题目:
Say you have an array for which the ith element is the price of a given stock on day i.
If you were only permitted to complete at most one transaction (i.e., buy one and sell one share of the stock), design an algorithm to find the maximum profit.
Note that you cannot sell a stock before you buy one.
Example 1:
Input: [7,1,5,3,6,4]
Output: 5
Explanation: Buy on day 2 (price = 1) and sell on day 5 (price = 6), profit = 6-1 = 5.
Not 7-1 = 6, as selling price needs to be larger than buying price.
Example 2:
Input: [7,6,4,3,1]
Output: 0
Explanation: In this case, no transaction is done, i.e. max profit = 0.
解题思路:
在给定的数组中,找出前后两个数字相差最大的值(可以不相邻),把这个差值输出出来。
第一种解法:
public int maxProfit(int[] prices) {
if (prices.length < 2) {
return 0;
}
int[] result = new int[prices.length];
Arrays.fill(result, 0);
for (int i = 0; i < prices.length; i++) {
for (int j = 0; j < i; j++) {
int temp = prices[i] - prices[j];
if (temp > 0 && result[i] < temp) {
result[i] = temp;
}
}
}
Arrays.sort(result);
return result[result.length - 1];
}
这是自己一开始的解法,想要采取动态规划的方法,对数组本身进行二次遍历,把每一个数的最大差值都求出来,放在新的数组中,最后数组中的最大的数,即为最大差值。
后面又参考了网上大神的思路
扫描二维码关注公众号,回复:
8560026 查看本文章
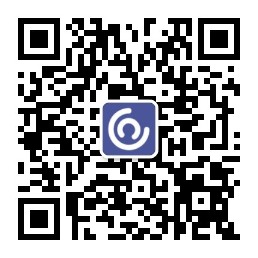
第二种解法:
public int maxProfit(int[] prices) {
int ans = 0;
// 边界值判断
if (prices.length == 0) {
return ans;
}
// 默认第一个为买入的值
int bought = prices[0];
for (int i = 1; i < prices.length; i++) {
if (prices[i] > bought) {
// 如果后面的数大于前面的数并且后面的数和前面数的差大于之前记录的差值
if (ans < (prices[i] - bought)) {
// 使用当前差值替换现有差值
ans = prices[i] - bought;
}
} else {
// 后面的数小于前面的数,用小的数替换买入值
bought = prices[i];
}
}
return ans;
}
对于这道题来讲,只要自身遍历一遍,把数组中的最大最小值获取到,返回两者的差值。无论是空间复杂度还是时间复杂度都做到了简化。
时间复杂度:O(n^2)-->O(n)
空间复杂度:虽然都是O(n),但是第二种方式减少了记录差值的数组