使用sklearn可以实现线性回归算法,新手练习写一个类似于线性回归的算法,进行比较。
1、调用sklearn中的LinearRegression:
导包:
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
from sklearn.linear_model import LinearRegression
生成数据:
X = np.linspace(2.5,12,25)
w = np.random.randint(2,10,size = 1)[0]
b = np.random.randint(-5,5,size = 1)[0]
y = X*w + b +np.random.randn(25)*2
#使用np.random.randn(25)*2,使数据变得随机
显示数据图:
plt.scatter(X,y)
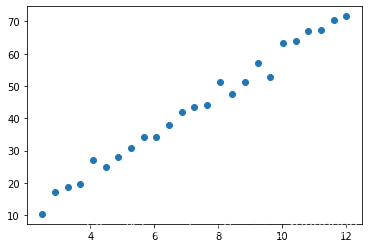
调用库线性回归函数
lr = LinearRegression()
#X必须是二维
lr.fit(X.reshape(-1,1),y)
显示斜率和截距
lr.coef_
lr.intercept_
2、实现线性回归函数
class Linear_model(object):
def __init__(self):
self.w = np.random.randn(1)[0]
self.b = np.random.randn(1)[0]
print('----------------------起始随机生成的斜率和截距',self.w,self.b)
#model就是方程:f(x) = wx + b
def model(self,x):
return self.w * x +self.b
#线性问题,原理都是最小二乘法:
def loss(self,x,y):
#在这个方程中,有几个未知数?
cost = (y - self.model(x))**2
#求偏导数,把其他的都当作已知数,求一个未知数
g_w = 2*(y-self.model(x))*(-x)
g_b = 2*(y - self.model(x))*(-1)
return g_w,g_b
#梯度下降
def gradient_descent(self,g_w,g_b,step = 0.01):
#更新新的斜率和截距:
self.w = self.w - g_w*step
self.b = self.b - g_b*step
print('----------------------更新',self.w,self.b)
def fit(self,X,y):
w_last = self.w + 1
b_last = self.b + 1
precision = 0.00001
max_count = 3000
count = 0
while True:
if (np.abs(self.w - w_last) < precision) and (np.abs(self.b - b_last) < precision):
break
if count > max_count:
break
#更新斜率和截距:
g_w = 0
g_b = 0
size = X.shape[0]
for xi,yi in zip(X,y):
g_w += self.loss(xi,yi)[0] / size
g_b += self.loss(xi,yi)[1] / size
self.gradient_descent(g_w,g_b)
count += 1
def coef_(self):
return self.w
def intercept_(self):
return self.b
调用
lm = Linear_model()
lm.fit(X,y)
查看斜率和截距
lm.coef_()
lr.intercept_
程序均在jupyter中实现