指针作为函数参量
指针作为函数参量,以地址的方式传递数据,可以用来返回函数处理结果;实参是数组名时形参可以是指针。
题目:读入三个浮点数,将整数部分和小数部分分别输出
#include <iostream>
using namespace std;
void splitfloat(float x, int *intpart, float *fracpart)
{ //形参intpart、 fracpart是指针
*intpart=int(x); // 取x的整数部分
*fracpart=x-*intpart; //取x的小数部分
}
int main()
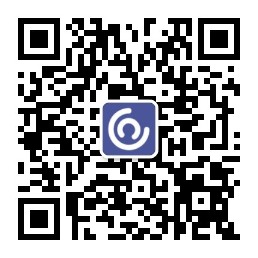
{
int i, n;
float x, f;
cout<<"Enter three(3) floating point numbers"
<< endl;
for (i = 0; i < 3; i++)
{
cin >> x;
splitfloat(x,&n,&f); //变量地址做实参
cout<<"Integer Part is "<< n
<<" Fraction Part is "<<f<<endl;
}
}
运行结果:
Enter three(3) floating point numbers
4.7
Integer Part is 4 Fraction Part is 0.7
8.913
Integer Part is 8 Fraction Part is 0.913
-4.7518
Integer Part is -4 Fraction Part is -0.7518
输出数组元素的内容和地址
#include <iostream>
#include <iomanip>
using namespace std;
void Array_Ptr(long *P, int n)
{ int i;
cout<<"In func, address of array is "
<<unsigned long(P)<<endl;
cout<<"Accessing array using pointers"<< endl;
for (i = 0; i < n; i++)
{ cout<<" Address for index "<<i<<" is "
<<unsigned long(P+i);
cout<<" Value is "<<*(P+i)<<endl;
}
}
int main()
{ long list[5]={50, 60, 70, 80, 90};
cout<<"In main, address of array is "
<< unsigned long(list)<<endl;
cout<<endl;
Array_Ptr(list,5);
}
某一次运行结果:
In main, address of array is 6684132
In func, address of array is 6684132
Accessing array using pointers
Address for index 0 is 6684132 Value is 50
Address for index 1 is 6684136 Value is 60
Address for index 2 is 6684140 Value is 70
Address for index 3 is 6684144 Value is 80
Address for index 4 is 6684148 Value is 90
用指向常量的指针做形参
#include<iostream>
using namespace std;
const int N=6;
void print(const int *p,int n);
int main()
{ int array[N];
for(int i=0;i<N;i++)
cin>>array[i];
print(array,N);
}
void print(const int *p, int n)
{
cout<<"{"<<*p;
for(int i=1;i<n;i++)
cout<<"."<<*(p+i);
cout<<"}"<<endl;
}
当函数的返回值是地址时,该函数就是指针型函数。声明:存储类型 数据类型 *函数名();
指向函数的指针 存储类型 数据类型 (*函数名)();数据指针指向数据存储区,而函数指针指向的程序代码的存储区(函数名是函数代码的恰是地址)。
#include <iostream>
using namespace std;
void print_stuff(float data_to_ignore);
void print_message(float list_this_data);
void print_float(float data_to_print);
void (*function_pointer)(float); //void类型的函数指针
int main() //主函数
{
float pi = (float)3.14159;
float two_pi = (float)2.0 * pi;
print_stuff(pi);
function_pointer = print_stuff; //函数指针指向print_stuff
function_pointer(pi); //函数指针调用,相当于调用print_stuff
function_pointer = print_message; //函数指针指向print_message
function_pointer(two_pi); //函数指针调用
function_pointer(13.0); //函数指针调用
function_pointer = print_float; //函数指针指向print_float
function_pointer(pi); //函数指针调用
print_float(pi);
}
void print_stuff(float data_to_ignore)
{ cout<<"This is the print stuff function.\n"; }
void print_message(float list_this_data)
{ cout<<"The data to be listed is "<<list_this_data<<endl; }
void print_float(float data_to_print)
{ cout<<"The data to be printed is "<<data_to_print<<endl; }
//运行结果:
This is the print stuff function.
This is the print stuff function.
The data to be listed is 6.283180
The data to be listed is 13.000000
The data to be printed is 3.141590
The data to be printed is 3.141590