- 简单的介绍一下,管道通信类 指的是 IPC通信中的一种,即两个不同的进程之间的通信
- 现在实现一下管道通信类,方便以后调用使用
- 头文件(引用相关的库)
#pragma once
#include "targetver.h"
#include <stdio.h>
#include <tchar.h>
#include <iostream>
#include <Windows.h>
#include <string>
#include <memory>
#include "stdafx.h"
enum PIPEUSERTYPE{
USER_CLIENT,
USER_SERVER,
};
class PipeIPC{
public:
PipeIPC();
~PipeIPC();
bool InitPipeIPC(std::wstring pipe_name,PIPEUSERTYPE states);
bool WriteData(std::string datas);
bool ReadData(std::string& datas);
private:
std::wstring m_pipe_name;
HANDLE m_hPipeHandle;
PIPEUSERTYPE m_state;
};
- 类的声明也很简单,主要实现了写入数据和读出数据 这两个操作,至少写入数据的格式之类的,其实都随便=== 理解原理就很简单
- 接下来是实现
#include "stdafx.h"
#include "PipeDemo.h"
using namespace std;
PipeIPC::PipeIPC()
{
m_pipe_name = L"";
m_hPipeHandle = nullptr;
m_state = USER_CLIENT;
}
PipeIPC::~PipeIPC()
{
DisconnectNamedPipe(m_hPipeHandle);
CloseHandle(m_hPipeHandle);
}
bool PipeIPC::InitPipeIPC(std::wstring pipe_name,PIPEUSERTYPE states)
{
m_state = states;
std::wstring spice_name = L"\\\\.\\pipe\\";
spice_name.append(pipe_name);
m_pipe_name.append(spice_name);
if (m_state == USER_SERVER)
{
m_hPipeHandle = CreateNamedPipe(m_pipe_name.c_str(),PIPE_ACCESS_DUPLEX,
PIPE_TYPE_MESSAGE|PIPE_READMODE_MESSAGE|PIPE_WAIT,
PIPE_UNLIMITED_INSTANCES,
0,
0,
NULL,nullptr);
if (m_hPipeHandle == INVALID_HANDLE_VALUE)
{
cout << "" << endl;
return false;
}
cout << "初始化服务端PIPE成功!" << endl;
}
else
{
m_hPipeHandle = CreateFile(
m_pipe_name.c_str(),
GENERIC_READ |
GENERIC_WRITE,
0,
NULL,
OPEN_EXISTING,
FILE_ATTRIBUTE_NORMAL,
NULL);
if (m_hPipeHandle == INVALID_HANDLE_VALUE)
{
return false;
}
cout << "初始化客户端PIPE成功!" << endl;
}
return true;
}
bool PipeIPC::ReadData(std::string& datas)
{
DWORD rLen = 0;
char szBuffer[4096] = {0};
if (!ReadFile(m_hPipeHandle,szBuffer,4096,&rLen,nullptr))
{
cout << "读取失败" << endl;
}
cout << "读取数据为:" << szBuffer << endl;
datas = szBuffer;
return true;
}
bool PipeIPC::WriteData(std::string datas)
{
if (m_state == USER_SERVER)
{
if (!ConnectNamedPipe(m_hPipeHandle,NULL))
{
cout << "客户端还没连接" << endl;
return false;
}
}
DWORD wLen;
WriteFile(m_hPipeHandle,datas.c_str(),strlen(datas.c_str()),&wLen,NULL);
return true;
}
unique_ptr<PipeIPC> m_p(new PipeIPC());
std::wstring Names = L"NAMEs11s";
std::string datas = "ddsss";
m_p->InitPipeIPC(Names,USER_SERVER);
m_p->WriteData(datas);
m_p->ReadData(datas);
system("pause");
unique_ptr<PipeIPC> m_p(new PipeIPC());
std::wstring Names = L"NAMEs11s";
std::string datas = "ddsss";
m_p->InitPipeIPC(Names,USER_CLIENT);
m_p->ReadData(datas);
std::string datasss = "ceshi";
m_p->WriteData(datasss);
system("pause");
return 0;
- 运行结果截图:
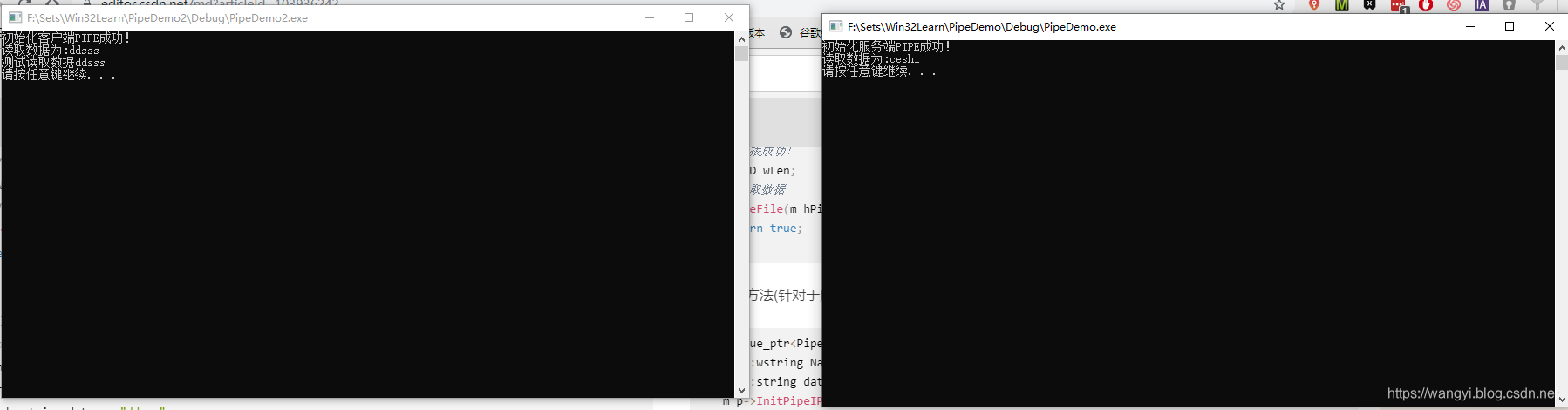