一、前言
1.本次只是简单的整合了 Spring和MybatisPlus,并做了最简单的测试
2.想要整合Spring和MybatisPlus,首先要熟悉Spring框架
3.使用maven项目构建工具
二、步骤
1.创建工程就省略了,简单展示一下目录结构。。。
2.首先在pom文件添加依赖
<dependencies>
<!-- mp 依赖 -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus</artifactId>
<version>2.3</version>
</dependency>
<!--junit -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.9</version>
</dependency>
<!-- log4j -->
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
<!-- c3p0连接池 -->
<dependency>
<groupId>com.mchange</groupId>
<artifactId>c3p0</artifactId>
<version>0.9.5.2</version>
</dependency>
<!-- mysql驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.6</version>
</dependency>
<!-- spring相关 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>4.2.4.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-orm</artifactId>
<version>4.2.4.RELEASE</version>
</dependency>
</dependencies>
3.学过mybatis的都应该知道,mybatis有一个全局配置文件,但是与Spring整合后里面不需要添加内容,但是我们一般都要保留。在resource目录下建一个mybatis-config.xml
这个xml文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
</configuration>
4.resource目录下建一个Spring的核心文件applicationContext.xml
这个xml文件。
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:tx="http://www.springframework.org/schema/tx" xmlns:util="http://www.springframework.org/schema/util"
xmlns="http://www.springframework.org/schema/beans" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
xmlns:mybatis-spring="http://mybatis.org/schema/mybatis-spring"
xsi:schemaLocation="http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.2.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.2.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.2.xsd http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-4.2.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.2.xsd http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd http://mybatis.org/schema/mybatis-spring http://mybatis.org/schema/mybatis-spring-1.2.xsd">
<!--引入properties配置文件-->
<context:property-placeholder location="classpath:db.properties" />
<!--配置数据源,使用C3p0连接池-->
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="${jdbc.driver}"></property>
<property name="jdbcUrl" value="${jdbc.url}"></property>
<property name="user" value="${jdbc.username}"></property>
<property name="password" value="${jdbc.password}"></property>
</bean>
<!--事务管理器-->
<bean id="dataSourceTransactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"></property>
</bean>
<!--开启基于注解的配置-->
<tx:annotation-driven transaction-manager="dataSourceTransactionManager"/>
<!--整合Mybatis-->
<!--Spring一启动就会创建sqlSessionFactory对象-->
<!--
mybatis提供的:org.mybatis.spring.SqlSessionFactoryBean
mybatisPlus 提供的:com.baomidou.mybatisplus.spring.MybatisSqlSessionFactoryBean -->
<bean id="sqlSessionFactory" class="com.baomidou.mybatisplus.spring.MybatisSqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"></property>
<!--配置mybatis的全局配置文件-->
<property name="configLocation" value="classpath:mybatis-config.xml"></property>
</bean>
5.resource目录下建一个db.proerties
这个properties文件
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/db_mybatis_plus?characterEncoding=utf-8
jdbc.username=root
jdbc.password=自己数据的密码
6.为了我们测试方便,添加一个日志文件。resource目录下建一个log4j.xml
这个xml文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE log4j:configuration SYSTEM "log4j.dtd">
<log4j:configuration
xmlns:log4j="http://jakarta.apache.org/log4j/">
<appender name="STDOUT"
class="org.apache.log4j.ConsoleAppender">
<param name="Encoding" value="UTF-8" />
<layout class="org.apache.log4j.PatternLayout">
<param name="ConversionPattern"
value="%-5p %d{MM-dd HH:mm:ss,SSS} %m (%F:%L) \n" />
</layout>
</appender>
<logger name="java.sql">
<level value="debug" />
</logger>
<logger name="org.apache.ibatis">
<level value="info" />
</logger>
<root>
<level value="debug" />
<appender-ref ref="STDOUT" />
</root>
</log4j:configuration>
7.既然要测试,我们就要准备数据库表和实体类
(1)数据库表,使用的是mysql数据库,创建一个新的数据库和表,并添加了几条数据。
CREATE DATABASE db_mybatis_plus;
USE db_mybatis_plus;
CREATE TABLE tbl_employee(
id INT(11) PRIMARY KEY AUTO_INCREMENT,
last_name VARCHAR(50), email VARCHAR(50),
gender INT(1), age INT
);
INSERT INTO tbl_employee(last_name,email,gender,age) VALUES('Tom','[email protected]',1,22);
INSERT INTO tbl_employee(last_name,email,gender,age) VALUES('Jerry','[email protected]',0,25);
INSERT INTO tbl_employee(last_name,email,gender,age) VALUES('rose','[email protected]',1,30);
INSERT INTO tbl_employee(last_name,email,gender,age) VALUES('jack','[email protected]',0,35);
(2)Java实体类,对应数据库表字段。
private Integer id;
private String lastName;
private String email;
private Integer gender;
private Integer age;
//无参构造
//有参构造
//getter和setter方法
//toString 方法
//太占地了,我就省略l,但是你不要省略,特别的setter和getter方法。
(3)创建个mapper接口,继承MybatisPlus提提供的BaseMapper接口,泛型是实体类对象
EmployeeMapper
public interface EmployeeMapper extends BaseMapper<Employee>{
}
不放心,来张图
三、测试
1.首先测试一下连接数据库成功没有
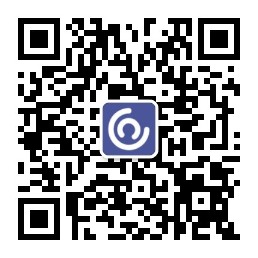
//获取Spring容器
private ApplicationContext ioc = new ClassPathXmlApplicationContext("applicationContext.xml");
private EmployeeMapper employeeMapper = ioc.getBean("employeeMapper",EmployeeMapper.class);
写个测试方法
@Test
public void testMp() throws SQLException {
DataSource dataSource = ioc.getBean("dataSource",DataSource.class);
System.out.println(dataSource);
Connection connection = dataSource.getConnection();
System.out.println(connection);
connection.close();
}
来张图,没有报错,八成就是配置没有问题
日志效果
2.测试个insert插入方法的使用把,当然这么直接测试八成会报错
3.报错信息
org.mybatis.spring.MyBatisSystemException: nested exception is org.apache.ibatis.reflection.ReflectionException: Could not set property 'id' of 'class com.atguigu.mp.beans.Employee' with value '1237661424084324353' Cause: java.lang.IllegalArgumentException: argument type mismatch
4.解决办法,在实体类的id属性上加注解,声明主键是自增长策略
@TableId(type = IdType.AUTO)
5.再次运行还会抛出一个异常
com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException: Table 'mp.employee' doesn't exist
解决办法,在实体类类名哪加注解,声明实体类对应数据库表(因为实体类名和表名不一致,如果一直不会抛出这个异常)
@TableName(value = "tbl_employee")
这两个异常,当然还有更简单的解决方法,这里就先不提了
6.在运行,这次会成功,返回结果为1,并且打印出SQL语句
大功告成
写作不易,如果对您有所帮助,请给一个小小的赞,您的点赞是对我莫大的支持和鼓励,谢谢!!!