安装
- 直接下载(官方CDN)
https://unpkg.com/vue-router/...
通过页面script标签引入,如下:
<script src='https://unpkg.com/vue-router/dist/vue-router.js'></script>
- NPM安装
npm install vue-router --save-dev
安装完成后需要Vue.use()安装此功能,例如:
import Vue from 'vue';
import VueRouter from 'vue-router';
Vue.use(VueRouter);
入门
- 简单例子
<html>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<title>vue1217</title>
</head>
<body>
<div id="app"></div>
<!-- built files will be auto injected -->
</body>
</html>
<App.vue>
<template>
<div>
<p>
<router-link to="/foo">Foo Link</router-link>
<router-link to="/bar">Bar Link</router-link>
</p>
<router-view></router-view>
</div>
</template>
<script type="text/ecmascript-6">
export default {};
</script>
<style lang="stylus" rel="stylesheet/stylus">
</style>
<main.js>
// The Vue build version to load with the `import` command
// (runtime-only or standalone) has been set in webpack.base.conf with an alias.
import Vue from 'vue';
import VueRouter from 'vue-router';
import App from './App';
Vue.use(VueRouter);
const foo = {
template: '<div>foo</div>'
};
const bar = {
template: '<div>bar</div>'
};
const routes = [{
path: '/foo',
component: foo
}, {
path: '/bar',
component: bar
}];
let router = new VueRouter({
routes
});
/* eslint-disable no-new */
new Vue({
el: '#app',
router,
render: h => h(App)
});
- 动态路由匹配
通过带有冒号标记的属性匹配,例如
path: '/foo/test0'
path: '/foo/test1'
都可以被
path: '/foo/:id'
匹配到
并且被匹配到的组件内部可以通过
this.$route.params获取到被匹配的参数
如上:
test0组件下的params参数为
{
id: 'test0'
}
同样的:
/foo/test0/jason/0411
被
/foo/:id/:name/:birth
匹配到的参数如
{
id: 'test0',
name: 'jason',
birth: '0411'
}
小提示:对于通过路由进行组件间的切换时,并不会来回构建组件实例,而是复用已存在的组件,想要监听路由参数变化可以通过watch属性或者通过beforeRouteUpdate钩子函数做操作
{
watch: {
'$route'(to, from) {
// something to do
}
}
}
{
beforeRouteUpdate(to, from, next) {
// something to do
next();// next必须执行不然的话beforeRouteUpdate钩子函数不会resolved
}
}
- 嵌套路由
顾名思义就是路由跳到的组件又包含有路由。
举个栗子:
<App.vue>
<template>
<div>
<p>
<router-link to="/foo">foo</router-link>
<router-link to="/foo1/hm">foo1</router-link>
<router-link to="/foo2/pf">foo2</router-link>
<router-link to="/foo3/ps">foo3</router-link>
</p>
<router-view></router-view>
</div>
</template>
const foo = {
template: `
<div>
<p>{{ $route.params.id }}</p>
<router-view></router-view>
</div>
`
};
<main.js>
const home = {
template: '<div>home</div>'
};
const hm = {
template: '<div>hm</div>'
};
const pf = {
template: '<div>pf</div>'
};
const ps = {
template: '<div>ps</div>'
};
const routes = [{
path: '/:id',
component: foo,
children: [
{
path: '',
component: home
}, {
path: 'hm',
component: hm
}, {
path: 'pf',
component: pf
}, {
path: 'ps',
component: ps
}
]
}];
- 通过$router导航
在组件Vue实例内部可以通过
this.$router.push(location, onComplete?, onAbort?)
this.$router.replace(location, onComplete?, onAbort?)
this.$router.go(n)
// 字符串
router.push('home')
// 对象
router.push({ path: 'home' })
// 命名的路由
router.push({ name: 'user', params: { userId: 123 }})
// 带查询参数,变成 /register?plan=private
router.push({ path: 'register', query: { plan: 'private' }})
const userId = 123
router.push({ name: 'user', params: { userId }}) // -> /user/123
router.push({ path: `/user/${userId}` }) // -> /user/123
// 这里的 params 不生效
router.push({ path: '/user', params: { userId }}) // -> /user
// 在浏览器记录中前进一步,等同于 history.forward()
router.go(1)
// 后退一步记录,等同于 history.back()
router.go(-1)
// 前进 3 步记录
router.go(3)
// 如果 history 记录不够用,那就默默地失败呗
router.go(-100)
router.go(100)
- 命名路由
在创建路由的时候可以通过name属性设置路由名称
let routes = [{
path: '/foo/:userId',
name: 'test',
component: User
}];
如果需要链接到这个命名路由我们可以酱紫
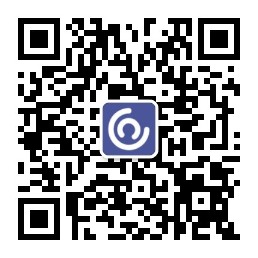
<router-link :to="{ 'name' : 'test', 'params' : { 'userId' : 410100 }}"></router-link>
也可以酱紫
router.push({
name: 'test',
params: {
userId: 410100
}
});
- 命名视图
很多时候我们会碰到并存的多个视图(router-view),我们就需要为视图设置name没有设置的为default
<router-view class="view one"></router-view>
<router-view class="view two" name="a"></router-view>
<router-view class="view three" name="b"></router-view>
当然构建路由的时候也需要设置对应视图的组件映射
const router = new VueRouter({
routes: [
{
path: '/',
components: {
default: Foo,
a: Bar,
b: Baz
}
}
]
})
- 重定向
let routes = [{
path: '/',
redirect: '/goods'
},{
path: '/goods',
component: goods
}];
当path为/的时候会自动重定向至/goods加载goods组件
当然也可以通过name重定向,作用是相同的
let routes = [{
path: '/',
redirect: {
name: 'goods'
}
},{
path: '/goods',
name: 'goods',
component: goods
}];
当然也可以是一个方法
let routes = [{ path: '/a', redirect: to => {
// 方法接收 目标路由 作为参数
// return 重定向的 字符串路径/路径对象
}}];
- 别名
别名与重定向只有一丢丢不同,重定向指/a路径调到/b路径加载,别名指还是加载/a路径只是加载内容是/b路径下的
const router = new VueRouter({
routes: [{
path: '/a',
component: A,
alias: '/b'
}]
});
5.路由参数传递
有三种模式进行传递:布尔模式、对象模式、函数模式
布尔模式: 如果props被设置为true,route.params将会被设置为组件属性。
router-link的路径
<router-link to="/foo/params">foo</router-link>
foo的路由
{
path: '/foo/:userName',
name: 'foo',
component: foo,
props: true
}
foo组件
<template>
<div>
foo
<div>{{userName}}</div>
</div>
</template>
<script type="text/ecmascript-6">
export default {
props: {
userName: {
type: String,
default: ''
}
}
};
</script>
点击router-link后的结果
你会发现/foo/params被path匹配后
route.params = {
userName: 'params'
};
props中的userName就会接收到字符串'params',因此输出结果如图所示
对象模式:如果props是一个对象,它会被按原样设置为组件属性。
{
path: '/foo',
name: 'foo',
component: foo,
props: {
userName: 'From Obj'
}
}
渲染后的结果:
函数模式
{
path: '/foo',
name: 'foo',
component: foo,
props: (route) => ({userName: route.query.name})
}
看起来还是很清楚的,只是props会接收一个当前的路由参数,将参数中的值转换成你想要的值,以上三种模式自然也不影响通过router-view的v-bind:user-name这种方式传递,但是对于同名的参数值会有影响。
let lastProps = {};
let vBindProps = {
userName: 'vBind',
name: 'vBind'
};
let routeProps ={
userName: 'route'
};
Object.assign(lastProps,vBindProps,routeProps);
// {userName: "route", name: "vBind"}
最终的lastProps就是传入的props