径向渐变
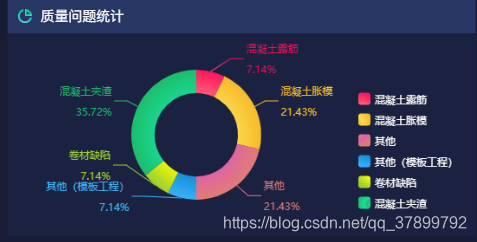
drawPie() {
//var myChart = this.$echarts.init(document.getElementById("echart-pie"));//vue项目
var myChart = echarts.init(document.getElementById("echart-pie"));//jquery
var data = [
{ value: 1, name: "混凝土露筋", color0: "#FF687D", color1: "#FC0C59" },
{ value: 3, name: "混凝土胀模", color0: "#FCDE54", color1: "#F7BA2A" },
{ value: 3, name: "其他", color0: "#df5cb4", color1: "#e07c76" },
{
value: 1,
name: "其他(模板工程)",
color0: "rgba(13,138,212,1)",
color1: "rgba(60,181,251,1)"
},
{ value: 1, name: "卷材缺陷", color0: "yellow", color1: "yellowgreen" },
{ value: 5, name: "混凝土夹渣", color0: "#15E09B", color1: "#1DBD6B" }
];
// 计算总数
var total = data.map(v => v.value).reduce((o, n) => o + n);
// 计算每一个data的其实角度和末了角度 θ1和θ2
data.reduce((o, v) => {
v.theta1 = o;
v.theta2 = o + v.value / total;
return v.theta2;
}, 0);
// 添加渐变
data.forEach((v, i) => {
var ops = calc(v.theta1 * 2 * Math.PI, v.theta2 * 2 * Math.PI);
if (v.value)
v.itemStyle = {
color: {
type: "radial",
x: ops.center[0],
y: ops.center[1],
r: ops.radius,
colorStops: [
{
offset: 0,
color: v.color0
},
{
offset: 0.5,
color: v.color0
},
{
offset: 0.3,
color: v.color1
},
{
offset: 1,
color: v.color1
}
]
// globalCoord: false // 缺省为 false
}
};
v.label = {
normal: {
show: true,
formatter: "{b}\n\n{d}%",
textStyle: {
fontSize: 12,
color: v.color1
},
position: "outside"
},
emphasis: {
show: true
}
};
});
// 计算渐变中心以及渐变半径
function calc(theta1, theta2) {
var r = 0.5; // 半径是0.5 其实表示0.5个直径
var inner = 0.6; // 里面镂空部分占60% option中radius为[33%, 55%] 55 * 0.6 == 33
var cos = Math.cos;
var sin = Math.sin;
var PI = Math.PI;
var min = Math.min;
var max = Math.max;
var bottom = 0;
var left = 2 * r;
var right = 0;
// y0: θ1对应的外部点的y值
// y1: θ2对应的外部点的y值
// _y0: θ1对应的内部点的y值
// _y1: θ2对应的内部点的y值
// x0: θ1对应的外部点的x值
// x1: θ2对应的外部点的x值
// _x0: θ1对应的内部点的x值
// _x1: θ2对应的内部点的x值
var y0 = r * (1 - cos(theta1));
var y1 = r * (1 - cos(theta2));
var _y0 = r * (1 - inner * cos(theta1));
var _y1 = r * (1 - inner * cos(theta2));
// 如果这个弧经过θ == PI的点 则bottom = 2PI
// bottom用于之后的max计算中
if (theta1 < PI && theta2 > PI) {
bottom = 2 * r;
}
// 计算这几个点的最大最小值
var ymin = min(_y0, _y1, y0, y1);
var ymax = max(_y0, _y1, y0, y1, bottom);
var x0 = r * (1 + sin(theta1));
var x1 = r * (1 + sin(theta2));
var _x0 = r * (1 + inner * sin(theta1));
var _x1 = r * (1 + inner * sin(theta2));
// 如果这个弧经过θ == PI / 2的点 则right = 2PI
if (theta1 < PI / 2 && theta2 > PI / 2) {
right = 2 * r;
}
// 如果这个弧经过θ == PI / 2 * 3的点 则left = 0
if (theta1 < (PI / 2) * 3 && theta2 > (PI / 2) * 3) {
left = 0;
}
var xmin = min(_x0, _x1, x0, x1, left);
var xmax = max(_x0, _x1, x1, x0, right);
return {
// 计算圆心以及半径
center: [(r - xmin) / (xmax - xmin), (r - ymin) / (ymax - ymin)],
radius: r / min(xmax - xmin, ymax - ymin)
};
}
var option = {
tooltip: {
trigger: "item",
formatter: "{a} <br/>{b} : {c} ({d}%)"
},
legend: {
orient: "vertical",
x: "75%",
y: "25%",
itemWidth: 14,
itemHeight: 14,
align: "left",
data: [
"混凝土露筋",
"混凝土胀模",
"其他",
"其他(模板工程)",
"卷材缺陷",
"混凝土夹渣"
],
textStyle: {
color: "#fff"
}
},
series: [
{
name: "问题类型",
type: "pie",
radius: ["45%", "70%"],
center: ["40%", "50%"],
data: data,
itemStyle: {
emphasis: {
shadowBlur: 10,
shadowOffsetX: 0,
shadowColor: "rgba(255, 255, 255,1)"
}
}
// animation: false
}
]
};
myChart.setOption(option);
window.addEventListener("resize", () => {
myChart.resize();
});
},
线性渐变
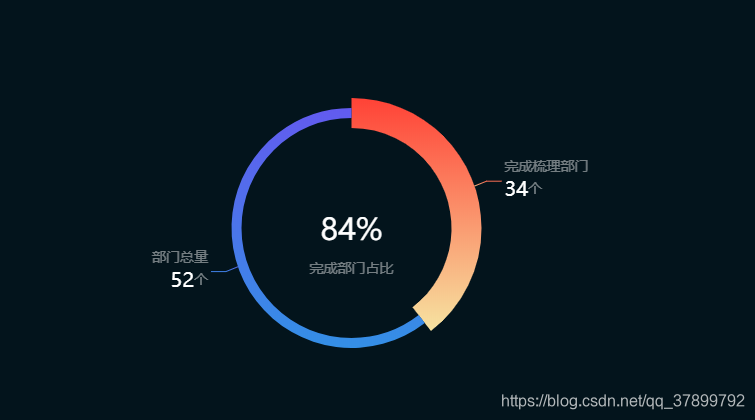
option = {
backgroundColor: "#03141c",
title: {
text: "84%",
subtext: '完成部门占比',
x: 'center',
y: 'center',
textStyle: {
color: "#fff",
fontSize: 30,
fontWeight: 'normal'
},
subtextStyle: {
color: "rgba(255,255,255,.45)",
fontSize: 14,
fontWeight: 'normal'
}
},
tooltip: {
trigger: 'item',
formatter: "{a} <br/>{b} : {c} ({d}%)"
},
legend: {
x: 'center',
y: 'bottom',
data: ['rose3', 'rose5', 'rose6', 'rose7', 'rose8']
},
calculable: true,
series: [
{
name: '面积模式',
type: 'pie',
radius: [100, 130],
center: ['50%', '50%'],
data: [{
value: 34,
name: '吴际帅\n牛亚莉',
itemStyle: {
color: new echarts.graphic.LinearGradient(0, 1, 0, 0, [{
offset: 0,
color: '#f6e3a1'
}, {
offset: 1,
color: '#ff4236'
}])
},
label: {
color: "rgba(255,255,255,.45)",
fontSize: 14,
formatter: '完成梳理部门\n{a|34}个',
rich: {
a: {
color: "#fff",
fontSize: 20,
lineHeight: 30
},
}
}
},
{
value: 52,
name: 'rose2',
itemStyle: {
color: "transparent"
}
}
]
},
{
name: '面积模式',
type: 'pie',
radius: [110, 120],
center: ['50%', '50%'],
data: [{
value: 34,
name: '吴际帅\n牛亚莉',
itemStyle: {
color: "transparent"
}
},
{
value: 52,
name: 'rose2',
itemStyle: {
color: new echarts.graphic.LinearGradient(0, 1, 0, 0, [{
offset: 0,
color: '#348fe6'
}, {
offset: 1,
color: '#625bef'
}])
},
label: {
color: "rgba(255,255,255,.45)",
fontSize: 14,
formatter: '部门总量\n{a|52}个',
rich: {
a: {
color: "#fff",
fontSize: 20,
lineHeight: 30
},
}
}
}
]
}
]
};