3、设计四个类,分别是:
(1)Shape表示图形类,有面积属性area、周长属性per,颜色属性color,有两个构造方法(一个是默认的、一个是为颜色赋值的),还有3个抽象方法,分别是:getArea计算面积、getPer计算周长、showAll输出所有信息,还有一个求颜色的方法getColor。
1 public abstract class Shape { 2 double area; 3 double per; 4 char color; 5 Shape(){ 6 7 } 8 Shape(char color){ 9 this.color = color; 10 } 11 12 public abstract double getArea(); 13 public abstract double getPer(); 14 public abstract void showAll(); 15 16 public char getColor(){ 17 return color; 18 } 19 }
(2)2个子类:
1)Rectangle表示矩形类,增加两个属性,Width表示长度、height表示宽度,重写getPer、getArea和showAll三个方法,另外又增加一个构造方法(一个是默认的、一个是为高度、宽度、颜色赋值的)。
public class Rectangle extends Shape { double width; double height; Rectangle(){ } Rectangle(double width, double height,char color){ super(color); this.width = width; this.height = height; } @Override public double getArea() { area = width*height; return area; } @Override public double getPer() { per = 2*(width+height); return per; } @Override public void showAll() { System.out.println("长:"+width); System.out.println("宽:"+height); System.out.println("面积:"+getArea()); System.out.println("周长:"+getPer()); System.out.println("颜色:"+getColor()); } }
2)Circle表示圆类,增加1个属性,radius表示半径,重写getPer、getArea和showAll三个方法,另外又增加两个构造方法(为半径、颜色赋值的)。
public class Circle extends Shape { final double pi = 3.14; double radius; Circle(){ } Circle(double radius,char color){ super(color); this.radius = radius; } @Override public double getArea() { area = pi*radius*radius; return area; } @Override public double getPer() { per = 2*pi*radius; return per; } @Override public void showAll() { System.out.println("半径:"+radius); System.out.println("面积:"+getArea()); System.out.println("周长:"+getPer()); System.out.println("颜色:"+getColor()); } }
(3)一个测试类PolyDemo,在main方法中,声明创建每个子类的对象,并调用2个子类的showAll方法。
public class PolyDemo { public static void main(String[] args) { Rectangle r = new Rectangle(1,2,'蓝'); Circle c = new Circle(1.2,'红'); r.showAll(); System.out.println("-----------------"); c.showAll(); System.out.println("-----------------"); } }
4、Cola公司的雇员分为以下若干类:
(1) ColaEmployee :这是所有员工总的父类,属性:员工的姓名,员工的生日月份。
(2) SalariedEmployee : ColaEmployee 的子类,拿固定工资的员工。
(3) HourlyEmployee :ColaEmployee 的子类,按小时拿工资的员工,每月工作超出160 小时的部分按照1.5 倍工资发放。
(4) SalesEmployee :ColaEmployee 的子类,销售人员,工资由月销售额和提成率决定。
(5) 定义一个类Company,在该类中写一个方法,调用该方法可以打印出某月某个员工的工资数额,写一个测试类TestCompany,在main方法,把若干各种类型的员工放在一个ColaEmployee 数组里,并单元出数组中每个员工当月的工资。
public abstract class ColaEmployee { String name; int year; int month; int day; ColaEmployee(){ } ColaEmployee(String name,int year,int month,int day){ this.name = name; this.day = day; this.month = month; this.year = year; } abstract double getSalary(int month); } public class SalariedEmployee extends ColaEmployee { double salary; SalariedEmployee(){ } SalariedEmployee(String name,int year,int month,int day,double salary){ super(name, year, month, day); this.salary =salary; } @Override double getSalary(int month) { if(month == this.month){ salary +=100; } return salary; } } public class SalesEmployee extends ColaEmployee{ double monthSalary; double rate; SalesEmployee(){ } public class HourlyEmployee extends ColaEmployee{ double hourSalary; int hour; HourlyEmployee(){ } HourlyEmployee(String name,int year,int month,int day,double hourSalary,int hour){ super(name, year, month, day); this.hourSalary =hourSalary; this.hour = hour; } @Override double getSalary(int month) { double salary = 0; if(hour<=160){ salary = hour * hourSalary; }else{ salary = 160 * hourSalary + (hour - 160 )*hourSalary*1.5; } if(month == this.month){ salary +=100; } return salary; } } SalesEmployee(String name,int year,int month,int day,double monthSalary,double rate){ super(name, year, month, day); this.monthSalary =monthSalary; this.rate = rate; } @Override double getSalary(int month) { double salary=monthSalary * rate; if(month == this.month){ salary +=100; } return salary; } } public class Company { static void getSalary(int month,ColaEmployee c){ System.out.println(month+"月"+c.name+"的工资:"+c.getSalary(month)); } } public class TestCompany { /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub ColaEmployee[] a = new ColaEmployee[3]; a[0]=new SalariedEmployee("大川",1988,2,29,3500); a[1]=new HourlyEmployee("海鸥",1988,2,29,20,200); a[2]=new SalesEmployee("abc",1988,5,10,30000,0.1); for(ColaEmployee c:a){ Company.getSalary(2, c); } } }
5、利用接口实现动态的创建对象:
(1)创建4个类
1苹果
2香蕉
3葡萄
4园丁
(2)在三种水果的构造方法中打印一句话.
以苹果类为例
class apple
{
public apple()
{
System.out.println(“创建了一个苹果类的对象”);
}
}
(3)类图如下:
(4)要求从控制台输入一个字符串,根据字符串的值来判断创建三种水果中哪个类的对象。
运行结果如图:
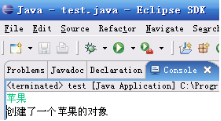
public abstract interface Fruit { } public class Apple implements Fruit{ Apple(){ System.out.println("创建了一个苹果类的对象"); } } public class Banana implements Fruit{ Banana(){ System.out.println("创建了一个香蕉类的对象"); } } public class Putao implements Fruit{ Putao(){ System.out.println("创建了一个葡萄类的对象"); } } public class Gardener { public Fruit creat(){ Fruit f =null; Scanner input =new Scanner(System.in); String name = input.next(); if(name.equals("苹果")){ f = new Apple(); }else if(name.equals("香蕉")){ f = new Banana(); }else if(name.equals("葡萄")){ f =new Putao(); }else{ System.out.println("不会种"); } return f; } }