版权声明:转载请注明出处 https://blog.csdn.net/qq_42292831/article/details/85526932
Given a string, find the length of the longest substring without repeating characters.
Example 1:
Input: "abcabcbb" Output: 3 Explanation: The answer is "abc", with the length of 3.
Example 2:
Input: "bbbbb" Output: 1 Explanation: The answer is "b", with the length of 1.
Example 3:
Input: "pwwkew" Output: 3 Explanation: The answer is "wke", with the length of 3. Note that the answer must be a substring, "pwke"is a subsequence and not a substring.
************************************************************************************************************************************
这里我实在很无奈,看着排名靠前的那些代码,思路一团糟,只能找点可以理解的给消化掉:
扫描二维码关注公众号,回复:
4735004 查看本文章
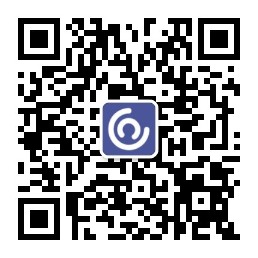
解决方案与思路分析*3:
单独开辟内存空间来存储子串*temp,而*(length+i)表示最外层循环每次对应的子串长度;
首先控制最外层循环所执行的次数为count,内部子序列循环次数依旧是count,起始值为i+1;
在内层循环过程中需要对子串进行判断(是否和index大于i的数据相等),不相等*(length+i)++,否则跳出两个循环。
int lengthOfLongestSubstring(char* s) {
int count =0;
while(s[count]!='\0')
{
count++;
}
char *temp = (char *)malloc(sizeof(char)*count);
int *length = (int *)malloc(sizeof(int)*count);
for(int i=0;i<count;i++)
{
length[i] = 1;
temp[0] = s[i];
for(int j=i+1;j<count;j++)
{
bool flag =false;
for(int k=j-1;k>=i;k--)
{
if(s[j]==s[k])
{
break;
}
else if(s[j]!=s[k]&&k==i)
{
temp[j] = s[j];
length[i]++;
flag = true;
}
}
if(!flag)
{
break;
}
}
}
int max_length =length[0];
for(int i=0;i<count-1;i++)
{
if(max_length<=length[i+1])
{
max_length = length[i+1];
}
}
return max_length;
}
分析后发现,没有必要额外存储子串,删除后,时间减少为184ms:
*也可以使用strlen()来直接求字符串数组长度,时间上没有太大差别
分析了部分其他人的源码,发现还可以使用ASCII码的思想来做:
基本思想:
将每个字符的ASCII码映作为另一个数组的下标,对该下标的值进行替换;
这样做可以直接在第二层循环中开始进行判断,而不需要像上面一样再新增加一个循环;
(实际上是增加了空间的消耗来减少了时间的消耗)
int lengthOfLongestSubstring(char* s)
{
int count = strlen(s);
int *length = (int*)malloc(sizeof(int)*count);
int ch;
int ascii_in_s[256];
for(int i=0;i<256;i++) //初始化ASCII码数组
{
ascii_in_s[i] = -1;
}
for(int i=0;i<count;i++) //初始化长度数组
{
length[i]=1;
}
for(int i=0;i<count;i++)
{
ch = s[i];
ascii_in_s[ch] = i;
for(int j =i+1;j<count;j++)
{
ch = s[j];
if(ascii_in_s[ch]==-1)
{
ascii_in_s[ch] = j;
length[i]++;
}
else
{
break;
}
}
for (int k = 0; k < 256; k++) //最外层循环每进行一次,需要对数组进行一次初始化
{
ascii_in_s[k] = -1;
}
}
int max_length =length[0];
for(int i=0;i<count-1;i++)
{
if(max_length<=length[i+1])
{
max_length = length[i+1];
}
}
return max_length;
}