Padding组件
class HomeContent extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Padding(
padding: EdgeInsets.fromLTRB(0, 0, 10, 0),
child: GridView.count(
crossAxisCount: 2,
childAspectRatio: 1.5,
children: <Widget>[
Padding(
padding: EdgeInsets.fromLTRB(10, 10, 0, 0),
child: Image.network('https://profile.csdnimg.cn/B/0/A/1_qq_39424143',
fit: BoxFit.cover),
),
Padding(
padding: EdgeInsets.fromLTRB(10, 10, 0, 0),
child: Image.network('https://profile.csdnimg.cn/B/0/A/1_qq_39424143',
fit: BoxFit.cover),
),
Padding(
padding: EdgeInsets.fromLTRB(10, 10, 0, 0),
child: Image.network('https://profile.csdnimg.cn/B/0/A/1_qq_39424143',
fit: BoxFit.cover),
),
Padding(
padding: EdgeInsets.fromLTRB(10, 10, 0, 0),
child: Image.network('https://profile.csdnimg.cn/B/0/A/1_qq_39424143',
fit: BoxFit.cover),
),
Padding(
padding: EdgeInsets.fromLTRB(10, 10, 0, 0),
child: Image.network('https://profile.csdnimg.cn/B/0/A/1_qq_39424143',
fit: BoxFit.cover),
),
Padding(
padding: EdgeInsets.fromLTRB(10, 10, 0, 0),
child: Image.network('https://profile.csdnimg.cn/B/0/A/1_qq_39424143',
fit: BoxFit.cover),
),
],
),
);
}
}
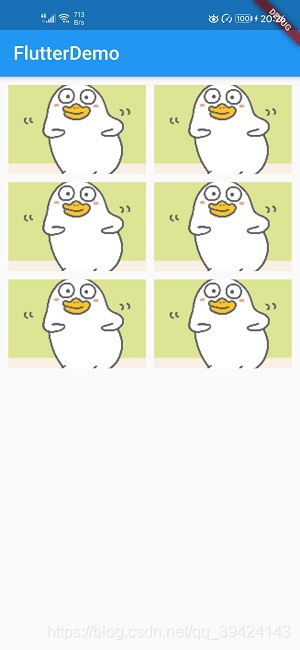
Row水平布局组件
class HomeContent extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(
height: 700,
width: 500,
color: Colors.black26,
child: Row(
crossAxisAlignment: CrossAxisAlignment.center, // 垂直方向排列未居中
mainAxisAlignment: MainAxisAlignment.spaceEvenly, // 类似于weight, 在这里使水平方向均匀分布
children: <Widget>[
IconContainer(Icons.home, color: Colors.red),
IconContainer(Icons.search, color: Colors.blue),
IconContainer(Icons.send, color: Colors.orange),
],
),
);
}
}
class IconContainer extends StatelessWidget {
double size;
IconData icon;
Color color;
IconContainer(this.icon, {this.size, this.color = Colors.blue}) {
this.size = 32.0;
}
@override
Widget build(BuildContext context) {
return Container(
width: this.size + 60,
height: this.size + 60,
color: this.color,
child: Center(
child: Icon(this.icon, color: Colors.white, size: this.size)));
}
}
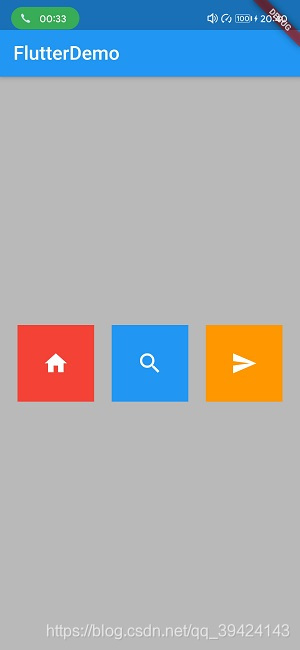
Column垂直布局
class HomeContent extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(
height: 700,
width: 500,
color: Colors.black26,
child: Column(
crossAxisAlignment: CrossAxisAlignment.center, // 水平方向排列未居中
mainAxisAlignment: MainAxisAlignment.spaceEvenly, // 类似于weight, 在这里使水平方向均匀分布
children: <Widget>[
IconContainer(Icons.home, color: Colors.red),
IconContainer(Icons.search, color: Colors.blue),
IconContainer(Icons.send, color: Colors.orange),
],
),
);
}
}
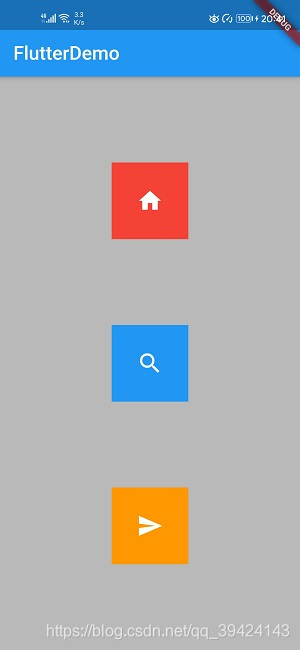
Expand
属性 |
释义 |
flex |
元素占父布局的比例,类似于weight |
@override
Widget build(BuildContext context) {
return Row(
children: <Widget>[
Expanded(
flex: 1,
child: IconContainer(Icons.search,color: Colors.blue)
),
Expanded(
flex: 2,
child: IconContainer(Icons.home,color: Colors.orange),
),
Expanded(
flex: 1,
child: IconContainer(Icons.select_all,color: Colors.red),
),
],
);
}
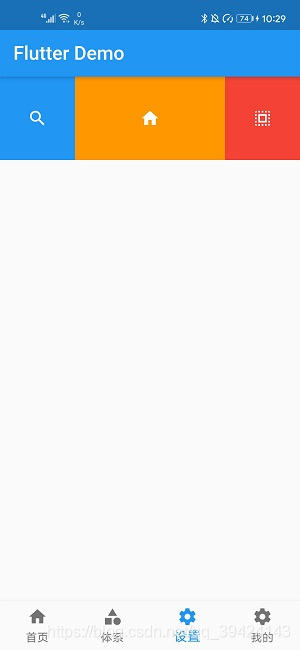