作者 | Jeskson
来源 | 达达前端小酒馆
Vue简介
Vue框架,框架的作者,尤雨溪,组件化,快速开发的特点。
生命周期
beforeCreate:组件刚刚被创建
created:组件创建完成
生成
beforeMount:挂载之前
mounted:挂载之后
成熟
beforeDestory:组件销毁前调用
destoryed:组件销毁后调用
老年
安装:
全局安装:vue-cli
npm install --global vue-cli
创建一个基于webpack模板的新项目
vue init webpack my-project
安装依赖包:
cd my-project
npm install
npm run dev
@代表src目录:
import Vue from 'vue'
import Router from 'vue-router'
import Hello from '@/components/Hello'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/',
name: 'hello',
component: Hello
}
]
})
生命周期调用:
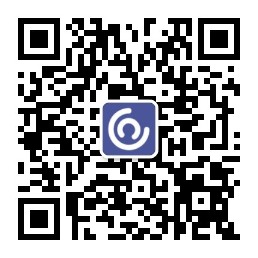
beforeCreate
created
beforeMount
mounted
实例生命周期钩子
比如 created 钩子
new Vue({
data: {
a: 1
},
created: function () {
// `this` 指向 vm 实例
console.log('a is: ' + this.a)
}
})
// => "a is: 1"
生命周期钩子的 this 上下文指向调用它的 Vue 实例。
生命周期图示
选项数据:data,computed,methods
全局组件的变量的定义:
<template>
<div>
<div>{{msg}}</div>
</div>
</template>
<script>
export default{
data(){
return{
msg:'hello',
a: 1,
}
}
}
</script>
computed: {
// 里面函数
msg() {
return this.a * 2;
}
}
@click="say('hi')"
methods: {
say(){
}
}
模板语法
data,模板中直接嵌入js代码,指令,v-html,v-on,v-bind等,计算属性,过滤器。
<div>{{number+1}}</div>
v-on:click=""
简写
@click
{{msg.split('').reverse().join('')}}
this.msg.split('').reverse().join('');
class与style绑定
<div class="static"
v-bind:class="{ active: isActive, 'text-danger': hasError }">
class1
</div>
data(){
return{
isActive: true,
hasError: false
}
}
<div class="active text-danger">
</div>
<div v-bind:style="{color: activeColor, fontSize: fontSize+'px'}">
原始的写法:
<div style="color: red; fontSize: 12px">
dada
</div>
<div v-bind:style="{ 'color': activeColor, 'fontSize': fontSize + 'px' }">
style
</div>
<div v-bind:style="styleObject">style2</div>
<div v-bind:style=[baseStyles, overridingStyles]">
style3
</div>
条件渲染
v-if指令
v-if v-else
v-if v-else-if v-else
<h1 v-if="ok">dada</h1>
<script>
export default{
data(){
ok: true
}
}
</script>
<div v-if="type==='A'">
A
</div>
<div v-else-if="type === 'B'">
B
</div>
<div v-else-if="type==='C'">
C
</div>
<div v-else>
D
</div>
v-show和v-if意思一致
列表渲染
v-for指令
v-for="item in items" 数组
v-for="(item, index) in items" 数组
v-for="(value,key) in object" 对象
<ul id="">
<li v-for="(item, index) in items">
{{index}}-{{item.message}}
</li>
</ul>
<li v-for="item in items">
{{item.message}}
</li>
<div id="">
<li v-for="(value, key) in obj">
{{key}}-{{value}}
</li>
</div>
事件处理器
v-on指令,缩写@
v-on:click 或 @click
<button v-on:click="counter+=1"></button>
<p>{{counter}}</p>
自定义组件
组件写在components文件夹下,组件基本要素,props,$emit等,通过import导入自定义的组件。
<script>
import countdown from '@/components/countdown.vue'
export default{
data(){
return{
}
},
components:{
}
}
</script>
<template>
<p>{{time}}</p>
</template>
<script>
export default{
data(){
return {
time: 10
}
},
mounted(){
var vm = this;
var t = setInterval(function(){
vm.time--;
if(vm.time==0){
clearInterval(t);
vm.$emit("end");
}
},1000)
}}
</script>
Vue中的Dom操作
<div ref="head"></div>
this.$refs.head.innerHTML = ‘dada’;
过渡效果
过渡transition
样式方式写过渡
<button v-on:click="show = !show">
dada
</button>
<transition name="dada">
<p v-if="show">dada</p>
</transition>
路由vue-router
router-link
npm install 引入vue-router包
<router-link to="/demo">dada</router-link>
<router-link :to="{name: 'demo', params: {userId: 123}}>
da
</router-link>
<router-link :to="{name:'demo',params:{userId:123},
query: { plan: 'pri'}}">da< /router-link>
toUrl(){
this.$router.push({path: 'dada' })
}
状态管理
npm install 引入 vuex包
全局的状态管理,所有页面共享数据
设置数据:
this.$store.dispatch("dada", 1222);
获取数据:
this.$store.state.num
export default new Vuex.Store({
state: {
count: 0,
num: 1
},
mutations: {
increment(state,num){
state.count++
state.num = num
}
},
actions: {
inc ({commit}, obj){
commit('increment',obj)
}
}
})
this.$store.dispatch('inc', 111);
slot插槽
常用语组件调用
import slots from '@/components/slot.vue'
<template>
<div>
<slots>
da
</slots>
</div>
</template>
vue-resource请求
npm install 引入 vue-resource包
this.$http.get('/daurl')
this.$http.post('/daurl', {foo:'bar'})
this.$http.get('/dadaurl').then(response => {
console.log(response.body);
}, response => {
// error
});
}
}
this.$http.post('/daurl', {foo: 'bar'}).then(response => {
console.log(response.body);
},response => {
// error callback
});
移动组件库mint UI
地址:
http://mint-ui.github.io/docs/#/zh-cn
❤️ 不要忘记留下你学习的脚印 [点赞 + 收藏 + 评论]
作者Info:
【作者】:Jeskson
【原创公众号】:达达前端小酒馆。
【福利】:公众号回复 “资料” 送自学资料大礼包(进群分享,想要啥就说哈,看我有没有)!
【转载说明】:转载请说明出处,谢谢合作!~
大前端开发,定位前端开发技术栈博客,PHP后台知识点,web全栈技术领域,数据结构与算法、网络原理等通俗易懂的呈现给小伙伴。谢谢支持,承蒙厚爱!!!
若本号内容有做得不到位的地方(比如:涉及版权或其他问题),请及时联系我们进行整改即可,会在第一时间进行处理。
请点赞!因为你们的赞同/鼓励是我写作的最大动力!
欢迎关注达达的CSDN!
这是一个有质量,有态度的博客