容器组件(Container)
一个组件它往往包含了一些常见的painting, positioning和sizing这样的小部件。
Container相当于我们常用的div,在Flutter中用的非常多,通过一个Container组件可以实现同时需要装饰、变换、限制的场景。下面是Container的定义:
Container({
this.alignment,
this.padding, //容器内补白,属于decoration的装饰范围
Color color, // 背景色
Decoration decoration, // 背景装饰
Decoration foregroundDecoration, //前景装饰
double width,//容器的宽度
double height, //容器的高度
BoxConstraints constraints, //容器大小的限制条件
this.margin,//容器外补白,不属于decoration的装饰范围
this.transform, //变换
this.child,
})
容器的大小可以通过width、height属性来指定,也可以通过constraints来指定;如果它们同时存在时,width、height优先。实际上Container内部会根据width、height来生成一个constraints。
color和decoration是互斥的,如果同时设置它们则会报错!实际上,当指定color时,Container内会自动创建一个decoration。
Container 组件常用属性见下表:
1、alignment
这个属性是针对容器中的child的对其方式。我们先做一个效果:建立一个Container容器,然后让容器里面的文字内容居中对齐。具体代码如下:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: new CenterDemoPage() ,
);
}
}
class CenterDemoPage extends StatefulWidget {
@override
createState() =>new CenterDemoPageState();
}
class CenterDemoPageState extends State<CenterDemoPage> {
@override
Widget build(BuildContext context){
return new Scaffold(
appBar: new AppBar(
title: new Text('Container Widget Demo'),
),
body: _buildDemo(),
);
}
Widget _buildDemo() {
return new Center(
child: Container(
child: new Text('Hello world',style: TextStyle(fontSize: 48.0)),
alignment: Alignment.center,
),
);
}
}
这时候我们可以看见,文本居中显示在我们手机屏幕上了,出来居中对其这种方式,还有以下几种对齐方式可供选择:
topCenter:顶部居中对齐
topLeft:顶部左对齐
topRight:顶部右对齐
center:水平垂直居中对齐
centerLeft:垂直居中水平居左对齐
centerRight:垂直居中水平居右对齐
bottomCenter底部居中对齐
bottomLeft:底部居左对齐
bottomRight:底部居右对齐
2、decoration
容器的修饰器,用于背景或者border。
如果在decoration中用了color,就把容器中设置的color去掉,不要重复设置color,设置的边框样式是让四条边框的宽度为8px,颜色为黑色
child: Container(
child: new Text('Hello world',style: TextStyle(fontSize: 48.0)),
alignment: Alignment.center,
width: 300,
height: 300,
decoration: BoxDecoration(
color: Colors.redAccent,
border: Border.all(
color: Colors.black,
width: 8.0,
),
),
),
decoration里面还可以渐变色:如下
decoration: BoxDecoration(
gradient: LinearGradient(
begin: Alignment.topLeft,
end: Alignment(0.8, 0.0), // 10% of the width, so there are ten blinds.
colors: [const Color(0xFFFFFFEE), const Color(0xFF999999)], // whitish to gray
tileMode: TileMode.repeated, // repeats the gradient over the canvas
),
),
3、margin
margin属性是表示Container与外部其他组件的距离。
当然如果你不想设置每个外边距都一样,想分别设置的话,可以使用如下:
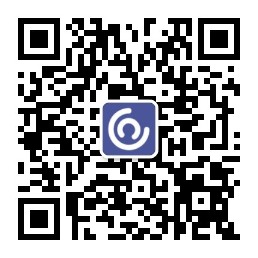
margin: EdgeInsets.fromLTRB(left, top, right, bottom),
4、padding
padding就是Container的内边距,指Container边缘与Child之间的距离。先试试让每个内边距都为20
padding: EdgeInsets.all(20.0),
如果不想让每个内边距一样,依据自己的需求来设置,可以使用如下方法:和margin的使用几乎一样。
padding: EdgeInsets.fromLTRB(left, top, right, bottom),
示例:
...
Container(
margin: EdgeInsets.all(20.0), //容器外补白
color: Colors.orange,
child: Text("Hello world!"),
),
Container(
padding: EdgeInsets.all(20.0), //容器内补白
color: Colors.orange,
child: Text("Hello world!"),
),
...
运行效果如图:
可以发现,直观的感觉就是margin的留白是在容器外部,而padding的留白是在容器内部,要记住这个差异。事实上,Container内margin和padding都是通过Padding 组件来实现的,上面的示例代码实际上等价于:
...
Padding(
padding: EdgeInsets.all(20.0),
child: DecoratedBox(
decoration: BoxDecoration(color: Colors.orange),
child: Text("Hello world!"),
),
),
DecoratedBox(
decoration: BoxDecoration(color: Colors.orange),
child: Padding(
padding: const EdgeInsets.all(20.0),
child: Text("Hello world!"),
),
),
...