自动拆装箱
有时我们需要将int这样的基本类型转换为对象。所有的基本类型都有一个与之对应的类。例如,Integer对应基本类型int。通常这种类称为包装器(wrapper)。这些对象包装器类拥有很鲜明的名字:Integer、Long、Float、Double、Short、Byte、Character、Void和Boolean。对象包装器类是不可变的,即一旦构造了包装器,就不允许更改包装在其中的值。同时,对象包装器类是final的,因此不能定义它们的子类。
提示:实际上,Java中还存在另外一种基本类型void
,它也有对应的包装类java.lang.Void
,不过我们无法直接对它们进行操作。
设想定义一个整型链表,而尖括号<>中类型就不允许是基本数据类型的,即不允许写成ArrayList<int>。这里就用到了Integer对象包装器类。我们可以声明一个Integer对象的链表:
ArrayList<Integer> list = new ArrayList<Integer>();
提示:由于每个值分别包装在对象中,所以ArrayList<Integer>的效率远远低于int[]数组。
概念:
1)自动装箱:Java自动将基本数据类型值转换成对应的对象,比如将int的变量转换成Integer对象,这个过程叫做装箱。
例如: 调用list.add(3);将自动地变换成 list.add(Integer.valueOf(3)); 这种变换被称为自动装箱。
2)自动拆箱就是:反之将Integer对象转换成int类型值,这个过程叫做拆箱。因为这里的装箱和拆箱是自动进行的非人为转换,所以就称作为自动装箱和拆箱。
相反的,当将一个Integer对象赋给一个int值时,将会自动地拆箱。例如:int n = list.get(i); 自动地装换成 int n = list.get(i).intValue();
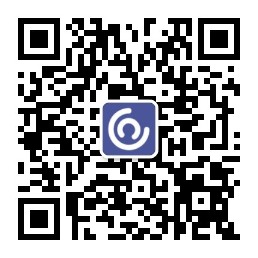
原理:
自动拆装箱都是由编译器自动完成地,而不是虚拟机。编译器在生成类地字节码时,插入必要的方法调用。
1)自动装箱时编译器调用valueOf将基本数据类型值转换成对象。
2)同时自动拆箱时,编译器通过调用类似intValue(),doubleValue()这类的方法将对象转换成基本数据类型值。
面试中相关的问题
下面这段代码的输出结果是什么?
public class Main {
public static void main(String[] args) {
Integer i1 = 100;
Integer i2 = 100;
Integer i3 = 200;
Integer i4 = 200;
System.out.println(i1==i2);
System.out.println(i3==i4);
}
}
答案如下:
true
false
输出结果表明i1和i2指向的是同一个对象,而i3和i4指向的是不同的对象。此时只需一看源码便知究竟,下面这段代码是Integer的valueOf方法的具体实现:
public static Integer valueOf(int i) {
if(i >= -128 && i <= IntegerCache.high)
return IntegerCache.cache[i + 128];
else
return new Integer(i);
}
下面是IntegerCache类的实现
private static class IntegerCache {
static final int high;
static final Integer cache[];
static {
final int low = -128;
// high value may be configured by property
int h = 127;
if (integerCacheHighPropValue != null) {
// Use Long.decode here to avoid invoking methods that
// require Integer's autoboxing cache to be initialized
int i = Long.decode(integerCacheHighPropValue).intValue();
i = Math.max(i, 127);
// Maximum array size is Integer.MAX_VALUE
h = Math.min(i, Integer.MAX_VALUE - -low);
}
high = h;
cache = new Integer[(high - low) + 1];
int j = low;
for(int k = 0; k < cache.length; k++)
cache[k] = new Integer(j++);
}
private IntegerCache() {}
}
从这2段代码可以看出,在通过valueOf方法创建Integer对象的时候,如果数值在[-128,127]之间,便返回指向IntegerCache.cache中已经存在的对象的引用;否则创建一个新的Integer对象。
上面的代码中i1和i2的数值为100,因此会直接从cache中取已经存在的对象,所以i1和i2指向的是同一个对象,而i3和i4则是分别指向不同的对象。
2.下面这段代码的输出结果是什么?
public class Main {
public static void main(String[] args) {
Double i1 = 100.0;
Double i2 = 100.0;
Double i3 = 200.0;
Double i4 = 200.0;
System.out.println(i1==i2);
System.out.println(i3==i4);
}
}
答案如下:
false
false
为什么会这样?当然是因为两个类的valueOf()方法不一样。下面是Double类的valueOf()方法。
public static Double valueOf(double d) {
return new Double(d);
}
注意,Integer、Short、Byte、Character、Long这几个类的valueOf方法的实现是类似的。
Double、Float的valueOf方法的实现是类似的。
以及Boolean的 Character的自己可以去试验一下。