模板可以接受模板参数类型是另一个类模板
如果想在代码中将一个模板类参数用作另一个模板
//: C05:TempTemp.cpp
// From "Thinking in C++, Volume 2", by Bruce Eckel & Chuck Allison.
// (c) 1995-2004 MindView, Inc. All Rights Reserved.
// See source code use permissions stated in the file 'License.txt',
// distributed with the code package available at www.MindView.net.
// Illustrates a template template parameter.
#include <cstddef>
#include <iostream>
using namespace std;
template<class T>
class Array { // A simple, expandable sequence
enum { INIT = 10 };
T* data;
size_t capacity;
size_t count;
public:
Array() {
count = 0;
data = new T[capacity = INIT];
}
~Array() { delete [] data; }
void push_back(const T& t) {
if(count == capacity) {
// Grow underlying array
size_t newCap = 2 * capacity;
T* newData = new T[newCap];
for(size_t i = 0; i < count; ++i)
newData[i] = data[i];
delete [] data;
data = newData;
capacity = newCap;
}
data[count++] = t;
}
void pop_back() {
if(count > 0)
--count;
}
T* begin() { return data; }
T* end() { return data + count; }
};
template<class T, template<class> class Seq>
class Container {
Seq<T> seq;
public:
void append(const T& t) { seq.push_back(t); }
T* begin() { return seq.begin(); }
T* end() { return seq.end(); }
};
int main() {
Container<int, Array> container;
container.append(1);
container.append(2);
int* p = container.begin();
while(p != container.end())
cout << *p++ << endl;
getchar();
} ///:~
输出
1
2
Array类模板是个很平常的序列容器
Container模板包含两个参数
一个参数是它持有的类对象类型
还有一个参数是它持有的类对象类型的序列数据结构
使用固定大小的数组
有一个特别的模板参数表示数组的长度
//: C05:TempTemp2.cpp
// From "Thinking in C++, Volume 2", by Bruce Eckel & Chuck Allison.
// (c) 1995-2004 MindView, Inc. All Rights Reserved.
// See source code use permissions stated in the file 'License.txt',
// distributed with the code package available at www.MindView.net.
// A multi-variate template template parameter.
#include <cstddef>
#include <iostream>
using namespace std;
template<class T, size_t N> class Array {
T data[N];
size_t count;
public:
Array() { count = 0; }
void push_back(const T& t) {
if(count < N)
data[count++] = t;
}
void pop_back() {
if(count > 0)
--count;
}
T* begin() { return data; }
T* end() { return data + count; }
};
template<class T,size_t N,template<class,size_t> class Seq>
class Container {
Seq<T,N> seq;
public:
void append(const T& t) { seq.push_back(t); }
T* begin() { return seq.begin(); }
T* end() { return seq.end(); }
};
int main() {
const size_t N = 10;
Container<int, N, Array> container;
container.append(1);
container.append(2);
int* p = container.begin();
while(p != container.end())
cout << *p++ << endl;
getchar();
} ///:~
输出
1
2
在Container的声明内部
Seq的声明中参数名称不是必需的
但是需要有两个参数来声明数据成员seq
无类型参数N出现在模板型参数前面
在固定大小的Array模板使用一个默认参数
显示了C++语言中适应古怪的举动
//: C05:TempTemp3.cpp {-bor}{-msc}
// From "Thinking in C++, Volume 2", by Bruce Eckel & Chuck Allison.
// (c) 1995-2004 MindView, Inc. All Rights Reserved.
// See source code use permissions stated in the file 'License.txt',
// distributed with the code package available at www.MindView.net.
// Template template parameters and default arguments.
#include <cstddef>
#include <iostream>
using namespace std;
template<class T, size_t N = 10> // A default argument
class Array {
T data[N];
size_t count;
public:
Array() { count = 0; }
void push_back(const T& t) {
if(count < N)
data[count++] = t;
}
void pop_back() {
if(count > 0)
--count;
}
T* begin() { return data; }
T* end() { return data + count; }
};
template<class T, template<class, size_t = 10> class Seq>
class Container {
Seq<T> seq; // Default used
public:
void append(const T& t) { seq.push_back(t); }
T* begin() { return seq.begin(); }
T* end() { return seq.end(); }
};
int main() {
Container<int, Array> container;
container.append(1);
container.append(2);
int* p = container.begin();
while(p != container.end())
cout << *p++ << endl;
getchar();
} ///:~
输出
1
2
不管是在Container中sql的定义
还是在main()中container的定义都使用了默认值
这里使用了默认值
默认参数在一个编辑单元内仅能出现一次的例外
由于标准序列容器 vector list deque 都有一个默认的分配器参数
传递这些序列容器中的一个作为模板参数
程序分别传递vector模板类型参数和list模板类型参数创建Container两个实例
扫描二维码关注公众号,回复:
2934170 查看本文章
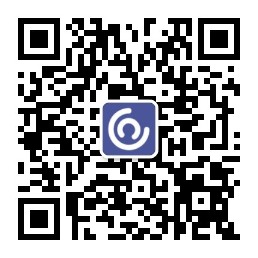
//: C05:TempTemp4.cpp {-bor}{-msc}
// From "Thinking in C++, Volume 2", by Bruce Eckel & Chuck Allison.
// (c) 1995-2004 MindView, Inc. All Rights Reserved.
// See source code use permissions stated in the file 'License.txt',
// distributed with the code package available at www.MindView.net.
// Passes standard sequences as template arguments.
#include <iostream>
#include <list>
#include <memory> // Declares allocator<T>
#include <vector>
using namespace std;
template<class T, template<class U, class = allocator<U> >
class Seq>
class Container {
Seq<T> seq; // Default of allocator<T> applied implicitly
public:
void push_back(const T& t) { seq.push_back(t); }
typename Seq<T>::iterator begin() { return seq.begin(); }
typename Seq<T>::iterator end() { return seq.end(); }
};
int main() {
// Use a vector
Container<int, vector> vContainer;
vContainer.push_back(1);
vContainer.push_back(2);
for(vector<int>::iterator p = vContainer.begin();
p != vContainer.end(); ++p) {
cout << *p << endl;
}
// Use a list
Container<int, list> lContainer;
lContainer.push_back(3);
lContainer.push_back(4);
for(list<int>::iterator p2 = lContainer.begin();
p2 != lContainer.end(); ++p2) {
cout << *p2 << endl;
}
getchar();
} ///:~
这里命名了内部模板Seq的第1个参数
这是因为标准序列容器的分配器必须使用与序列容器中所包含对象的类型相同
的类型对自己参数化
同时,默认的allocator参数是已知的
在随后引用的Seq<T>中省略掉它
输出
1
2
3
4