The factorial of a number N (written N!) is defined as the product of all the integers from 1 to N. It is often defined recursively as follows:
1! = 1
N! = N ∗ (N − 1)!
Factorials grow very rapidly — 5! = 120, 10! = 3, 628, 800. One way of specifying such large numbers is by specifying the number of times each prime number occurs in it, thus 825 could be specified as (0 1 2 0 1) meaning no twos, 1 three, 2 fives, no sevens and 1 eleven.
Write a program that will read in a number N (2 ≤ N ≤ 100) and write out its factorial in terms of the numbers of the primes it contains.
Input
Input will consist of a series of lines, each line containing a single integer N. The file will be terminated by a line consisting of a single ‘0’.
Output
Output will consist of a series of blocks of lines, one block for each line of the input. Each block will start with the number N, right justified in a field of width 3, and the characters ‘!’, space, and ‘=’. This will be followed by a list of the number of times each prime number occurs in N!.
These should be right justified in fields of width 3 and each line (except the last of a block, which may be shorter) should contain fifteen numbers. Any lines after the first should be indented.
Follow the layout of the example shown below exactly.
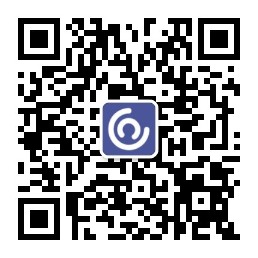
Sample Input
5
53
0
Sample Output
5! = 3 1 1
53! = 49 23 12 8 4 4 3 2 2 1 1 1 1 1 1
1
问题链接:UVA160 Factors and Factorials
问题简述:
求出n!的所有素数因子个数,按照 2 ,3,5,7...的顺序输出因子个数。
问题分析:
筛选法是必要的,先找出素数。
输出格式比较麻烦。
需要主要的一点是,需要多算一个素数!
题记:(略)
参考链接:(略)
/* UVA160 Factors and Factorials */ #include <iostream> #include <math.h> #include <string.h> using namespace std; const int N = 100 + 2; const int SQRTN = ceil(sqrt((double) N)); bool isnotPrime[N + 1]; int prime[N / 2], pcnt; int ans[N + 1]; // Eratosthenes筛选法 void esieve(void) { memset(isnotPrime, false, sizeof(isnotPrime)); isnotPrime[0] = isnotPrime[1] = true; for(int i=2; i<=SQRTN; i++) { if(!isnotPrime[i]) { for(int j=i*i; j<=N; j+=i) //筛选 isnotPrime[j] = true; } } pcnt = 0; for(int i = 2; i <= N; i++) if(!isnotPrime[i]) prime[pcnt++] = i; } int main() { esieve(); int n; while(cin >> n && n) { memset(ans, 0, sizeof(ans)); for(int i = 2; i <= n; i++) { int m = i, now = 0; while(m > 1) { while(m % prime[now] == 0) { ans[now]++; //质因子加1 m /= prime[now]; } now++; } } // 输出结果 printf("%3d! =", n); for(int j = 0; prime[j] <= n; j++) { printf("%3d", ans[j]); if((j + 1) % 15 == 0) { // 每行输出15个, printf("\n"); if(prime[j + 1] <= n) // 后面还有数的话,输出下一行前置空格 printf(" "); } } if(prime[14] > n || prime[15] <= n) // 判断是否需要换行 printf("\n"); } return 0; }