【LeetCode】684. Redundant Connection 冗余连接(Medium)(JAVA)
题目地址: https://leetcode.com/problems/redundant-connection/
题目描述:
In this problem, a tree is an undirected graph that is connected and has no cycles.
The given input is a graph that started as a tree with N nodes (with distinct values 1, 2, …, N), with one additional edge added. The added edge has two different vertices chosen from 1 to N, and was not an edge that already existed.
The resulting graph is given as a 2D-array of edges. Each element of edges is a pair [u, v] with u < v, that represents an undirected edge connecting nodes u and v.
Return an edge that can be removed so that the resulting graph is a tree of N nodes. If there are multiple answers, return the answer that occurs last in the given 2D-array. The answer edge [u, v] should be in the same format, with u < v.
Example 1:
Input: [[1,2], [1,3], [2,3]]
Output: [2,3]
Explanation: The given undirected graph will be like this:
1
/ \
2 - 3
Example 2:
Input: [[1,2], [2,3], [3,4], [1,4], [1,5]]
Output: [1,4]
Explanation: The given undirected graph will be like this:
5 - 1 - 2
| |
4 - 3
Note:
- The size of the input 2D-array will be between 3 and 1000.
- Every integer represented in the 2D-array will be between 1 and N, where N is the size of the input array.
Update (2017-09-26):
We have overhauled the problem description + test cases and specified clearly the graph is an undirected graph. For the directed graph follow up please see Redundant Connection II). We apologize for any inconvenience caused.
题目大意
在本问题中, 树指的是一个连通且无环的无向图。
输入一个图,该图由一个有着N个节点 (节点值不重复1, 2, …, N) 的树及一条附加的边构成。附加的边的两个顶点包含在1到N中间,这条附加的边不属于树中已存在的边。
结果图是一个以边组成的二维数组。每一个边的元素是一对[u, v] ,满足 u < v,表示连接顶点u 和v的无向图的边。
返回一条可以删去的边,使得结果图是一个有着N个节点的树。如果有多个答案,则返回二维数组中最后出现的边。答案边 [u, v] 应满足相同的格式 u < v。
解题方法
- 采用并查集
- 如果两个节点的父节点相同,说明两个已经相连了,直接返回结果;如果两个节点的父节点不同,就把两个节点进行合并
class Solution {
public int[] findRedundantConnection(int[][] edges) {
int[] fa = new int[edges.length + 1];
for (int i = 0; i < fa.length; i++) {
fa[i] = i;
}
for (int i = 0; i < edges.length; i++) {
if (find(fa, edges[i][0]) == find(fa, edges[i][1])) return edges[i];
merge(fa, edges[i][0], edges[i][1]);
}
return new int[]{-1, -1};
}
private int find(int[] fa, int x) {
if (fa[x] != x) return fa[x] = find(fa, fa[x]);
return fa[x];
}
private void merge(int[] fa, int x, int y) {
fa[find(fa, x)] = find(fa, y);
}
}
执行耗时:1 ms,击败了87.99% 的Java用户
内存消耗:38.8 MB,击败了34.46% 的Java用户
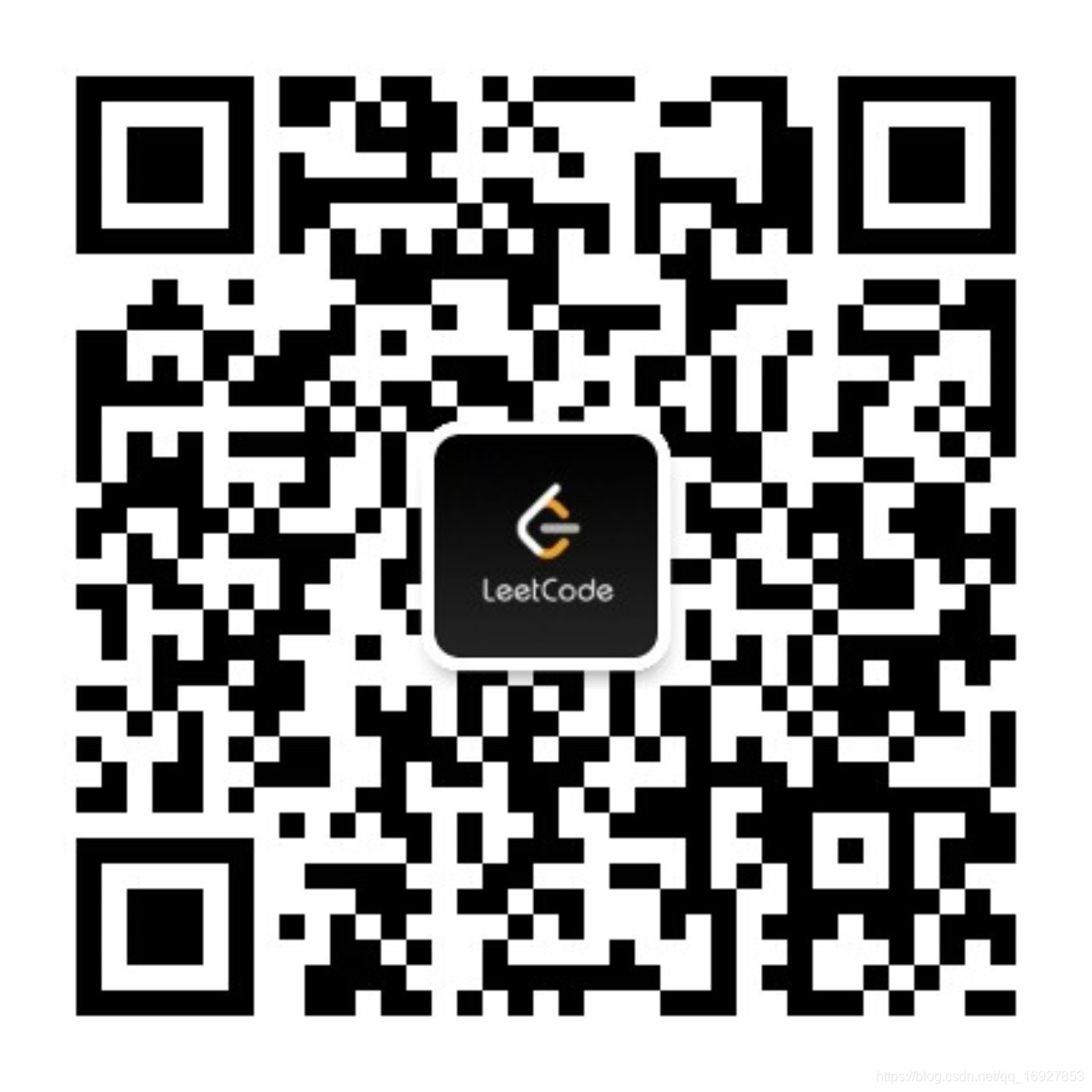