最近项目中要用到扫描二维码功能,网上找了一圈遇到不少坑,要么是代码太老无法运行,要么是效果不符合。把最终实现分享给大家。
function组件写法
实现效果如下:
代码:
import {RNCamera} from 'react-native-camera';
import React, {useEffect, useRef} from 'react';
import {
StyleSheet,
Animated,
PermissionsAndroid,
default as Easing,
ImageBackground,
View,
Text,
} from 'react-native';
let camera;
const ScanQRCode = () => {
const moveAnim = useRef(new Animated.Value(-2)).current;
useEffect(() => {
requestCameraPermission();
startAnimation();
// eslint-disable-next-line react-hooks/exhaustive-deps
}, []);
//请求权限的方法
const requestCameraPermission = async () => {
try {
const granted = await PermissionsAndroid.request(
PermissionsAndroid.PERMISSIONS.CAMERA,
{
title: '申请摄像头权限',
message: '扫描二维码需要开启相机权限',
buttonNeutral: '等会再问我',
buttonNegative: '不行',
buttonPositive: '好吧',
},
);
if (granted === PermissionsAndroid.RESULTS.GRANTED) {
console.log('现在你获得摄像头权限了');
} else {
console.log('用户没有允许相机权限');
}
} catch (err) {
console.warn(err);
}
};
/** 扫描框动画*/
const startAnimation = () => {
Animated.sequence([
Animated.timing(moveAnim, {
toValue: 200,
duration: 1500,
easing: Easing.linear,
useNativeDriver: false,
}),
Animated.timing(moveAnim, {
toValue: -1,
duration: 1500,
easing: Easing.linear,
useNativeDriver: false,
}),
]).start(() => startAnimation());
};
const onBarCodeRead = (result) => {
const {data} = result; //只要拿到data就可以了
//扫码后的操作
console.log(data);
alert(data);
};
return (
<View style={styles.container}>
<RNCamera
ref={(ref) => {
camera = ref;
}}
autoFocus={RNCamera.Constants.AutoFocus.on} /*自动对焦*/
style={[styles.preview]}
type={RNCamera.Constants.Type.back} /*切换前后摄像头 front前back后*/
flashMode={RNCamera.Constants.FlashMode.off} /*相机闪光模式*/
onBarCodeRead={onBarCodeRead}>
<View
style={{
width: 500,
height: 220,
backgroundColor: 'rgba(0,0,0,0.5)',
}}
/>
<View style={[{flexDirection: 'row'}]}>
<View
style={{
backgroundColor: 'rgba(0,0,0,0.5)',
height: 200,
width: 200,
}}
/>
<ImageBackground
source={require('./assets/qrcode_bg.png')}
style={{width: 200, height: 200}}>
<Animated.View
style={[styles.border, {transform: [{translateY: moveAnim}]}]}
/>
</ImageBackground>
<View
style={{
backgroundColor: 'rgba(0,0,0,0.5)',
height: 200,
width: 200,
}}
/>
</View>
<View
style={{
flex: 1,
backgroundColor: 'rgba(0, 0, 0, 0.5)',
width: 500,
alignItems: 'center',
}}>
<Text style={styles.rectangleText}>
将二维码放入框内,即可自动扫描
</Text>
</View>
</RNCamera>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'row',
},
preview: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
rectangleContainer: {
flex: 1,
justifyContent: 'flex-end',
alignItems: 'center',
},
rectangle: {
height: 200,
width: 200,
borderWidth: 1,
borderColor: '#fcb602',
backgroundColor: 'transparent',
borderRadius: 10,
},
rectangleText: {
flex: 0,
color: '#fff',
marginTop: 10,
},
border: {
flex: 0,
width: 196,
height: 2,
backgroundColor: '#fcb602',
borderRadius: 50,
},
});
export default ScanQRCode;
使用到了react-native-camera组件,具体安装细节就不赘述了,遇到问题可以留言评论。
安装方法请参照官网:
https://react-native-community.github.io/react-native-camera/docs/installation.html
安装步骤:
npm install react-native-camera --save
扫描二维码关注公众号,回复:
11603454 查看本文章
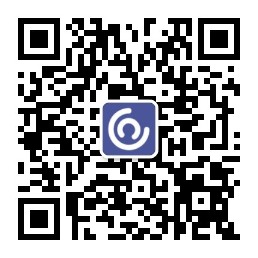
Android - other required steps
Add permissions to your app android/app/src/main/AndroidManifest.xml
file:
<!-- Required -->
<uses-permission android:name="android.permission.CAMERA" />
<!-- Include this only if you are planning to use the camera roll -->
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<!-- Include this only if you are planning to use the microphone for video recording -->
<uses-permission android:name="android.permission.RECORD_AUDIO"/>
Insert the following lines in android/app/build.gradle
:
android {
...
defaultConfig {
...
missingDimensionStrategy 'react-native-camera', 'general' // <--- insert this line
}
}
素材如下(中间是透明的):
参考:
https://blog.csdn.net/qq_38356174/article/details/95360470
如果看我文章还有疑问可以去看下,我的视频实录。