
}
else if(ma2.find(s)!=ma2.end()){
ans.push_back(wordlist[ma2[s]]);
}
else if(ma3.find(ss)!=ma3.end()){
ans.push_back(wordlist[ma3[ss]]);
}
else {
ans.push_back("");
}
}
return ans;
}
};
就是把查询的方式变成了直接用map find,哎,难受。