C++快速入门---assert函数和捕获异常(22)
assert()函数,专为调试而准备的工具函数。
assert()函数需要有一个参数,它将测试这个输入参数的真 or 假状态。
#include <iostream>
#include <cassert>
int main()
{
int i = 20;
assert (i == 65);
return 0;
}
assert()函数可以帮助我们调试程序。可以利用它在某个程序里的关键假设不成立时立刻停止该程序的执行并报错,从而避免发生更严重的问题。
另外,除了结合assert()函数,在程序的开发、测试阶段,我们还可以使用大量的cout语句来报告在程序里正在发生的事情。
捕获异常
为了对付潜在的编程错误(尤其在运行时的错误)。
异常(exception)就是与预期不相符合的反常现象。
扫描二维码关注公众号,回复:
4134110 查看本文章
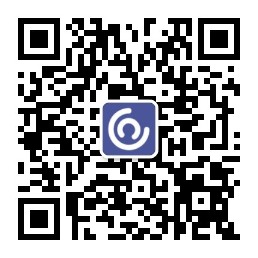
基本使用思路:
1、安排一些C++代码(try语句)去尝试某件事--尤其是那些可能会失败的事(比如打开一个文件或申请一些内存)
2、如果发生问题,就抛出一个异常(throw语句)
3、再安排一些代码(catch语句)去捕获这个异常并进行相应的处理
try
{
//Do something
//Throw an exception on error.
}
catch
{
//Do whatever
}
#include <iostream>
#include <climits>
unsigned long returnFactorial(unsigned short num) throw (const char *);
int main()
{
unsigned short num = 0;
std::cout << "请输入一个整数:";
while (!(std::cin >> num) || (num<1))
{
std::cin.clear(); //清除状态
std::cin.ignore(100, '\n'); //清楚缓冲区
std::cout << "请输入一个整数:";
}
std::cin.ignore(100, '\n');
try
{
unsigned long factorial = returnFactorial(num);
std::cout << num << "的阶乘值是:" << factorial;
}
catch(const char *e)
{
std::cout << e;
}
return 0;
}
unsigned long returnFactorial(unsigned short num) throw (const char *)
{
unsigned long sum = 1;
unsigned long max = ULONG_MAX;
for(int i = 1; i <= num; i++)
{
sum *= i;
max /= i;
}
if (max < 1)
{
throw "悲催。。。该基数太大,无法在该计算机计算求出阶乘值.\n";
}
else
{
return sum;
}
}