MailService
package mail.service;
import java.util.List;
import mail.dao.DaoFactory;
import mail.dao.MailDao;
import mail.dao.UserDao;
import mail.domain.Mail;
import mail.domain.User;
public class MailService {
private UserDao userDao = DaoFactory.getUserDao();
private MailDao mailDao = DaoFactory.getMailDao();
public User login(User form) throws UserException {
/*
* 使用form中的username进行查询
*/
User user = userDao.findByUsername(form.getUsername());
/*
* 如果返回null说明用户名不存在,抛出异常,异常信息为"用户名不存在"
*/
if (user == null) {
throw new UserException("用户名不存在");
}
/*
* 比较user的password和form的password,如果不同抛出异常
*/
if (!form.getPassword().equals(user.getPassword())) {
throw new UserException("密码错误");
}
return user;
}
public List<Mail> findByName(String username){
List<Mail> mail = mailDao.findByName(username);
return mail;
}
public Mail findById(int id){
Mail mail = mailDao.findById(id);
return mail;
}
public void addMail(Mail mail) {
mailDao.addMail(mail);
}
public void deleteById(int id) {
mailDao.deleteById(i
MailServlet
package mail.web.servlet;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.List;
import javax.mail.Session;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.junit.Test;
import mail.domain.Mail;
import mail.domain.User;
import mail.service.MailService;
import mail.service.UserException;
import cn.itcast.servlet.BaseServlet;
public class MailServlet extends BaseServlet {
public String login(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
User form = new User();
String username = request.getParameter("username");
String password = request.getParameter("password");
String email = request.getParameter("email");
form.setUsername(username);
form.setPassword(password);
form.setEmail(email);
try {
MailService us = new MailService();
User user = us.login(form);
request.getSession().setAttribute("userName", user.getUsername());
return "r:/MailServlet?method=findByName";
} catch (UserException e) {
request.setAttribute("msg", e.getMessage());
request.setAttribute("user", form);
return "f:/Login/login.jsp";
}
}
public String findByName(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
MailService us = new MailService();
List<Mail> mails = us.findByName((String)request.getSession().getAttribute("userName"));
request.setAttribute("mails", mails);
return "f:/Mail/show.jsp";
}
public String findById(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
MailService us = new MailService();
String param = request.getQueryString();
Mail findMail = us.findById(Integer.parseInt(param.split("&")[1]
.split("=")[1]));
request.getSession().setAttribute("fjr", findMail.getSendto());
request.getSession().setAttribute("bt", findMail.getTitle());
request.getSession().setAttribute("zw", findMail.getMsgcontent());
return "f:/Mail/showMail.jsp";
}
public String addMail(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
MailService us = new MailService();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");//设置日期格式
Mail mail = new Mail(request.getParameter("sjr"), request.getParameter("bt"), request.getParameter("zw"),
1, (String) request.getSession().getAttribute("userName"), df.format(new Date()));
us.addMail(mail);
return "f:/MailServlet?method=findByName";
}
public String deleteById(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String param = (String) request.getSession().getAttribute("ID");
MailService us = new MailService();
us.deleteById(Integer.valueOf(param.split("&")[1].split("=")[1]));
return "f:/MailServlet?method=findByName";
}
@Test
public void fun() {
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");// 设置日期格式
df.format(new Date());
}
JdbcMailDaoImp1
package mail.dao;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import org.junit.Test;
import mail.domain.Mail;
import com.mysql.jdbc.Connection;
public class JdbcMailDaoImpl implements MailDao {
public List<Mail> findByName(String username) {
List<Mail> Mails = new ArrayList<Mail>();
Connection con = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
// 得到数据库的连接
con = JdbcUtils.getConnercion();
// 定义sql语句 得到pstmt
String sql = "SELECT * FROM msg WHERE username=?";
pstmt = con.prepareStatement(sql);
pstmt.setString(1, username);
// 执行sql语句
rs = pstmt.executeQuery();
// 把rs转换成mail类
while (rs.next()) {
// 转换成mail类
Mail mail = new Mail();
mail.setMsgid(rs.getInt("msgid"));
mail.setUsername(rs.getString("username"));
mail.setTitle(rs.getString("title"));
mail.setMsgcontent(rs.getString("msgcontent"));
mail.setState(rs.getInt("state"));
mail.setSendto(rs.getString("sendto"));
mail.setMsg_create_date(rs.getString("msg_create_date"));
Mails.add(mail);
}
return Mails;
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
try {
if (pstmt != null)
pstmt.close();
if (con != null)
con.close();
} catch (SQLException e) {
}
}
}
public Mail findById(int id) {
Connection con = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
// 得到数据库的连接
con = JdbcUtils.getConnercion();
// 定义sql语句 得到pstmt
String sql = "SELECT * FROM msg WHERE msgid=?";
pstmt = con.prepareStatement(sql);
// 给sql语句中的问好赋值
pstmt.setInt(1, id);
// 执行sql语句
rs = pstmt.executeQuery();
if (rs == null)
return null;
if (rs.next()) {
Mail mail = new Mail();
mail.setMsgid(rs.getInt("msgid"));
mail.setUsername(rs.getString("username"));
mail.setTitle(rs.getString("title"));
mail.setMsgcontent(rs.getString("msgcontent"));
mail.setState(rs.getInt("state"));
mail.setSendto(rs.getString("sendto"));
mail.setMsg_create_date(rs.getString("msg_create_date"));
return mail;
} else {
return null;
}
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
try {
if (pstmt != null)
pstmt.close();
if (con != null)
con.close();
} catch (SQLException e) {
}
}
}
public void addMail(Mail mail) {
Connection con = null;
PreparedStatement pstmt = null;
try {
// 得到数据库的连接
con = JdbcUtils.getConnercion();
// 定义sql语句 得到pstmt
// insert into
// msg(username,title,msgcontent,state,sendto,msg_create_date)
String sql = "INSERT INTO msg(username,title,msgcontent,state,sendto,msg_create_date) VALUES(?,?,?,?,?,?)";
pstmt = con.prepareStatement(sql);
// 给sql语句中的问好赋值
pstmt.setString(1, mail.getUsername());
pstmt.setString(2, mail.getTitle());
pstmt.setString(3, mail.getMsgcontent());
pstmt.setInt(4, mail.getState());
pstmt.setString(5, mail.getSendto());
pstmt.setString(6, mail.getMsg_create_date());
// 执行sql语句
pstmt.executeUpdate();
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
// 关闭(倒关)
try {
if (pstmt != null)
pstmt.close();
if (con != null)
con.close();
} catch (SQLException e) {
}
}
}
public void deleteById(int id) {
Connection con = null;
PreparedStatement pstmt = null;
try {
// 得到数据库的连接
con = JdbcUtils.getConnercion();
// 定义sql语句 得到pstmt
// DELETE FROM 表名 [WHERE 条件
String sql = "DELETE FROM msg WHERE msgid=?";
pstmt = con.prepareStatement(sql);
// 给sql语句中的问好赋值
pstmt.setInt(1, id);
// 执行sql语句
pstmt.executeUpdate();
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
// 关闭(倒关)
try {
if (pstmt != null)
pstmt.close();
if (con != null)
con.close();
} catch (SQLException e) {
}
}
}
}
JdbcUserDaoImp1.java
package mail.dao;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import com.mysql.jdbc.Connection;
import mail.domain.User;
public class JdbcUserDaoImpl implements UserDao {
@Override
public User findByUsername(String username) {
Connection con = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
// 得到数据库的连接
con = JdbcUtils.getConnercion();
// 定义sql语句 得到pstmt
String sql = "SELECT * FROM m_user WHERE username=?";
pstmt = con.prepareStatement(sql);
// 给sql语句中的问好赋值
pstmt.setString(1, username);
// 执行sql语句
rs = pstmt.executeQuery();
// 把rs转换成user类
if (rs == null)
return null;
if (rs.next()) {
// 转换成user类
User mail = new User();
mail.setUsername(rs.getString("username"));
mail.setPassword(rs.getString("password"));
mail.setEmail(rs.getString("email"));
return mail;
} else {
return null;
}
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
try {
if (pstmt != null)
pstmt.close();
if (con != null)
con.close();
} catch (SQLException e) {
}
}
}
}
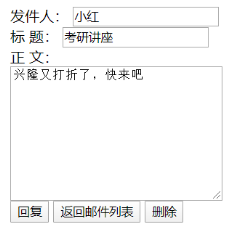