Android 登录注册页面实现分享
学了一段时间的Android开发,通过写博客记录一下学习过程遇到的问题和一些想要分享的东西。
页面效果如图所示:
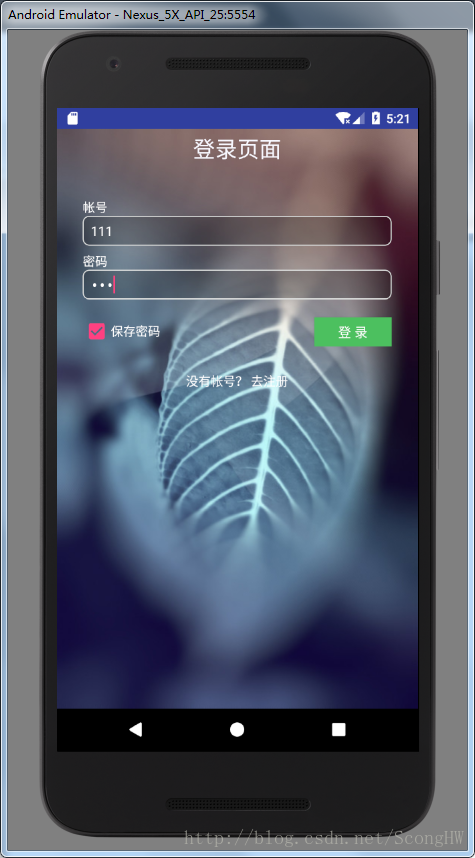
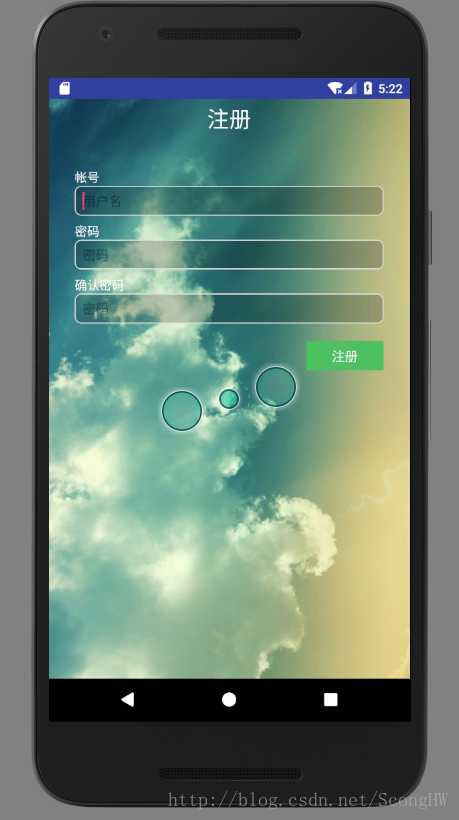
整个登录和注册页面都是连接服务器进行操作的,客户端向服务端发出请求,服务端接收请求并进行相应的处理。登录过程中,客户端将用户输入的账号和密码发送给服务器,服务端查询数据库验证登录是否成功,返回登录结果。注册过程中,服务端先查询用户输入的账号是否存在,若存在则要求用户更改账号名,不存在则对数据库执行插入数据操作,返回注册结果。
1、登录页面代码
public class LoginActivity extends Activity {
private SharedPreferences.Editor editor;
private EditText accountEdit;
private EditText passwordEdit;
private Button login;
private static final int OK = 200;
private CheckBox rememberPass;
private SharedPreferences sp;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
sp = getSharedPreferences("info1.txt", MODE_PRIVATE);
accountEdit = (EditText) findViewById(R.id.user);
passwordEdit = (EditText) findViewById(R.id.pass);
rememberPass = (CheckBox) findViewById(R.id.remember_pass);
login = (Button) findViewById(R.id.login);
boolean isRemember = sp.getBoolean("remember_password", false);
if (isRemember) {
String account = sp.getString("account", "");
String password = sp.getString("password", "");
accountEdit.setText(account);
passwordEdit.setText(password);
rememberPass.setChecked(true);
}
login.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
final String username = accountEdit.getText().toString();
final String password = passwordEdit.getText().toString();
final String serverPath = "http://xxx.xxx.xxx.xxx:8080/ServletTest/login";
if (TextUtils.isEmpty(username) || TextUtils.isEmpty(password)) {
Toast.makeText(LoginActivity.this,"用户名或密码不能为空!",Toast.LENGTH_SHORT).show();
} else {
editor = sp.edit();
if (rememberPass.isChecked()) {
editor.putBoolean("remember_password", true);
editor.putString("account", username);
editor.putString("password", password);
} else {
editor.clear();
}
editor.commit();
new Thread(new Runnable() {
@Override
public void run() {
try {
URL url = new URL(serverPath + "?username=" + username + "&password=" + password);
HttpURLConnection httpURLConnection = (HttpURLConnection) url.openConnection();
httpURLConnection.setRequestMethod("GET");
httpURLConnection.setConnectTimeout(5000);
httpURLConnection.setRequestProperty("User-Agent", "Mozilla/5.0 (Windows NT 6.1; WOW64; rv:22.0) Gecko/20100101 Firefox/22.0");
int responseCode = httpURLConnection.getResponseCode();
if (200 == responseCode) {
InputStream inputStream = httpURLConnection.getInputStream();
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream, "UTF-8"));
final String responseMsg = bufferedReader.readLine();
runOnUiThread(new Runnable() {
@Override
public void run() {
if (responseMsg.equals("true")){
Toast.makeText(LoginActivity.this, "登录成功!", Toast.LENGTH_LONG).show();
}else {
Toast.makeText(LoginActivity.this, "登录失败!", Toast.LENGTH_LONG).show();
}
}
});
bufferedReader.close();
httpURLConnection.disconnect();
} else {
}
} catch (Exception e) {
e.printStackTrace();
}
}
}).start();
}
}
});
TextView tv_register=(TextView) findViewById(R.id.register);
tv_register.setClickable(true);
tv_register.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent2=new Intent(LoginActivity.this,RegisterActivity.class);
startActivity(intent2);
}
});
}
}
2、注册页面代码
public class RegisterActivity extends Activity implements View.OnClickListener {
private EditText user;
private EditText pw;
private EditText pw2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_register);
user = (EditText) findViewById(R.id.et_user_register);
pw = (EditText) findViewById(R.id.et_pass_register);
pw2 = (EditText) findViewById(R.id.et_pass_register2);
Button button = (Button) findViewById(R.id.register);
button.setOnClickListener(this);
}
@Override
public void onClick(View v) {
final String username1 = user.getText().toString().trim();
final String password1 = pw.getText().toString().trim();
final String password2 = pw2.getText().toString().trim();
final String serverPath = "http://xxx.xxx.xxx.xxx:8080/ServletTest/register";
if (TextUtils.isEmpty(username1) || TextUtils.isEmpty(password1) || TextUtils.isEmpty(password2)) {
Toast.makeText(RegisterActivity.this, "请输入用户名,密码和确认密码!!!", Toast.LENGTH_SHORT).show();
} else {
if (!(password1.equals(password2))) {
Toast.makeText(RegisterActivity.this, "两次输入的密码不同,请重新输入!!!", Toast.LENGTH_SHORT).show();
} else {
new Thread(new Runnable() {
@Override
public void run() {
try {
URL url = new URL(serverPath + "?username=" + username1 + "&password=" + password1);
HttpURLConnection httpURLConnection = (HttpURLConnection) url.openConnection();
httpURLConnection.setRequestMethod("GET");
httpURLConnection.setConnectTimeout(5000);
httpURLConnection.setRequestProperty("User-Agent", "Mozilla/5.0 (Windows NT 6.1; WOW64; rv:22.0) Gecko/20100101 Firefox/22.0");
final int responseCode = httpURLConnection.getResponseCode();
if (200 == responseCode) {
InputStream inputStream = httpURLConnection.getInputStream();
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream, "UTF-8"));
final String responseMsg = bufferedReader.readLine();
runOnUiThread(new Runnable() {
@Override
public void run() {
if (responseMsg.equals("equal")){
Toast.makeText(RegisterActivity.this, "账号存在,请更改用户名!!", Toast.LENGTH_LONG).show();
}else if(responseMsg.equals("true")){
Toast.makeText(RegisterActivity.this, "注册成功!", Toast.LENGTH_LONG).show();
Intent intent=new Intent(RegisterActivity.this,LoginActivity.class);
startActivity(intent);
}else {
Toast.makeText(RegisterActivity.this, "注册失败!", Toast.LENGTH_LONG).show();
}
}
});
bufferedReader.close();
httpURLConnection.disconnect();
} else {
}
} catch (Exception e) {
e.printStackTrace();
}
}
}).start();
}
}
}
}
3、在AndroidManifest.xml清单文件中添加Android联网权限
<uses-permission android:name="android.permission.INTERNET" />
注:页面布局比较简单就不给出了