默认为递增顺序;注:一下例子希望自己再次复习时,可以用笔在纸上画画内存图。
排序方法:(比较) | 排序思想: |
选择排序 | 每一次从待排序的数据元素中选出最小(或最大)的一个元素,存放在序列的起始位置,直到全部待排序的数据元素排完。 |
冒泡排序 | 它重复地走访过要排序的数列,一次比较两个元素,如果它们的顺序错误就把它们交换过来。走访数列的工作是重复地进行直到没有再需要交换,也就是说该数列已经排序完成。 |
插入排序 | 通过构建有序序列,对于未排序数据,在已排序序列中从后向前扫描,找到相应位置并插入。 |
快速排序 | 通过一趟排序将待排记录分隔成独立的两部分,其中一部分记录的关键字均比另一部分的关键字小,则可分别对这两部分记录继续进行排序,以达到整个序列有序。 |
<--------------------------------------选择排序--------------------------------------->
1、选择排序:
选择排序的思想是,每一次从待排序的数据元素中选出最小(或最大)的一个元素,存放在序列的起始位置,直到全部待排序的数据元素排完。
选择排序1:
private static void selectionSort(int[] a){
for(int i = 0;i<a.length;i++){
for(int j = i+1;j<a.length;j++){
if( a[j] <a[i]){
int temp = a[i];
a[i] = a[j];
a[j] = temp;
}
}
}
}
选择排序2(最优):
private static void OptimizeNumSort(int[] a){
int k,temp;// k and temp is not need to allocation internal storage everytime.
for(int i=0;i<a.length;i++){
k = i; //use k record minimum number at present ,hypothesis i is minimum./用k记录那个最小的数
for(int j = k+1;j<a.length;j++){
if(a[j]<a[k]){
k = j;
}
}
if(i != k ){
temp = a[i];
a[i] = a[k];
a[k] = temp;
}
}
}
<---------------------------------------冒泡排序-------------------------------------------------->
2、冒泡排序:
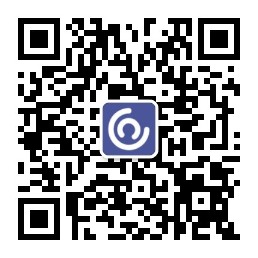
基本思想
设排序表长为n,从后向前或者从前向后两两比较相邻元素的值,如果两者的相对次序不对(A[i-1] > A[i]),则交换它们,其结果是将最小的元素交换到待排序序列的第一个位置,我们称它为一趟冒泡。下一趟冒泡时,前一趟确定的最小元素不再参与比较,待排序序列减少一个元素,每趟冒泡的结果把序列中最小的元素放到了序列的”最前面”。
比较相邻的元素。如果第一个比第二个大,就交换他们两个。
对每一对相邻元素作同样的工作,从开始第一对到结尾的最后一对。在这一点,最后的元素应该会是最大的数。
针对所有的元素重复以上的步骤,除了最后一个。
持续每次对越来越少的元素重复上面的步骤,直到没有任何一对数字需要比较。
冒泡排序是一种简单的排序算法。它重复地走访过要排序的数列,一次比较两个元素,如果它们的顺序错误就把它们交换过来。走访数列的工作是重复地进行直到没有再需要交换,也就是说该数列已经排序完成。这个算法的名字由来是因为越小的元素会经由交换慢慢“浮”到数列的顶端。
算法描述
- 比较相邻的元素。如果第一个比第二个大,就交换它们两个;
- 对每一对相邻元素作同样的工作,从开始第一对到结尾的最后一对,这样在最后的元素应该会是最大的数;
- 针对所有的元素重复以上的步骤,除了最后一个;
- 重复步骤1~3,直到排序完成。
private static void bubbleSort(int[] a){
int len = a.length;
int temp;
for(int i = len-1; i>=1;i--){
for(int j = 0;j<len-1;j++){
if(a[j]>a[j+1]){
temp = a[j];
a[j] = a[j+1];
a[j+1] = temp;
}
}
}
}
来个小练习:对某个类的对象进行排序:
对日期(yeda-month-day)进行排序:
使用到条件运算符(?:)
public int compare(Date date){
return (year>date.year)?1
:year<date.year?-1
:month>date.month?1
:month<date.month?-1
:day>date.day?1
:day<date.day?-1:0;
}
详细如下:
public class Test{
public static void main(String[] args) {
Date[] days = new Date[5];
days[0] = new Date(2006,5,4);
days[1] = new Date(2006,7,4);
days[2] = new Date(2008,5,4);
days[3] = new Date(2004,5,9);
days[4] = new Date(2004,5,4);
for(int i=0;i<days.length;i++ ) {
System.out.println(days[i]);
}
System.out.println("\nUp is not sort---------------------------Down after sort:\n");
//bubbleSort(days);// call the mothod of bubbleSort().
selectionSort(days);// call the mothod of selectionSort().
for(int i=0;i<days.length;i++ ) {
System.out.println(days[i]);
}
}
/*Bubble sort and return sort a type;
learn to bubbleSort' thinking;
every sort will get the maximum.
*/
public static Date[] bubbleSort(Date[] a){
int len = a.length;
for(int i=len-1;i>=1;i--){
for (int j = 0;j<=i-1 ;j++ ) {
if(a[j].compare(a[j+1]) > 0){
Date temp = a[j];
a[j] = a[j+1];
a[j+1] = temp;
}
}
}
return a;
}
/*selection sort */
public static Date[] selectionSort(Date[] a){
int len = a.length;
int k;
Date temp;
for(int i =0 ; i<len;i++){
k = i;
for(int j = k+1;j<len;j++){
if(a[k].compare(a[j]) == 1){
k = j;
}
}
if(k != i)
{
temp = a[k];
a[k] = a[i];
a[i] = temp;
}
}
return a;
}
}
class Date{
int year,month,day;
Date(int y,int m,int d){
year = y;
month = m;
day = d;
}
/*
method compare() mainly to compare two Date objects' size.
if a>b return 1;
else if a<b return -1;
else a = b return 0;
according to return value ,whether to exchange objects'quote
*/
public int compare(Date date){
return (year>date.year)?1
:year<date.year?-1
:month>date.month?1
:month<date.month?-1
:day>date.day?1
:day<date.day?-1:0;
}
/*
remember: when print an objects'quote ,is equal to call toString() method.
so have to overwrite the toString*
another:you can query the API/
*/
public String toString(){
return "Year:Month:Day-- " + year + "-"
+ month + "-" + day;
}
}
<----------------------------------------选择排序------------------------------------------------------------>
选择排序(Selection Sort)
选择排序(Selection-sort)是一种简单直观的排序算法。它的工作原理:首先在未排序序列中找到最小(大)元素,存放到排序序列的起始位置,然后,再从剩余未排序元素中继续寻找最小(大)元素,然后放到已排序序列的末尾。以此类推,直到所有元素均排序完毕。
算法描述
n个记录的直接选择排序可经过n-1趟直接选择排序得到有序结果。具体算法描述如下:
- 初始状态:无序区为R[1..n],有序区为空;
- 第i趟排序(i=1,2,3…n-1)开始时,当前有序区和无序区分别为R[1..i-1]和R(i..n)。该趟排序从当前无序区中-选出关键字最小的记录 R[k],将它与无序区的第1个记录R交换,使R[1..i]和R[i+1..n)分别变为记录个数增加1个的新有序区和记录个数减少1个的新无序区;
- n-1趟结束,数组有序化了。
public class Test{
public static void main(String[] args) {
int[] a = new int[args.length];
for(int i=0;i<args.length;i++){
a[i]=Integer.parseInt(args[i]);
}
print(a);
selectionSort(a);
print(a);
}
/*选择排序*/
private static void selectionSort(int[] a){
System.out.println("Start....");
int minIndex,temp;
for(int i = 0 ;i<a.length;i++){
minIndex = i ;//minIndex 用来保存最小的数的索引
for(int j = i+ 1;j<a.length ;j++){ //i是前一个数的索引,j是后一个数的索引。
if(a[minIndex]>a[j]){
minIndex = j;
}
}
temp = a[i];
a[i] = a[minIndex];
a[minIndex] = temp;
}
}/*输出函数*/
private static void print( int[] a){
for(int i = 0 ;i<a.length ;i++){
System.out.print(a[i]+" ");
}
System.out.println();
}
}
两层for循环,相当于两个指针,最外一个for循环确定前面一个数,里面一个for循环确定后面的一个数,然后两个数用来比较。
<--------------------------------插入排序-------------------------------------->
3、插入排序(Insertion Sort)
插入排序(Insertion-Sort)的算法描述是一种简单直观的排序算法。它的工作原理是通过构建有序序列,对于未排序数据,在已排序序列中从后向前扫描,找到相应位置并插入。
3.1 算法描述
一般来说,插入排序都采用in-place在数组上实现。具体算法描述如下:
- 1、从第一个元素开始,该元素可以认为已经被排序;
- 2、取出下一个元素,在已经排序的元素序列中从后向前扫描;
- 3、如果该元素(已排序)大于新元素,将该元素移到下一位置;
- 4、重复步骤3,直到找到已排序的元素小于或者等于新元素的位置;
- 5、将新元素插入到该位置后;
- 6、重复步骤2~5。
private static void insertionSort(int[] a){
System.out.println("Start....");
int preIndex,current;
for(int i = 1 ;i <a.length;i++){
preIndex = i - 1;
current = a[i];
while(preIndex >= 0 && a[preIndex] >current){
a[preIndex + 1] = a[preIndex];
preIndex --;
}
a[preIndex + 1] = current;
}
}