实现一个二叉搜索树迭代器。你将使用二叉搜索树的根节点初始化迭代器。
调用 next() 将返回二叉搜索树中的下一个最小的数。
示例:
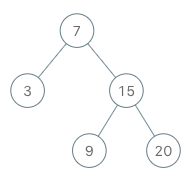
BSTIterator iterator = new BSTIterator(root);
iterator.next(); // 返回 3
iterator.next(); // 返回 7
iterator.hasNext(); // 返回 true
iterator.next(); // 返回 9
iterator.hasNext(); // 返回 true
iterator.next(); // 返回 15
iterator.hasNext(); // 返回 true
iterator.next(); // 返回 20
iterator.hasNext(); // 返回 false
提示:
next() 和 hasNext() 操作的时间复杂度是 O(1),并使用 O(h) 内存,其中 h 是树的高度。
你可以假设 next() 调用总是有效的,也就是说,当调用 next() 时,BST 中至少存在一个下一个最小的数。
*/
/**
* Your BSTIterator object will be instantiated and called as such: BSTIterator obj = new
* BSTIterator(root); int param_1 = obj.next(); boolean param_2 = obj.hasNext();
*/
二叉搜索树中序遍历: 元素从小到大排列.
如:
中序遍历结果: 3,7,9,15,20
思路1: 队列式. 中序遍历二叉树,将所有节点添加到队列中.
空间复杂度=O(n) > O(h)
next() 时间复杂度O(1)
思路2: 栈式. 利用栈中序遍历,即通过调用next()来推动遍历.在没有调用next()方法时,接下来的元素不一定被访问到.
空间复杂度=O(h)
next() 时间复杂度O(1) **(均摊)**
1 //队列式
2 class BSTIterator_queue {
3
4 private Queue<TreeNode> queue = new ArrayDeque<>();
5
6
7 public BSTIterator_queue(TreeNode root) {
8 addElem(root);
9 }
10
11 private void addElem(TreeNode root) {
12 if (root != null) {
13 addElem(root.left);
14 queue.add(root);
15 addElem(root.right);
16 }
17 }
18
19 /*
20 * @return the next smallest number
21 */
22 public int next() {
23 return queue.remove().val;
24 }
25
26 /*
27 * @return whether we have a next smallest number
28 */
29 public boolean hasNext() {
30 return queue.size() != 0;
31 }
32
33 }
34
35
36
37 =======================================
38
39
40
41 //栈式
42 class BSTIterator_stack {
43
44 private Stack<TreeNode> stack = new Stack<>();
45 private TreeNode currNode;
46
47 public BSTIterator_stack(TreeNode root) {
48 currNode = root;
49 }
50
51 /*
52 * @return the next smallest number
53 */
54 public int next() {
55 while (currNode != null) {
56 stack.push(currNode);
57 currNode = currNode.left;
58 }
59 currNode = stack.pop();
60 TreeNode tempNode = currNode;
61 currNode = currNode.right;
62 return tempNode.val;
63 }
64
65 /*
66 * @return whether we have a next smallest number
67 */
68 public boolean hasNext() {
69 return stack.isEmpty() && currNode == null;
70 }
71 }