参考 一篇文章带你搞懂 SpringBoot与Swagger整合 和 在 Spring Boot 项目中使用 Swagger 文档
1.引入依赖
<!-- https://mvnrepository.com/artifact/io.springfox/springfox-swagger2 -->
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
2.配置Swagger
基本配置:新建Swagger配置类
配置文档信息配置:Swagger 支持设置一些文档的版本号,联系人邮箱,网站,版权,开源协议等等信息。这些信息不是通过注解配置,而是通过创建一个ApiInfo对象,对使用Docket.appInfo()方法来设置。
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.builders.ApiInfoBuilder;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo())
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.any()).build();
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("project 实例文档")
.description("project 实例文档 1.0")
.termsOfServiceUrl("https:github")
.version("1.0")
.build();
}
}
3.如果Springboot项目中配置了拦截器,需要在拦截器配置放行swagger 。具体配置如下
参考Springboot引入拦截器并放行swagger代码实例 和 将拦截器添加到springmvc配置中,并放行swagger的相关资源
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Autowired
private TokenHelper tokenHelper;
@Override
public void addInterceptors(InterceptorRegistry registry) {
List<String> excludePath = new ArrayList<>();
// 获取token 不要验证
excludePath.add("/api/generateToken");
//放行 swagger
excludePath.add("/swagger-resources/**");
excludePath.add("/webjars/**");
excludePath.add("/v2/**");
excludePath.add("/swagger-ui.html/**");
registry.addInterceptor(new TokenInterceptor(tokenHelper)).addPathPatterns("/**")
.excludePathPatterns(excludePath);
}
}
4.看效果:重新启动一下项目,然后在浏览器中访问 http://localhost:8080/swagger-ui.html 就可以看到如下的效果了:
5.Swagger 注解具体使用
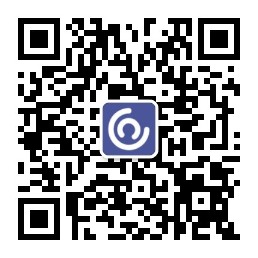
5.1 通过在控制器类上增加@Api
注解,可以给控制器增加描述和标签信息。
5.2 通过在接口方法上增加 @ApiOperation
注解来展开对接口的描述
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
@RestController
@RequestMapping("/api")
@Api(tags = "about test")
public class TestController {
@GetMapping(value = "/test")
@ApiOperation(value = "test function")
public void test() {
//to do
}
}
5.3 接口过滤
有些时候我们并不是希望所有的 Rest API 都呈现在文档上,这种情况下 Swagger2 提供给我们了两种方式配置,一种是基于 @ApiIgnore
注解,另一种是在 Docket 上增加筛选。这里只介绍 @ApiIgnore
注解方式。如果想在文档中屏蔽掉如下接口(/api/logdebug),那么只需要在删除用户的方法上加上 @ApiIgnore 即可。
@GetMapping(path = "/api/logdebug")
@ApiIgnore
public void logdebug(@RequestParam String aa,@RequestParam String bb){
logger.debug("====================this is /api/logdebug ====aa=="+aa);
logger.debug("====================this is /api/logdebug ====bb=="+bb);
}
5.4 实体描述,我们可以通过 @ApiModel
和 @ApiModelProperty
注解来对我们 API 中所涉及到的对象做描述
未写完,待补充……