输入特性
在主调函数分配内存,被调函数使用
1、在堆区创建
2、在栈上创建
测试源码
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
//二级指针做函数参数的输入特性
//主调函数分配内存,被调函数使用
void printArray( int ** pArray , int len )
{
for (int i = 0; i < len; i++)
{
printf("%d\n", *pArray[i]);
}
}
void test01()
{
//在堆区分配内存
int ** p = malloc(sizeof(int*)* 5);
//在栈上创建数据
int a1 = 10;
int a2 = 20;
int a3 = 30;
int a4 = 40;
int a5 = 50;
p[0] = &a1;
p[1] = &a2;
p[2] = &a3;
p[3] = &a4;
p[4] = &a5;
printArray(p, 5);
if (p!=NULL)
{
free(p);
p = NULL;
}
}
void test02()
{
//在栈上创建
int * pArray[5];
for (int i = 0; i < 5;i++)
{
pArray[i] = malloc(4);
*(pArray[i]) = 100 + i;
}
int len = sizeof(pArray) / sizeof(int*);
printArray(pArray, len);
for (int i = 0; i < 5;i++)
{
if (pArray[i]!= NULL)
{
free(pArray[i]);
pArray[i] = NULL;
}
}
}
int main(){
test01();
test02();
system("pause");
return EXIT_SUCCESS;
}
测试结果
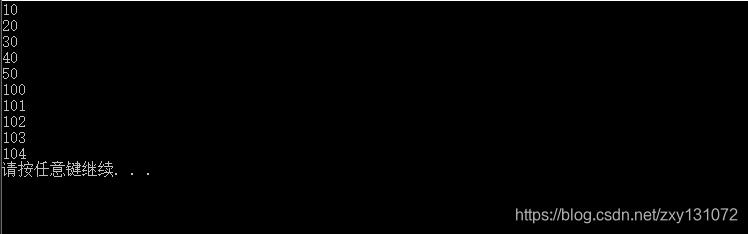
输出特性
在被调函数中分配内存,主调函数使用
测试源码
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
void allocateSpace(int **p)
{
int * arr = malloc(sizeof(int)* 10);
for (int i = 0; i < 10; i++)
{
arr[i] = i + 10;
}
*p = arr;
}
void printArray(int ** pArray, int len)
{
for (int i = 0; i < 10; i++)
{
printf("%d\n", (*pArray)[i]);
}
}
void freeSpace( int ** p )
{
if (*p!= NULL)
{
free(*p);
*p = NULL;
}
}
void test01()
{
int * p = NULL;
allocateSpace(&p);
printArray(&p, 10);
freeSpace(&p);
if (p == NULL)
{
printf("空指针\n");
}
else
{
printf("野指针\n");
}
}
int main(){
test01();
system("pause");
return EXIT_SUCCESS;
}
测试结果
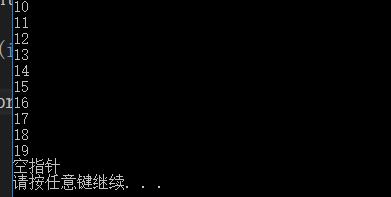