一、安装 vite-plugin-svg-icons
-
npm i vite-plugin-svg-icons -D
-
// 或者
-
yarn add vite-plugin-svg-icons -D
二、在main.js引入
import 'virtual:svg-icons-register'
三、配置SVG图片文件夹
如:
四、在vite.config.js中配置
-
//import path,{ resolve } from 'path'
-
import path
from
'path'
-
import {createSvgIconsPlugin}
from
'vite-plugin-svg-icons'
-
-
export
default
defineConfig(
(command) => {
-
return {
-
plugins: [
-
createSvgIconsPlugin({
-
// 指定要缓存的文件夹
-
iconDirs: [path.
resolve(process.
cwd(),
'src/assets/icons')],
-
// 指定symbolId格式
-
symbolId:
'[name]'
-
})
-
],
-
}
-
-
})
五、新建svg封装组件,路径参考:src\components\svg-icon\index.vue
-
<template>
-
<svg :class="svgClass" aria-hidden="true">
-
<use class="svg-use" :href="symbolId" />
-
</svg>
-
</template>
-
-
<script>
-
import { defineComponent, computed }
from
'vue'
-
-
export
default
defineComponent({
-
name:
'SvgIcon',
-
props: {
-
prefix: {
-
type:
String,
-
default:
'icon'
-
},
-
name: {
-
type:
String,
-
required:
true
-
},
-
className: {
-
type:
String,
-
default:
''
-
}
-
},
-
setup(
props) {
-
const symbolId =
computed(
() =>
`#${props.name}`)
-
const svgClass =
computed(
() => {
-
if (props.
className) {
-
return
`svg-icon ${props.className}`
-
}
-
return
'svg-icon'
-
})
-
return { symbolId, svgClass }
-
}
-
})
-
</script>
-
<style scope>
-
.svg-icon {
-
vertical-align: -
0.1em;
/* 因icon大小被设置为和字体大小一致,而span等标签的下边缘会和字体的基线对齐,故需设置一个往下的偏移比例,来纠正视觉上的未对齐效果 */
-
fill: currentColor;
/* 定义元素的颜色,currentColor是一个变量,这个变量的值就表示当前元素的color值,如果当前元素未设置color值,则从父元素继承 */
-
overflow: hidden;
-
}
-
</style>
六、按需引入使用
-
<template>
-
-
<SvgIcon name="issue">
</SvgIcon>
-
-
</template>
-
-
<script setup>
-
import
SvgIcon
from
"@/components/SvgIcon.vue";
-
</script>
七、全局引入使用
在main.js中加入
-
import svgIcon
from
'./components/svgIcon/index.vue'
-
-
createApp(
App).
component(
'svg-icon', svgIcon)
扫描二维码关注公众号,回复:
15080937 查看本文章
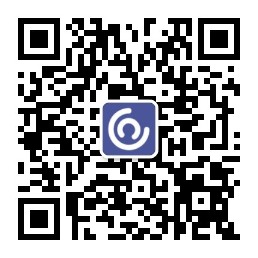
一、安装 vite-plugin-svg-icons
-
npm i vite-plugin-svg-icons -D
-
// 或者
-
yarn add vite-plugin-svg-icons -D
二、在main.js引入
import 'virtual:svg-icons-register'
三、配置SVG图片文件夹
如:
四、在vite.config.js中配置
-
//import path,{ resolve } from 'path'
-
import path
from
'path'
-
import {createSvgIconsPlugin}
from
'vite-plugin-svg-icons'
-
-
export
default
defineConfig(
(command) => {
-
return {
-
plugins: [
-
createSvgIconsPlugin({
-
// 指定要缓存的文件夹
-
iconDirs: [path.
resolve(process.
cwd(),
'src/assets/icons')],
-
// 指定symbolId格式
-
symbolId:
'[name]'
-
})
-
],
-
}
-
-
})
五、新建svg封装组件,路径参考:src\components\svg-icon\index.vue
-
<template>
-
<svg :class="svgClass" aria-hidden="true">
-
<use class="svg-use" :href="symbolId" />
-
</svg>
-
</template>
-
-
<script>
-
import { defineComponent, computed }
from
'vue'
-
-
export
default
defineComponent({
-
name:
'SvgIcon',
-
props: {
-
prefix: {
-
type:
String,
-
default:
'icon'
-
},
-
name: {
-
type:
String,
-
required:
true
-
},
-
className: {
-
type:
String,
-
default:
''
-
}
-
},
-
setup(
props) {
-
const symbolId =
computed(
() =>
`#${props.name}`)
-
const svgClass =
computed(
() => {
-
if (props.
className) {
-
return
`svg-icon ${props.className}`
-
}
-
return
'svg-icon'
-
})
-
return { symbolId, svgClass }
-
}
-
})
-
</script>
-
<style scope>
-
.svg-icon {
-
vertical-align: -
0.1em;
/* 因icon大小被设置为和字体大小一致,而span等标签的下边缘会和字体的基线对齐,故需设置一个往下的偏移比例,来纠正视觉上的未对齐效果 */
-
fill: currentColor;
/* 定义元素的颜色,currentColor是一个变量,这个变量的值就表示当前元素的color值,如果当前元素未设置color值,则从父元素继承 */
-
overflow: hidden;
-
}
-
</style>
六、按需引入使用
-
<template>
-
-
<SvgIcon name="issue">
</SvgIcon>
-
-
</template>
-
-
<script setup>
-
import
SvgIcon
from
"@/components/SvgIcon.vue";
-
</script>
七、全局引入使用
在main.js中加入
-
import svgIcon
from
'./components/svgIcon/index.vue'
-
-
createApp(
App).
component(
'svg-icon', svgIcon)