第三章:使用 Vue 脚手架
3.1 初识脚手架
3.1.1 说明
1. Vue 脚手架是 Vue 官方提供的标准化开发工具(开发平台)
2. 最新版本 5.x (尚硅谷教程里的是 4.x,另外现在官网 Vue CLI 处于维护模式)
3. 文档:https://cli.vuejs.org/zh/
3.1.2 具体步骤
- 第一步:全局安装 @vue/cli(仅第一次执行)
npm install -g @vue/cli
- 第二步:切换到你要创建项目的目录,然后使用命令创建项目
vue create xxxx // xxx 是项目的名称,注意尽可能回避主流库的名字
执行完后,会出现代码执行选择 Vue 的版本:分别是 Vue 2 和 Vue 3,以及自定义选择
项目创建好会出现的界面是:
- 第三步:启动项目
npm run serve
【注】
■ 在进行全局安装之前,必须要先配置 npm 淘宝镜像:
npm config set registry https://registry.npm.taobao.org/
如果没配置好,会出现下载缓慢甚至中断的情况 !
■ 若全局安装过程出现以下情况,请以管理员身份运行 cmd (博主当时安装时就一直出现这个问题,后面以管理员身份运行 cmd ,才成功进行了全局安装)
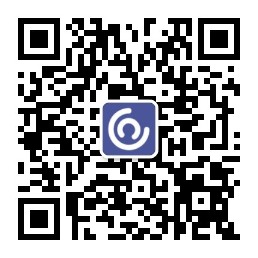
■ Vue 脚手架隐藏了所有 webpack 相关的配置,若想查看具体的 webpack 配置,请执行:
vue inspect > output.js
(上面的代码在 VSCode 终端上执行,其作用是把 Vue 脚手架默认的配置整理成 .js 文件)
■ 暂停项目的快捷键:Ctrl + C
3.1.3 分析脚手架(项目)的结构
脚手架文件结构
├── node_modules
├── public
│ ├── favicon.ico: 页签图标
│ └── index.html: 主页面
├── src
│ ├── assets: 存放静态资源
│ │ └── logo.png
│ │── component: 存放组件
│ │ └── HelloWorld.vue
│ │── App.vue: 汇总所有组件
│ │── main.js: 入口文件
├── .gitignore: git版本管制忽略的配置
├── babel.config.js: babel的配置文件
├── package.json: 应用包配置文件
├── README.md: 应用描述文件
├── package-lock.json:包版本控制文件
- src/components/School.vue
<template>
<div class="demo">
<h2>学校名称:{
{ name }}</h2>
<h2>学校地址:{
{ address }}</h2>
<button @click="showName">点我提示学校名</button>
</div>
</template>
<script>
export default {
name: "School",
data() {
return {
name: "尚硅谷",
address: "北京昌平",
}
},
methods: {
showName() {
alert(this.name)
},
},
}
</script>
<style>
.demo {
background-color: orange;
}
</style>
- src/components/Student.vue
<template>
<div>
<h2>学生姓名:{
{ name }}</h2>
<h2>学生年龄:{
{ age }}</h2>
</div>
</template>
<script>
export default {
name: "Student",
data() {
return {
name: "张三",
age: 18,
}
},
}
</script>
- src/App.vue
<template>
<div>
<img src="./assets/logo.png" alt="logo" />
<School></School>
<Student></Student>
</div>
</template>
<script>
//引入组件
import School from "./components/School"
import Student from "./components/Student"
export default {
name: "App",
components: {
School,
Student,
},
}
</script>
- src/main.js
/*
该文件是整个项目的入口文件
*/
// 引入vue
import Vue from 'vue'
// 引入APP,它是所有组件的父组件
import App from './App.vue'
// 关闭vue的生产提示
Vue.config.productionTip = false
// 创建vue实例对象---vm
new Vue({
// el: '#app',
// 下面一行代码完成的功能是:将App组件放入容器中
render: h => h(App),
}).$mount('#app') // 等同于在12行代码下面放 el:'#app',
// })
- public/index.html
<!DOCTYPE html>
<html lang="">
<head>
<meta charset="utf-8">
<!-- 针对IE浏览器的一个特殊配置,含义是让IE浏览器以最高的渲染级别渲染页面 -->
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<!-- 开启移动端的理想视口 -->
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<!-- 配置页签图标 -->
<link rel="icon" href="<%= BASE_URL %>favicon.ico">
<!-- 配置网页标题 -->
<title>
<%= htmlWebpackPlugin.options.title %>
</title>
</head>
<body>
<!-- 当浏览器不支持js时 ,noscript中的元素就会被渲染 -->
<noscript>
<strong>We're sorry but <%= htmlWebpackPlugin.options.title %> doesn't work properly without JavaScript enabled.
Please enable it to continue.</strong>
</noscript>
<!-- 容器 -->
<div id="app"></div>
<!-- built files will be auto injected -->
</body>
</html>
效果:
3.1.4 render 函数
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
new Vue({
// render函数完成了这个功能:将App组件放入容器中
// 简写形式
render: h => h(App),
}).$mount('#app') // 相当于(el:'#app')
// 完整形式
// render(createElement){
// return createElement(App)
// }
/* --------------------------------------------*/
/* new Vue({
el:'#app'
// render函数完成了这个功能:将App组件放入容器中
// 简写形式
render: h => h(App),
})
// 完整形式
// render(createElement){
// return createElement(App)
// }
*/
总结:
关于不同版本的 Vue
1. vue.js 与 vue.runtime.xxx.js 的区别:
(1). vue.js 是完整版的 Vue,包含:核心功能+模板解析器。
(2). vue.runtime.xxx.js 是运行版的 Vue,只包含:核心功能;没有模板解析器。
2. 因为 vue.runtime.xxx.js 没有模板解析器,所以不能使用 template 配置项,需要使用 render 函数接收到的 createElement 函数去指定具体内容。
3.1.5 修改默认配置
vue.config.js
是一个可选的配置文件,如果项目的 (和package.json
同级的) 根目录中存在这个文件,那么它会被@vue/cli-service
自动加载。也可以使用package.json
中的vue
字段,但是注意这种写法需要你严格遵照 JSON 的格式。- 使用 vue inspect > output.js 可以查看到 vue 脚手架的默认配置。
- 使用 vue.config.js 可以对脚手架进行个性化定制,详情见:配置参考 | Vue CLI。
vue.config.js:↓ ↓ ↓
module.exports ={ // →CommonJS 是 node.js 默认的模块化方案
pages: {
index: {
// page 的入口
entry: 'src/main.js',
},
/* 当使用只有入口的字符串格式时,
模板会被推导为 `public/subpage.html`
并且如果找不到的话,就回退到 `public/index.html`。
输出文件名会被推导为 `subpage.html`。
subpage: 'src/subpage/main.js'
*/
},
lintOnSave: false // 关闭语法检查
}
【注】若修改了 vue.config.js ,终端必须重新 npm run serve !!!
3.2 ref 属性
- src/main.js
// 引入 vue
import Vue from 'vue'
// 引入App
import App from './App.vue'
// 关闭Vue的生产提示
Vue.config.productionTip = false
//创建vm
new Vue({
el: '#app',
render: h => h(App)
})
- src/components/School.vue
<template>
<div class="school">
<h2>学校名称:{
{name}}</h2>
<h2>学校地址:{
{address}}</h2>
</div>
</template>
<script>
export default {
name: 'School',
data() {
return {
name: '尚硅谷',
address:'北京昌平'
}
}
}
</script>
<style>
.school{
background-color: gray;
}
</style>
- src/App.vue
<template>
<div>
<h1 v-text="msg" ref="title"></h1>
<button ref="btn" @click="showDOM">点我输出上方的DOM元素</button>
<School ref="sch"/>
</div>
</template>
<script>
// 引入School组件
import School from './components/School'
export default {
name: 'App',
components: { School },
data() {
return {
msg:'欢迎学习Vue!'
}
},
methods: {
showDOM() {
console.log(this.$refs.title) // 真实DOM元素
console.log(this.$refs.btn) // 真实DOM元素
console.log(this.$refs.sch) // School组件的实例对象
}
}
}
</script>
效果:
总结:
ref 属性
1. 被用来给元素或子组件注册引用信息(id 的替代者)
2. 应用在 html 标签上获取的是真实 DOM 元素,应用在组件标签上是组件实例对象(vc)
3. 使用方式:
♦ 打标识:<h1 ref = "xxx">……</h1> 或 <School ref = "xxx"></School>
♦ 获取:this.$refs.xxx
3.3 props 配置项
- src/components/Student.vue
<template>
<div>
<h1>{
{msg}}</h1>
<h2>学生姓名:{
{name}}</h2>
<h2>学生性别:{
{sex}}</h2>
<h2>学生年龄:{
{myAge+1}}</h2>
<button @click="updateAge">尝试修改收到的年龄</button>
</div>
</template>
<script>
export default {
name:'Student',
data() {
console.log(this);
return {
msg: '我是一个尚硅谷的学生',
myAge:this.age
}
},
methods: {
updateAge() {
this.myAge++
}
},
// 简单声明接收
props:['name','age','sex']
// 接收的同时对数据进行类型限制
/* props: {
name: String,
age: Number,
sex:String
}
*/
// 接收的同时对数据进行类型限制+默认值的指定+必要性的限制(最完整的写法)
// props: {
// name: {
// type: String, //name 的类型是字符串
// required:true // name 是必要的
// },
// age: {
// type: Number,
// default:99 // 默认值
// },
// sex: {
// type: String, //sex 的类型是字符串
// required:true
// }
// }
}
</script>
- src/App.vue
<template>
<div>
<Student name= "李四" sex= "女" :age="18" />
</div>
</template>
<script>
import Student from './components/Student'
export default {
name: 'App',
components: { Student }
}
</script>
效果:
总结:
props 配置项:
1. 功能:让组件接收外部传过来的数据
2. 传递数据:<Demo name = "xxx"/>
3. 接收数据:
◑ 第一种方式(只接收):props:['name']
◑ 第二种方式(限制类型):props:{name:String}
◑ 第三种方式(限制类型、限制必要性、指定默认值):
props:{
name:{
type:String, //类型
required:true, //必要性
default:'老王' //默认值
}
}
【备注】props 是只读的,Vue 底层会监测你对 props 的修改,如果进行了修改,就会发出警告,若业务需求确实需要修改,那么请复制 props 的内容到 data 中一份,然后去修改 data 中的数据。
3.4 mixin 混入
局部混入
- src/mixin.js
export const hunhe = {
methods: {
showName() {
alert(this.name)
}
},
mounted() {
console.log('你好啊!')
},
}
- src/main.js
// 引入 vue
import Vue from 'vue'
// 引入App
import App from './App.vue'
// 关闭Vue的生产提示
Vue.config.productionTip = false
//创建vm
new Vue({
el: '#app',
render: h => h(App)
})
- src/components/School.vue
<template>
<div>
<h2 @click="showName">学校名称:{
{name}}</h2>
<h2>学校地址:{
{address}}</h2>
</div>
</template>
<script>
// 引入一个hunhe
import { hunhe} from '../mixin'
export default {
name:'School',
data() {
return {
name: '尚硅谷',
address:'北京'
}
},
mixins:[hunhe]
}
</script>
-
src/components/Student.vue
<template>
<div>
<h2 @click="showName">学生姓名:{
{name}}</h2>
<h2>学生性别:{
{sex}}</h2>
</div>
</template>
<script>
// 引入一个hunhe
import { hunhe} from '../mixin'
export default {
name:'Student',
data() {
return {
name: '张三',
sex:'男'
}
},
mixins:[hunhe]
}
</script>
- src/App.vue
<template>
<div>
<School/>
<hr>
<Student/>
</div>
</template>
<script>
import School from './components/School'
import Student from './components/Student'
export default {
name: 'App',
components: { School,Student }
}
</script>
效果:
全局混入
除 App.vue 和 mixin.js 不变,其他稍作修改:
- src/main.js
// 引入 vue
import Vue from 'vue'
// 引入App
import App from './App.vue'
import {hunhe} from './mixin'
// 关闭Vue的生产提示
Vue.config.productionTip = false
Vue.mixin(hunhe) // 所有的 vm 和 vc 都会得到 hunhe
//创建vm
new Vue({
el: '#app',
render: h => h(App)
})
- src/components/School.vue
<template>
<div>
<h2 @click="showName">学校名称:{
{ name }}</h2>
<h2>学校地址:{
{ address }}</h2>
</div>
</template>
<script>
// import { hunhe} from '../mixin' //不需要从这里引入了
export default {
name: "School",
data() {
return {
name: "尚硅谷",
address: "北京",
}
},
}
</script>
- src/components/Student.vue
<template>
<div>
<h2 @click="showName">学生姓名:{
{ name }}</h2>
<h2>学生性别:{
{ sex }}</h2>
</div>
</template>
<script>
// import { hunhe} from '../mixin' // 不需要从这里引入了
export default {
name: "Student",
data() {
return {
name: "张三",
sex: "男",
}
},
}
</script>
效果:
总结:
mixin (混入)
1. 功能:可以把多个组件共用的配置提取成一个混入对象
2. 使用方式:
○ 第一步定义混合:
{
data(){....},
methods:{....}
....
}
○ 第二步使用混入:
▶ 全局混入:Vue.mixin(xxx)
▶ 局部混入:mixins:['xxx']
3. 备注:
○ 组件和混入对象存在同名选项冲突时,以组件为主
○ 同名生命周期钩子将合并为一个数组,不以谁为主,都将被调用。
- src/mixin.js
export const hunhe = {
data() {
return {
x:100,
y:200
}
},
methods: {
showName() {
alert(this.name)
}
},
mounted() {
console.log('你好啊!')
console.log(this.$data);
},
}
- src/components/School.vue
<template>
<div>
<h2 @click="showName">学校名称:{
{ name }}</h2>
<h2>学校地址:{
{ address }}</h2>
</div>
</template>
<script>
// 引入一个hunhe
import { hunhe} from '../mixin'
export default {
name: "School",
data() {
return {
name: "尚硅谷",
address: "北京",
x: 666,
}
},
mixins: [hunhe],
mounted() {
console.log('你好啊!!!')
}
}
</script>
效果:
3.5 插件
- src/main.js
// 引入 vue
import Vue from 'vue'
// 引入App
import App from './App.vue'
// 引入插件
import plugins from "./plugins"
// 关闭Vue的生产提示
Vue.config.productionTip = false
//应用(使用)插件
Vue.use(plugins)
//创建vm
new Vue({
el: '#app',
render: h => h(App)
})
- src/plugin.js
export default {
install(Vue) {
//全局过滤器
Vue.filter('mySlice', function (value) {
return value.slice(0, 4)
})
//定义全局指令
Vue.directive('fbind', {
//指令与元素成功绑定时(一上来)
bind(element, binding) {
element.value = binding.value
},
//指令所在元素被插入页面时
inserted(element, binding) {
element.focus()
},
//指令所在的模板被重新解析时
update(element, binding) {
element.value = binding.value
}
})
//定义混入
Vue.mixin({
data() {
return {
x: 100,
y: 200
}
},
})
//给Vue原型上添加一个方法(vm和vc就都能用了)
Vue.prototype.hello = () => { alert('你好啊') }
}
}
- src/App.vue
<template>
<div>
<School/>
<hr>
<Student/>
</div>
</template>
<script>
import School from './components/School'
import Student from './components/Student'
export default {
name: 'App',
components: { School,Student }
}
</script>
- src/components/School.vue
<template>
<div>
<h2>学校名称:{
{name | mySlice}}</h2>
<h2>学校地址:{
{address}}</h2>
<input type="text" v-fbind:value="address">
<button @click="test">点我测试一个hello方法</button>
</div>
</template>
<script>
// 引入一个hunhe
export default {
name:'School',
data() {
return {
name: '尚硅谷atguigu',
address:'北京'
}
},
methods: {
test() {
this.hello()
}
}
}
</script>
- src/components/Student.vue
<template>
<div>
<h2>学生姓名:{
{name}}</h2>
<h2>学生性别:{
{sex}}</h2>
<input type="text" v-fbind:value="name">
</div>
</template>
<script>
export default {
name:'Student',
data() {
return {
name: '张三',
sex:'男'
}
},
}
</script>
效果:
总结:
1. 功能:用于增强 Vue
2. 本质:包含 install() 方法的一个对象,install 的第一个参数是 Vue,第二个以后的参数是插件使用者传递的数据。
3. 一个插件可以是一个拥有 install()
方法的对象,也可以直接是一个安装函数本身
4. 定义插件:
对象.install = function (Vue, options) {
// 1. 添加全局过滤器
Vue.filter(....)
// 2. 添加全局指令
Vue.directive(....)
// 3. 配置全局混入(合)
Vue.mixin(....)
// 4. 添加实例方法
Vue.prototype.$myMethod = function () {...}
Vue.prototype.$myProperty = xxxx
}
4. 使用插件:Vue.use()
3.6 scoped 样式
src/components/School.vue
<template>
<div class="demo">
<h2>学校名称:{
{name}}</h2>
<h2>学校地址:{
{address}}</h2>
</div>
</template>
<script>
export default {
name:'School',
data() {
return {
name: '尚硅谷atguigu',
address:'北京'
}
},
}
</script>
<style scoped>
.demo{
background-color: skyblue;
}
</style>
- src/components/Student.vue
<template>
<div class="demo">
<h2 class="title">学生姓名:{
{name}}</h2>
<h2 class="atguigu">学生性别:{
{sex}}</h2>
</div>
</template>
<script>
export default {
name:'Student',
data() {
return {
name: '张三',
sex:'男'
}
},
}
</script>
<style scoped lang="less">
.demo{
background-color:pink;
.atguigu{
font-size: 40px;
}
}
</style>
- src/App.vue
<template>
<div>
<h1 class="title">你好啊</h1>
<School/>
<hr>
<Student/>
</div>
</template>
<script>
import School from './components/School'
import Student from './components/Student'
export default {
name: 'App',
components: { School,Student }
}
</script>
<style scoped>
.title{
color: red;
}
</style>
效果:
总结:
scoped 样式
1. 作用:让样式在局部生效,防止冲突。
2. 写法:<style scoped>
3.7 Todo-list 案例
- src/components/MyHeader.vue
<template>
<div class="todo-header">
<input type="text" placeholder="请输入你的任务名称,按回车键确认" v-model="title" @keyup.enter="add"/>
</div>
</template>
<script>
import { nanoid } from "nanoid";
export default {
name: 'MyHeader',
props: ['addTodo'],
data() {
return {
title:''
}
},
methods: {
add() {
// 校验数据
if(!this.title.trim()) return alert('输入不能为空')
// 将用户的输入包装成一个todo对象
const todoObj = { id: nanoid(), title: this.title, done: false }
// 通知App组件去添加一个todo对象
this.addTodo(todoObj)
//清空输入
this.title=''
}
}
}
</script>
<style scoped>
/*header*/
.todo-header input {
width: 560px;
height: 28px;
font-size: 14px;
border: 1px solid #ccc;
border-radius: 4px;
padding: 4px 7px;
}
.todo-header input:focus {
outline: none;
border-color: rgba(82, 168, 236, 0.8);
box-shadow: inset 0 1px 1px rgba(0, 0, 0, 0.075), 0 0 8px rgba(82, 168, 236, 0.6);
}
</style>
- src/components/MyItem.vue
<template>
<li>
<label>
<input type="checkbox" :checked="todo.done" @change="handleCheck(todo.id)"/>
<span>{
{todo.title}}</span>
</label>
<button class="btn btn-danger" @click="HandleDelete(todo.id)">删除</button>
</li>
</template>
<script>
export default {
name: 'MyItem',
// 声明接收todo 对象
props: ['todo','checkTodo','deleteTodo'],
methods: {
//勾选或取消勾选
handleCheck(id) {
// 通知App组件将对应的todo值取反
this.checkTodo(id)
},
//删除
HandleDelete(id) {
if (confirm('确定删除吗?')) {
this.deleteTodo(id)
}
}
}
}
</script>
<style scoped>
/*item*/
li {
list-style: none;
height: 36px;
line-height: 36px;
padding: 0 5px;
border-bottom: 1px solid #ddd;
}
li label {
float: left;
cursor: pointer;
}
li label li input {
vertical-align: middle;
margin-right: 6px;
position: relative;
top: -1px;
}
li button {
float: right;
display: none;
margin-top: 3px;
}
li:before {
content: initial;
}
li:last-child {
border-bottom: none;
}
li:hover{
background-color: #ddd;
}
li:hover button{
display: block;
}
</style>
- src/components/MyList.vue
<template>
<ul class="todo-main">
<MyItem
v-for="todoObj in todos"
:key="todoObj.id"
:todo="todoObj"
:checkTodo="checkTodo"
:deleteTodo="deleteTodo"
/>
</ul>
</template>
<script>
import MyItem from './MyItem'
export default {
name: 'MyList',
components: { MyItem },
props:['todos','checkTodo','deleteTodo'],
}
</script>
<style scoped>
/*main*/
.todo-main {
margin-left: 0px;
border: 1px solid #ddd;
border-radius: 2px;
padding: 0px;
}
.todo-empty {
height: 40px;
line-height: 40px;
border: 1px solid #ddd;
border-radius: 2px;
padding-left: 5px;
margin-top: 10px;
}
</style>
- src/components/MyFooter.vue
<template>
<div class="todo-footer" v-show="total">
<label>
<input type="checkbox" v-model="isAll"/>
</label>
<span>
<span>已完成{
{doneTotal}}</span> / 全部{
{total}}
</span>
<button class="btn btn-danger" @click="clearAll">清除已完成任务</button>
</div>
</template>
<script>
export default {
name: 'MyFooter',
props: ['todos','checkAllTodo','clearAllTodo'],
computed: {
total() {
return this.todos.length
},
doneTotal() {
return this.todos.reduce((pre,todo)=>pre+(todo.done?1:0),0)
},
isAll:{
get(){
return this.doneTotal === this.total && this.total > 0
},
set(value) {
this.checkAllTodo(value)
}
}
},
methods: {
clearAll() {
this.clearAllTodo()
}
}
}
</script>
<style scoped>
/*footer*/
.todo-footer {
height: 40px;
line-height: 40px;
padding-left: 6px;
margin-top: 5px;
}
.todo-footer label {
display: inline-block;
margin-right: 20px;
cursor: pointer;
}
.todo-footer label input {
position: relative;
top: -1px;
vertical-align: middle;
margin-right: 5px;
}
.todo-footer button {
float: right;
margin-top: 5px;
}
</style>
- src/App.vue
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<MyHeader :addTodo="addTodo"/>
<MyList :todos="todos" :checkTodo="checkTodo" :deleteTodo="deleteTodo"/>
<MyFooter :todos="todos" :checkAllTodo="checkAllTodo" :clearAllTodo="clearAllTodo"/>
</div>
</div>
</div>
</template>
<script>
import MyHeader from './components/MyHeader'
import MyList from './components/MyList'
import MyFooter from './components/MyFooter'
export default {
name: 'App',
components: { MyHeader, MyList, MyFooter },
data(){
return{
todos: [
{id:'001',title:'抽烟',done:true},
{id:'002',title:'喝酒',done:false},
{id:'003',title:'开车',done:true}
]
}
},
methods: {
//添加一个todo
addTodo(todoObj) {
this.todos.unshift(todoObj)
},
//勾选or取消勾选一个todo
checkTodo(id) {
this.todos.forEach((todo) => {
if(todo.id===id) todo.done=!todo.done
})
},
//删除一个todo
deleteTodo(id) {
this.todos=this.todos.filter(todo=>todo.id !==id)
},
//全选或者取消全选
checkAllTodo(done) {
this.todos.forEach((todo) => {
todo.done=done
})
},
//清除所有已经完成的todo
clearAllTodo() {
this.todos = this.todos.filter((todo) => {
return !todo.done
})
}
}
}
</script>
<style >
/*base*/
body {
background: #fff;
}
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2), 0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
.btn:focus {
outline: none;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
效果:
总结:
1. 组件化编码流程:
● 拆分静态组件:组件要按照功能点拆分,命名不要与 html 元素冲突。
● 实现动态组件:考虑好数据的存放位置,数据是一个组件在用,还是一些组件在用:
○ 一个组件在用:放在组件自身即可。
○ 一些组件在用:放在他们共同的父组件上(状态提升 )
2. props 适用于:
● 父组件 ==> 子组件 通信
● 组件 ==> 父组件 通信(要求父先给子一个函数)
3. 使用 v-model 时要切记:v-model 绑定的值不能是 props 传过来的值,因为 props 是不可以修改的!
4. props 传过来的若是对象类型的值,修改对象中的属性时 Vue 不会报错,但不推荐这样做。
3.8 浏览器本地存储
- localStorage
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>localStorage</title>
</head>
<body>
<h2>localStorage</h2>
<button onclick="saveData()">点我保存一个数据</button>
<button onclick="readData()">点我读取一个数据</button>
<button onclick="deleteData()">点我删除一个数据</button>
<button onclick="deleteAllData()">点我清空一个数据</button>
<script type="text/javascript">
let p = { name: '张三', age: 18 }
function saveData() {
localStorage.setItem('msg', 'hello!!!')
localStorage.setItem('msg2', 666)
localStorage.setItem('person', JSON.stringify(p))
}
function readData() {
console.log(localStorage.getItem('msg'))
console.log(localStorage.getItem('msg2'))
const result = localStorage.getItem('person')
console.log(JSON.parse(result))
// console.log(localStorage.getItem('msg3'))
}
function deleteData() {
localStorage.removeItem('msg2')
}
function deleteAllData() {
localStorage.clear()
}
</script>
</body>
</html>
- sessionStorage
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>sessionStorage</title>
</head>
<body>
<h2>sessionStorage</h2>
<button onclick="saveData()">点我保存一个数据</button>
<button onclick="readData()">点我读取一个数据</button>
<button onclick="deleteData()">点我删除一个数据</button>
<button onclick="deleteAllData()">点我清空一个数据</button>
<script type="text/javascript">
let p = { name: '张三', age: 18 }
function saveData() {
sessionStorage.setItem('msg', 'hello!!!')
sessionStorage.setItem('msg2', 666)
sessionStorage.setItem('person', JSON.stringify(p))
}
function readData() {
console.log(sessionStorage.getItem('msg'))
console.log(sessionStorage.getItem('msg2'))
const result = sessionStorage.getItem('person')
console.log(JSON.parse(result))
// console.log(sessionStorage.getItem('msg3'))
}
function deleteData() {
sessionStorage.removeItem('msg2')
}
function deleteAllData() {
sessionStorage.clear()
}
</script>
</body>
</html>
效果:
总结:
webStorage
1. 存储内容大小一般支持5MB左右(不同浏览器可能还不一样)
2. 浏览器端通过 Window.sessionStorage 和 Window.localStorage 属性来实现本地存储机制。
3. 相关 API:
● xxxxxStorage.setItem('key', 'value'):该方法接受一个键和值作为参数,会把键值对添加到存储中,如果键名存在,则更新其对应的值。
● xxxxxStorage.getItem('person'):该方法接受一个键名作为参数,返回键名对应的值。
● xxxxxStorage.removeItem('key'): 该方法接受一个键名作为参数,并把该键名从存储中删除。
● xxxxxStorage.clear():该方法会清空存储中的所有数据。
4. 备注:
① SessionStorage 存储的内容会随着浏览器窗口关闭而消失。
② LocalStorage 存储的内容,需要手动清除才会消失。
③ xxxxxStorage.getItem(xxx) 如果 xxx 对应的 value 获取不到,那么 getItem 的返回值是 null。
④ JSON.parse(null) 的结果依然是 null。
利用本地存储优化 Todolist 案例
修改 App.vue 文件 :
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<MyHeader :addTodo="addTodo"/>
<MyList :todos="todos" :checkTodo="checkTodo" :deleteTodo="deleteTodo"/>
<MyFooter :todos="todos" :checkAllTodo="checkAllTodo" :clearAllTodo="clearAllTodo"/>
</div>
</div>
</div>
</template>
<script>
import MyHeader from './components/MyHeader'
import MyList from './components/MyList'
import MyFooter from './components/MyFooter'
export default {
name: 'App',
components: { MyHeader, MyList, MyFooter },
data(){
return{
// 由于 todos 是 MyHeader 组件和 MyFooter 组件都在使用,所以放在 App 中(状态提升)
todos: JSON.parse(localStorage.getItem('todos')) || []
}
},
methods: {
//添加一个todo
addTodo(todoObj) {
this.todos.unshift(todoObj)
},
//勾选or取消勾选一个todo
checkTodo(id) {
this.todos.forEach((todo) => {
if(todo.id===id) todo.done=!todo.done
})
},
//删除一个todo
deleteTodo(id) {
this.todos=this.todos.filter(todo=>todo.id !==id)
},
//全选或者取消全选
checkAllTodo(done) {
this.todos.forEach((todo) => {
todo.done=done
})
},
//清除所有已经完成的todo
clearAllTodo() {
this.todos = this.todos.filter((todo) => {
return !todo.done
})
}
},
watch: {
todos: {
deep:true,
handler(value) {
localStorage.setItem('todos', JSON.stringify(value))
}
}
}
}
</script>
<style >
/*base*/
body {
background: #fff;
}
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2), 0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
.btn:focus {
outline: none;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
3.9 Vue 组件中的自定义事件
3.9.1 绑定
- src/App.vue
<template>
<div class="app">
<h1>{
{ msg }}</h1>
<!-- 通过父组件给子组件传递函数类型的props实现:子给父传递数据 -->
<School :getSchoolName="getSchoolName" />
<!-- 通过父组件给子组件绑定一个自定义事件实现:子给父传递数据 (第一种写法),使用@或者v-on -->
<!-- 第二种写法注意要把下面 mounted()里的内容删掉-->
<!--<Student v-on:atguigu="getStudentName"/> -->
<!--<Student @atguigu="getStudentName" @demo="m1" />-->
<!-- 通过父组件给子组件绑定一个自定义事件实现:子给父传递数据 (第二种写法),使用ref -->
<Student ref="student"/>
</div>
</template>
<script>
import School from "./components/School"
import Student from "./components/Student"
export default {
name: "App",
components: { School, Student },
data() {
return {
msg: "你好啊",
}
},
methods: {
getSchoolName(name) {
console.log("App收到了学校名:", name)
},
getStudentName(name) {
console.log("App收到了学生姓名:", name)
},
m1() {
console.log("demo事件被触发了")
},
},
mounted() {
this.$refs.student.$on("atguigu", this.getStudentName)
},
}
</script>
<style scoped>
.app {
background-color: gray;
padding: 5px;
}
</style>
- src/components/School.vue
<template>
<div class="school">
<h2>学校名称:{
{name}}</h2>
<h2>学校地址:{
{address}}</h2>
<button @click="sendSchoolName">把学校名给App</button>
</div>
</template>
<script>
export default {
name: 'School',
props:['getSchoolName'],
data() {
return {
name: '尚硅谷',
address:'北京'
}
},
methods: {
sendSchoolName() {
this.getSchoolName(this.name)
}
}
}
</script>
<style scoped>
.school{
background-color: skyblue;
padding: 5px;
}
</style>
- src/components/Student.vue
<template>
<div class="student">
<h2>学生姓名:{
{ name }}</h2>
<h2>学生性别:{
{ sex }}</h2>
<h2>当前求和为:{
{ number }}</h2>
<button @click="sendStudentName">把学生姓名给App</button>
</div>
</template>
<script>
export default {
name: "Student",
data() {
return {
name: "张三",
sex: "男",
number: 0,
}
},
methods: {
sendStudentName() {
//触发Student组件实例身上的atguigu事件
this.$emit("atguigu", this.name)
},
},
}
</script>
<style scoped lang="less">
.student {
background-color: pink;
padding: 5px;
margin-top: 30px;
}
</style>
效果:
3.9.2 解绑
App.vue 和 School.vue 不变,修改 Student.vue
- src/components/Student.vue
<template>
<div class="student">
<h2>学生姓名:{
{ name }}</h2>
<h2>学生性别:{
{ sex }}</h2>
<h2>当前求和为:{
{ number }}</h2>
<!-- <button @click="add">点我number++</button> -->
<button @click="sendStudentName">把学生姓名给App</button>
<button @click="unbind">解绑atguigu事件</button>
<!-- <button @click="death">销毁当前Student组件的实例(vc)</button> -->
</div>
</template>
<script>
export default {
name: "Student",
data() {
return {
name: "张三",
sex: "男",
number: 0,
}
},
methods: {
sendStudentName() {
//触发Student组件实例身上的atguigu事件
this.$emit("atguigu", this.name)
},
unbind() {
//this.$off('atguigu')// 只适用于解绑一个自定义事件
//this.$off(['atguigu','demo'])// 适用于解绑多个个自定义事件
this.$off() // 解绑所有自定义事件(比较暴力)
},
},
}
</script>
<style scoped lang="less">
.student {
background-color: pink;
padding: 5px;
margin-top: 30px;
}
</style>
效果:
【注】-------- 销毁 vc (子组件)实例 和销毁 vm (根组件)的效果
以 Student.vue 为例,先销毁 vc,修改 Student.vue 代码
<template>
<div class="student">
<h2>学生姓名:{
{ name }}</h2>
<h2>学生性别:{
{ sex }}</h2>
<h2>当前求和为:{
{ number }}</h2>
<button @click="add">点我number++</button>
<button @click="sendStudentName">把学生姓名给App</button>
<button @click="unbind">解绑atguigu事件</button>
<button @click="death">销毁当前Student组件的实例(vc)</button>
</div>
</template>
<script>
export default {
name: "Student",
data() {
return {
name: "张三",
sex: "男",
number: 0,
}
},
methods: {
add() {
console.log("add回调被调用了")
this.number++
},
sendStudentName() {
//触发Student组件实例身上的atguigu事件
this.$emit("atguigu", this.name)
},
unbind() {
//this.$off('atguigu')// 只适用于解绑一个自定义事件
//this.$off(['atguigu','demo'])// 适用于解绑多个个自定义事件
this.$off() // 解绑所有自定义事件(比较暴力)
},
death() {
this.$destroy() //销毁了当前Student组件的实例,销毁后所有Student实例的自定义事件全都不奏效。
},
},
}
</script>
<style scoped lang="less">
.student {
background-color: pink;
padding: 5px;
margin-top: 30px;
}
</style>
效果:
这里原生的 DOM 事件(number++)也不能调用了 ,原因是 vue.js 版本的问题
再看看销毁 vm 效果如何:
修改 main.js
// 引入 vue
import Vue from 'vue'
// 引入App
import App from './App.vue'
// 关闭Vue的生产提示
Vue.config.productionTip = false
//创建vm
new Vue({
el: '#app',
render: h => h(App),
mounted() {
setTimeout(() => {
this.$destroy()
},3000)
},
})
效果:
总结:
组件的自定义事件
1. 一种组件间通信的方式,适用于:子组件 ===> 父组件
2. 使用场景:A是父组件,B是子组件,B想给A传数据,那么就要在A中给B绑定自定义事件(事件的回调在 A 中)。
3. 绑定自定义事件:
● 第一种方式,在父组件中:<Demo @atguigu="test"/> 或 <Demo v-on:atguigu="test"/>
● 第二种方式,在父组件中:
<Demo ref="demo"/>
......
mounted(){
this.$refs.xxx.$on('atguigu',this.test)
}
● 若想让自定义事件只能触发一次,可以使用 once 修饰符,或 $once 方法。
4. 触发自定义事件:this.$emit('atguigu',数据)
5. 解绑自定义事件 this.$off('atguigu')
6. 组件上也可以绑定原生 DOM 事件,需要使用 native 修饰符。
7. 注意:通过 this.$refs.xxx.$on('atguigu',回调) 绑定自定义事件时,回调 <span style="color:red">要么配置在 methods 中,要么用箭头函数 </span>,否则 this 指向会出问题!
3.9.3 TodoList 案例___自定义事件
- src/components/MyHeader.vue
<template>
<div class="todo-header">
<input type="text" placeholder="请输入你的任务名称,按回车键确认" v-model="title" @keyup.enter="add"/>
</div>
</template>
<script>
import { nanoid } from "nanoid";
export default {
name: 'MyHeader',
data() {
return {
title:''
}
},
methods: {
add() {
// 校验数据
if(!this.title.trim()) return alert('输入不能为空')
// 将用户的输入包装成一个todo对象
const todoObj = { id: nanoid(), title: this.title, done: false }
// 通知App组件去添加一个todo对象
this.$emit('addTodo',todoObj)
//清空输入
this.title=''
}
}
}
</script>
<style scoped>
/*header*/
.todo-header input {
width: 560px;
height: 28px;
font-size: 14px;
border: 1px solid #ccc;
border-radius: 4px;
padding: 4px 7px;
}
.todo-header input:focus {
outline: none;
border-color: rgba(82, 168, 236, 0.8);
box-shadow: inset 0 1px 1px rgba(0, 0, 0, 0.075), 0 0 8px rgba(82, 168, 236, 0.6);
}
</style>
- src/components/MyFooter
<template>
<div class="todo-footer" v-show="total">
<label>
<!-- <input type="checkbox" :checked="isAll" @change="checkAll"/> -->
<input type="checkbox" v-model="isAll"/>
</label>
<span>
<span>已完成{
{doneTotal}}</span> / 全部{
{total}}
</span>
<button class="btn btn-danger" @click="clearAll">清除已完成任务</button>
</div>
</template>
<script>
export default {
name: 'MyFooter',
props: ['todos'],
computed: {
total() {
return this.todos.length
},
doneTotal() {
return this.todos.reduce((pre,todo)=>pre+(todo.done?1:0),0)
},
isAll:{
get(){
return this.doneTotal === this.total && this.total > 0
},
set(value) {
// this.checkAllTodo(value)
this.$emit('checkAllTodo',value)
}
}
},
methods: {
// checkAll(e) {
// this.checkAllTodo(e.target.checked)
// }
clearAll() {
// this.clearAllTodo()
this.$emit('clearAllTodo')
}
}
}
</script>
<style scoped>
/*footer*/
.todo-footer {
height: 40px;
line-height: 40px;
padding-left: 6px;
margin-top: 5px;
}
.todo-footer label {
display: inline-block;
margin-right: 20px;
cursor: pointer;
}
.todo-footer label input {
position: relative;
top: -1px;
vertical-align: middle;
margin-right: 5px;
}
.todo-footer button {
float: right;
margin-top: 5px;
}
</style>
- src/App.vue
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<MyHeader @addTodo="addTodo"/>
<MyList :todos="todos" :checkTodo="checkTodo" :deleteTodo="deleteTodo"/>
<MyFooter :todos="todos" @checkAllTodo="checkAllTodo" @clearAllTodo="clearAllTodo"/>
</div>
</div>
</div>
</template>
<script>
import MyHeader from './components/MyHeader'
import MyList from './components/MyList'
import MyFooter from './components/MyFooter'
export default {
name: 'App',
components: { MyHeader, MyList, MyFooter },
data(){
return{
todos: JSON.parse(localStorage.getItem('todos')) || []
}
},
methods: {
//添加一个todo
addTodo(todoObj) {
this.todos.unshift(todoObj)
},
//勾选or取消勾选一个todo
checkTodo(id) {
this.todos.forEach((todo) => {
if(todo.id===id) todo.done=!todo.done
})
},
//删除一个todo
deleteTodo(id) {
this.todos=this.todos.filter(todo=>todo.id !==id)
},
//全选或者取消全选
checkAllTodo(done) {
this.todos.forEach((todo) => {
todo.done=done
})
},
//清除所有已经完成的todo
clearAllTodo() {
this.todos = this.todos.filter((todo) => {
return !todo.done
})
}
},
watch: {
todos: {
deep:true,
handler(value) {
localStorage.setItem('todos', JSON.stringify(value))
}
}
}
}
</script>
<style >
/*base*/
body {
background: #fff;
}
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2), 0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
.btn:focus {
outline: none;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
效果:
3.10 全局事件总线(GlobalEventBus)
- src/main.js
// 引入 vue
import Vue from 'vue'
// 引入App
import App from './App.vue'
// 关闭Vue的生产提示
Vue.config.productionTip = false
Vue.prototype.x = { a: 1, b: 2 }
//创建vm
new Vue({
el: '#app',
render: h => h(App),
beforeCreate() {
Vue.prototype.$bus = this //安装全局事件总线
}
})
- src/App.vue
<template>
<div class="app">
<h1>{
{msg}}</h1>
<School/>
<Student/>
</div>
</template>
<script>
import School from './components/School'
import Student from './components/Student'
export default {
name: 'App',
components: { School, Student },
data() {
return {
msg:'你好啊'
}
},
}
</script>
<style scoped>
.app{
background-color:gray;
padding: 5px;
}
</style>
- src/components/School.vue
<template>
<div class="school">
<h2>学校名称:{
{ name }}</h2>
<h2>学校地址:{
{ address }}</h2>
</div>
</template>
<script>
export default {
name: "School",
data() {
return {
name: "尚硅谷",
address: "北京",
}
},
mounted() {
console.log("School", this.x)
this.$bus.$on("hello", (data) => {
console.log("我是School组件,收到了数据", data)
})
},
beforeDestroy() {
this.$bus.$off("hello")
},
}
</script>
<style scoped>
.school {
background-color: skyblue;
padding: 5px;
}
</style>
- src/components/Student.vue
<template>
<div class="student">
<h2>学生姓名:{
{ name }}</h2>
<h2>学生性别:{
{ sex }}</h2>
<button @click="sendStudentName">把学生名School组件</button>
</div>
</template>
<script>
export default {
name: "Student",
data() {
return {
name: "张三",
sex: "男",
}
},
mounted() {
console.log("Student", this.x)
},
methods: {
sendStudentName() {
this.$bus.$emit("hello", this.name)
},
},
}
</script>
<style scoped lang="less">
.student {
background-color: pink;
padding: 5px;
margin-top: 30px;
}
</style>
效果:
总结:
全局事件总线(GlobalEventBus)
1. 一种组件间通信的方式,适用于任意组件间通信 。
2. 安装全局事件总线:
new Vue({
......
beforeCreate() {
Vue.prototype.$bus = this //安装全局事件总线,$bus 就是当前应用的 vm
},
......
})
3. 使用事件总线:
- 接收数据:A组件想接收数据,则在A组件中给 $bus 绑定自定义事件,事件的回调留在A组件自身。
methods(){
demo(data){......}
}
......
mounted() {
this.$bus.$on('xxxx',this.demo)
}
- 提供数据:this.$bus.$emit('xxxx',数据)
4. 最好在 beforeDestroy 钩子中,用 $off 去解绑当前组件所用到的事件。
【注】
● 所有组件实例对象的原型对象的原型对象就是 Vue 的原型对象
◇ 所有组件对象都能看到 Vue 原型对象上的属性和方法。
◇ Vue.prototype.$bus = new Vue(), 所有的组件对象都能看到 $bus 这个属性对象。
● 全局事件总线:
① 包含事件处理相关方法的对象(只有一个)
② 所有的组件都可以得到
TodoList 事件总线
- src/main.js
// 引入 vue
import Vue from 'vue'
// 引入App
import App from './App.vue'
// 关闭Vue的生产提示
Vue.config.productionTip = false
//创建vm
new Vue({
el: '#app',
render: h => h(App),
beforeCreate() {
Vue.prototype.$bus = this // 安装全局事件总线
}
})
- src/App.vue
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<MyHeader @addTodo="addTodo"/>
<MyList :todos="todos"/>
<MyFooter :todos="todos" @checkAllTodo="checkAllTodo" @clearAllTodo="clearAllTodo"/>
</div>
</div>
</div>
</template>
<script>
import MyHeader from './components/MyHeader'
import MyList from './components/MyList'
import MyFooter from './components/MyFooter'
export default {
name: 'App',
components: { MyHeader, MyList, MyFooter },
data(){
return{
todos: JSON.parse(localStorage.getItem('todos')) || []
}
},
methods: {
//添加一个todo
addTodo(todoObj) {
this.todos.unshift(todoObj)
},
//勾选or取消勾选一个todo
checkTodo(id) {
this.todos.forEach((todo) => {
if(todo.id===id) todo.done=!todo.done
})
},
//删除一个todo
deleteTodo(id) {
this.todos=this.todos.filter(todo=>todo.id !==id)
},
//全选或者取消全选
checkAllTodo(done) {
this.todos.forEach((todo) => {
todo.done=done
})
},
//清除所有已经完成的todo
clearAllTodo() {
this.todos = this.todos.filter((todo) => {
return !todo.done
})
}
},
watch: {
todos: {
deep:true,
handler(value) {
localStorage.setItem('todos', JSON.stringify(value))
}
}
},
mounted() {
this.$bus.$on('checkTodo',this.checkTodo)
this.$bus.$on('deleteTodo',this.deleteTodo)
},
beforeDestroy() {
this.$bus.$off('checkTodo')
this.$bus.$off('deleteTodo')
}
}
</script>
<style >
/*base*/
body {
background: #fff;
}
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2), 0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
.btn:focus {
outline: none;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
- src/components/MyList.vue
<template>
<ul class="todo-main">
<MyItem
v-for="todoObj in todos"
:key="todoObj.id"
:todo="todoObj"
/>
</ul>
</template>
<script>
import MyItem from './MyItem'
export default {
name: 'MyList',
components: { MyItem },
// 声明接收App传递过来的数据
props:['todos']
}
</script>
<style scoped>
/*main*/
.todo-main {
margin-left: 0px;
border: 1px solid #ddd;
border-radius: 2px;
padding: 0px;
}
.todo-empty {
height: 40px;
line-height: 40px;
border: 1px solid #ddd;
border-radius: 2px;
padding-left: 5px;
margin-top: 10px;
}
</style>
- src/components/MyItem.vue
<template>
<li>
<label>
<input type="checkbox" :checked="todo.done" @change="handleCheck(todo.id)"/>
<span>{
{todo.title}}</span>
</label>
<button class="btn btn-danger" @click="HandleDelete(todo.id)">删除</button>
</li>
</template>
<script>
export default {
name: 'MyItem',
// 声明接收todo 对象
props: ['todo'],
methods: {
//勾选或取消勾选
handleCheck(id) {
// 通知App组件将对应的todo对象的done值取反
// this.checkTodo(id)
this.$bus.$emit('checkTodo',id)
},
//删除
HandleDelete(id) {
if (confirm('确定删除吗?')) {
// 通知App组件将对应的todo对象删除
// this.deleteTodo(id)
this.$bus.$emit('deleteTodo',id)
}
}
}
}
</script>
<style scoped>
/*item*/
li {
list-style: none;
height: 36px;
line-height: 36px;
padding: 0 5px;
border-bottom: 1px solid #ddd;
}
li label {
float: left;
cursor: pointer;
}
li label li input {
vertical-align: middle;
margin-right: 6px;
position: relative;
top: -1px;
}
li button {
float: right;
display: none;
margin-top: 3px;
}
li:before {
content: initial;
}
li:last-child {
border-bottom: none;
}
li:hover{
background-color: #ddd;
}
li:hover button{
display: block;
}
</style>
3.11 消息订阅与发布
- src/App.vue
<template>
<div class="app">
<h1>{
{msg}}</h1>
<School/>
<Student/>
</div>
</template>
<script>
import School from './components/School'
import Student from './components/Student'
export default {
name: 'App',
components: { School, Student },
data() {
return {
msg:'你好啊'
}
},
}
</script>
<style scoped>
.app{
background-color:gray;
padding: 5px;
}
</style>
- src/components/School.vue
<template>
<div class="school">
<h2>学校名称:{
{ name }}</h2>
<h2>学校地址:{
{ address }}</h2>
</div>
</template>
<script>
import pubsub from "pubsub-js"
export default {
name: "School",
data() {
return {
name: "尚硅谷",
address: "北京",
}
},
mounted() {
console.log("School", this.x)
// this.$bus.$on('hello', (data) => {
// console.log("我是School组件,收到了数据", data)
// })
this.pubId = pubsub.subscribe("hello", (msgName, data) => {
console.log("有人发布了hello消息, hello消息的回调执行了", msgName, data)
})
},
beforeDestroy() {
// this.$bus.$off("hello")
pubsub.unsubscribe(this.pubId)
},
}
</script>
<style scoped>
.school {
background-color: skyblue;
padding: 5px;
}
</style>
- src/components/Student.vue
<template>
<div class="student">
<h2>学生姓名:{
{ name }}</h2>
<h2>学生性别:{
{ sex }}</h2>
<button @click="sendStudentName">把学生名School组件</button>
</div>
</template>
<script>
import pubsub from "pubsub-js"
export default {
name: "Student",
data() {
return {
name: "张三",
sex: "男",
}
},
mounted() {
console.log("Student", this.x)
},
methods: {
sendStudentName() {
// this.$bus.$emit("hello", this.name)
pubsub.publish("hello", 666)
},
},
}
</script>
<style scoped lang="less">
.student {
background-color: pink;
padding: 5px;
margin-top: 30px;
}
</style>
效果:
总结:
消息订阅与发布(pubsub)
1. 一种组件间通信的方式,适用于任意组件间通信。在线文档:https://github.com/mroderick/PubSubJS
2. 使用步骤:
① 安装 pubsub:npm i pubsub-js
② 引入:import pubsub from 'pubsub-js'
③ 接收数据:A组件想接收数据,则在A组件中订阅消息,订阅的回调留在A组件自身。
methods(){
demo(data){......}
}
......
mounted() {
this.pid = pubsub.subscribe('xxx',this.demo) //订阅消息
}
4. 提供数据:pubsub.publish('xxx',数据)
5. 最好在 beforeDestroy 钩子中,用 PubSub.unsubscribe(pid) 去取消订阅。
【注】----【相关语法】
□ import PubSub from 'pubsub-js' // 引入
□ PubSub.subscribe(‘msgName’, functon(msgName, data){})
□ PubSub.publish(‘msgName’, data) // 发布消息, 触发订阅的回调函数调用
□ PubSub.unsubscribe(token) // 取消消息的订阅
利用消息订阅与发布优化 TodoList 案例
- src/App.vue
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<MyHeader @addTodo="addTodo" />
<MyList :todos="todos" />
<MyFooter
:todos="todos"
@checkAllTodo="checkAllTodo"
@clearAllTodo="clearAllTodo"
/>
</div>
</div>
</div>
</template>
<script>
// 引入数据库的规则:第三方往上放,自己写的一般往下拷
import pubsub from "pubsub-js"
import MyHeader from "./components/MyHeader"
import MyList from "./components/MyList"
import MyFooter from "./components/MyFooter"
export default {
name: "App",
components: { MyHeader, MyList, MyFooter },
data() {
return {
todos: JSON.parse(localStorage.getItem("todos")) || [],
}
},
methods: {
//添加一个todo
addTodo(todoObj) {
this.todos.unshift(todoObj)
},
//勾选or取消勾选一个todo
checkTodo(id) {
this.todos.forEach((todo) => {
if (todo.id === id) todo.done = !todo.done
})
},
//删除一个todo
deleteTodo(_, id) {
this.todos = this.todos.filter((todo) => todo.id !== id)
},
//全选或者取消全选
checkAllTodo(done) {
this.todos.forEach((todo) => {
todo.done = done
})
},
//清除所有已经完成的todo
clearAllTodo() {
this.todos = this.todos.filter((todo) => {
return !todo.done
})
},
},
watch: {
todos: {
deep: true,
handler(value) {
localStorage.setItem("todos", JSON.stringify(value))
},
},
},
mounted() {
this.$bus.$on("checkTodo", this.checkTodo)
this.pubId = pubsub.subscribe("deleteTodo", this.deleteTodo)
},
beforeDestroy() {
this.$bus.$off("checkTodo")
pubsub.unsubscribe(this.pubId)
},
}
</script>
<style>
/*base*/
body {
background: #fff;
}
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2),
0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
.btn:focus {
outline: none;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
- src/components/MyItem.vue
<template>
<li>
<label>
<input type="checkbox" :checked="todo.done" @change="handleCheck(todo.id)"/>
<span>{
{todo.title}}</span>
</label>
<button class="btn btn-danger" @click="HandleDelete(todo.id)">删除</button>
</li>
</template>
<script>
import pubsub from 'pubsub-js'
export default {
name: 'MyItem',
// 声明接收todo 对象
props: ['todo'],
methods: {
//勾选或取消勾选
handleCheck(id) {
// 通知App组件将对应的todo对象的done值取反
// this.checkTodo(id)
this.$bus.$emit('checkTodo',id)
},
//删除
HandleDelete(id) {
if (confirm('确定删除吗?')) {
// 通知App组件将对应的todo对象删除
// this.deleteTodo(id)
// this.$bus.$emit('deleteTodo',id)
pubsub.publish('deleteTodo',id)
}
}
}
}
</script>
<style scoped>
/*item*/
li {
list-style: none;
height: 36px;
line-height: 36px;
padding: 0 5px;
border-bottom: 1px solid #ddd;
}
li label {
float: left;
cursor: pointer;
}
li label li input {
vertical-align: middle;
margin-right: 6px;
position: relative;
top: -1px;
}
li button {
float: right;
display: none;
margin-top: 3px;
}
li:before {
content: initial;
}
li:last-child {
border-bottom: none;
}
li:hover{
background-color: #ddd;
}
li:hover button{
display: block;
}
</style>
3.12 $nextTick
$nextTick(回调函数)
可以将回调延迟到下次 DOM 更新循环之后执行
使用 $nextTick 优化 TodoList案例:
- src/App.vue
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<MyHeader @addTodo="addTodo"/>
<MyList :todos="todos"/>
<MyFooter :todos="todos" @checkAllTodo="checkAllTodo" @clearAllTodo="clearAllTodo"/>
</div>
</div>
</div>
</template>
<script>
import pubsub from 'pubsub-js'
import MyHeader from './components/MyHeader'
import MyList from './components/MyList'
import MyFooter from './components/MyFooter'
export default {
name: 'App',
components: { MyHeader, MyList, MyFooter },
data(){
return{
todos: JSON.parse(localStorage.getItem('todos')) || []
}
},
methods: {
//添加一个todo
addTodo(todoObj) {
this.todos.unshift(todoObj)
},
//勾选or取消勾选一个todo
checkTodo(id) {
this.todos.forEach((todo) => {
if(todo.id === id) todo.done = !todo.done
})
},
//更新一个todo
updateTodo(id,title) {
this.todos.forEach((todo) => {
if(todo.id === id) todo.title = title
})
},
//删除一个todo
deleteTodo(_,id) {
this.todos = this.todos.filter(todo => todo.id !==id)
},
//全选或者取消全选
checkAllTodo(done) {
this.todos.forEach((todo) => {
todo.done=done
})
},
//清除所有已经完成的todo
clearAllTodo() {
this.todos = this.todos.filter((todo) => {
return !todo.done
})
}
},
watch: {
todos: {
deep:true,
handler(value) {
localStorage.setItem('todos', JSON.stringify(value))
}
}
},
mounted() {
this.$bus.$on('checkTodo',this.checkTodo)
this.$bus.$on('updateTodo',this.updateTodo)
this.pubId = pubsub.subscribe('deleteTodo',this.deleteTodo)
},
beforeDestroy() {
this.$bus.$off('checkTodo')
this.$bus.$off('updateTodo')
pubsub.unsubscribe(this.pubId)
}
}
</script>
<style>
/*base*/
body {
background: #fff;
}
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2), 0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-edit {
color: #fff;
background-color: skyblue;
border: 1px solid rgb(103, 159, 180);
margin-right: 5px;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
.btn:focus {
outline: none;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
- src/components/MyItem.vue
<template>
<li>
<label>
<input type="checkbox" :checked="todo.done" @change="handleCheck(todo.id)"/>
<span v-show="!todo.isEdit">{
{todo.title}}</span>
<input
type="text"
v-show="todo.isEdit"
:value="todo.title"
@blur="handleBlur(todo,$event)"
ref="inputTitle"
>
</label>
<button class="btn btn-danger" @click="handleDelete(todo.id)">删除</button>
<button v-show="!todo.isEdit" class="btn btn-edit" @click="handleEdit(todo)">编辑</button>
</li>
</template>
<script>
import pubsub from 'pubsub-js'
export default {
name: 'MyItem',
// 声明接收todo 对象
props: ['todo'],
methods: {
//勾选或取消勾选
handleCheck(id) {
// 通知App组件将对应的todo对象的done值取反
// this.checkTodo(id)
this.$bus.$emit('checkTodo',id)
},
//删除
handleDelete(id) {
if (confirm('确定删除吗?')) {
// 通知App组件将对应的todo对象删除
// this.deleteTodo(id)
// this.$bus.$emit('deleteTodo',id)
pubsub.publish('deleteTodo',id)
}
},
//编辑
handleEdit(todo) {
if (todo.hasOwnProperty('isEdit')) {
todo.isEdit = true
} else {
this.$set(todo,'isEdit',true)
}
this.$nextTick(function () {
this.$refs.inputTitle.focus()
})
},
// 失去焦点回调(真正执行修改逻辑)
handleBlur(todo,e) {
todo.isEdit = false
if(!e.target.value.trim()) return alert('输入不能为空!')
this.$bus.$emit('updateTodo',todo.id,e.target.value)
}
}
}
</script>
<style scoped>
/*item*/
li {
list-style: none;
height: 36px;
line-height: 36px;
padding: 0 5px;
border-bottom: 1px solid #ddd;
}
li label {
float: left;
cursor: pointer;
}
li label li input {
vertical-align: middle;
margin-right: 6px;
position: relative;
top: -1px;
}
li button {
float: right;
display: none;
margin-top: 3px;
}
li:before {
content: initial;
}
li:last-child {
border-bottom: none;
}
li:hover{
background-color: #ddd;
}
li:hover button{
display: block;
}
</style>
效果:
总结:
$nextTick :
1. 语法:this.$nextTick(回调函数)
2. 作用:在下一次 DOM 更新结束后执行其指定的回调。
3. 什么时候用:当改变数据后,要基于更新后的新 DOM 进行某些操作时,要在 nextTick 所指定的回调函数中执行。
3.13 Vue封装的过渡与动画
- src/App.vue
<template>
<div>
<Test />
<Test2 />
<Test3 />
</div>
</template>
<script>
import Test from "./components/Test"
import Test2 from "./components/Test2"
import Test3 from "./components/Test3"
export default {
name: "App",
components: { Test, Test2, Test3 },
}
</script>
- src/components/Test.vue
<template>
<div>
<button @click="isShow = !isShow">显示/隐藏</button>
<transition name="hello" appear>
<h1 v-show="isShow">你好啊!</h1>
</transition>
</div>
</template>
<script>
export default {
name: "Test",
data() {
return {
isShow: true,
}
},
}
</script>
<style scoped>
h1 {
background-color: orange;
}
.hello-enter-active {
animation: atguigu 0.5s linear;
}
.hello-leave-active {
animation: atguigu 1s reverse;
}
@keyframes atguigu {
from {
transform: translateX(-100%);
}
to {
transform: translateX(0px);
}
}
</style>
- src/components/Test2.vue
<template>
<div>
<button @click="isShow = !isShow">显示/隐藏</button>
<transition-group name="hello" appear>
<h1 v-show="isShow" key="1">你好啊!</h1>
<h1 v-show="isShow" key="2">尚硅谷!</h1>
</transition-group>
</div>
</template>
<script>
export default {
name: "Test",
data() {
return {
isShow: true,
}
},
}
</script>
<style scoped>
h1 {
background-color: orange;
/* transition: 0.5s linear; */
}
/* 进入的起点 、离开的终点*/
.hello-enter,
.hello-leave-to {
transform: translateX(-100%);
}
.hello-enter-active,
.hello-leave-active {
transition: 0.5s linear; /* 与h1中写这个一样的效果 */
}
/* 进入的终点 、离开的起点*/
.hello-enter-to,
.hello-leave {
transform: translateX(0);
}
</style>
- src/components/Test3.vue
<template>
<div>
<button @click="isShow = !isShow">显示/隐藏</button>
<transition-group
appear
name="animate__animated animate__bounce"
enter-active-class="animate__swing"
leave-active-class="animate__backOutUp"
>
<h1 v-show="isShow" key="1">你好啊!</h1>
<h1 v-show="isShow" key="2">尚硅谷!</h1>
</transition-group>
</div>
</template>
<script>
import "animate.css"
export default {
name: "Test",
data() {
return {
isShow: true,
}
},
}
</script>
<style scoped>
h1 {
background-color: orange;
/* transition: 0.5s linear; */
}
</style>
效果:
总结:
Vue 封装的过渡与动画 :
1. 作用:在插入、更新或移除 DOM 元素时,在合适的时候给元素添加样式类名。
2. 图示:
3. 写法:
● 准备好样式:
▲ 元素进入的样式:
① v-enter:进入的起点
② v-enter-active:进入过程中
③ v-enter-to:进入的终点
▲ 元素离开的样式:
① v-leave:离开的起点
② v-leave-active:离开过程中
③ v-leave-to:离开的终点
● 使用 <transition> 包裹要过渡的元素,并配置 name 属性:
<transition name="hello">
<h1 v-show="isShow">你好啊!</h1>
</transition>
定义 class 样式
▼指定过渡样式: transition
▼指定隐藏时的样式: opacity/其它
● 备注:若有多个元素需要过渡,则需要使用:<transition-group>,且每个元素都要指定 key 值。
TodoList 案例动画效果
- src/components/MyItem.vue
<template>
<!-- 除了在MyItem加transition外,还有另一种方法:在MyList里面修改 -->
<!-- <transition name="todo" appear> -->
<li>
<label>
<input
type="checkbox"
:checked="todo.done"
@change="handleCheck(todo.id)"
/>
<span v-show="!todo.isEdit">{
{ todo.title }}</span>
<input
type="text"
v-show="todo.isEdit"
:value="todo.title"
@blur="handleBlur(todo, $event)"
ref="inputTitle"
/>
</label>
<button class="btn btn-danger" @click="handleDelete(todo.id)">删除</button>
<button
v-show="!todo.isEdit"
class="btn btn-edit"
@click="handleEdit(todo)"
>
编辑
</button>
</li>
<!-- </transition> -->
</template>
<script>
import pubsub from "pubsub-js"
export default {
name: "MyItem",
// 声明接收todo 对象
props: ["todo"],
methods: {
//勾选或取消勾选
handleCheck(id) {
// 通知App组件将对应的todo对象的done值取反
// this.checkTodo(id)
this.$bus.$emit("checkTodo", id)
},
//删除
handleDelete(id) {
if (confirm("确定删除吗?")) {
// 通知App组件将对应的todo对象删除
// this.deleteTodo(id)
// this.$bus.$emit('deleteTodo',id)
pubsub.publish("deleteTodo", id)
}
},
//编辑
handleEdit(todo) {
if (todo.hasOwnProperty("isEdit")) {
todo.isEdit = true
} else {
this.$set(todo, "isEdit", true)
}
this.$nextTick(function () {
this.$refs.inputTitle.focus()
})
},
// 失去焦点回调(真正执行修改逻辑)
handleBlur(todo, e) {
todo.isEdit = false
if (!e.target.value.trim()) return alert("输入不能为空!")
this.$bus.$emit("updateTodo", todo.id, e.target.value)
},
},
}
</script>
<style scoped>
/*item*/
li {
list-style: none;
height: 36px;
line-height: 36px;
padding: 0 5px;
border-bottom: 1px solid #ddd;
}
li label {
float: left;
cursor: pointer;
}
li label li input {
vertical-align: middle;
margin-right: 6px;
position: relative;
top: -1px;
}
li button {
float: right;
display: none;
margin-top: 3px;
}
li:before {
content: initial;
}
li:last-child {
border-bottom: none;
}
li:hover {
background-color: #ddd;
}
li:hover button {
display: block;
}
/* .todo-enter-active {
animation: atguigu 0.5s linear;
}
.todo-leave-active {
animation: atguigu 1s reverse;
}
@keyframes atguigu {
from {
transform: translateX(100%);
}
to {
transform: translateX(0px);
}
} */
</style>
- src/components/MyList.vue
<template>
<ul class="todo-main">
<transition-group name="todo" appear>
<MyItem v-for="todoObj in todos" :key="todoObj.id" :todo="todoObj" />
</transition-group>
</ul>
</template>
<script>
import MyItem from "./MyItem"
export default {
name: "MyList",
components: { MyItem },
props: ["todos"],
}
</script>
<style scoped>
/*main*/
.todo-main {
margin-left: 0px;
border: 1px solid #ddd;
border-radius: 2px;
padding: 0px;
}
.todo-empty {
height: 40px;
line-height: 40px;
border: 1px solid #ddd;
border-radius: 2px;
padding-left: 5px;
margin-top: 10px;
}
.todo-enter-active {
animation: atguigu 0.5s linear;
}
.todo-leave-active {
animation: atguigu 1s reverse;
}
@keyframes atguigu {
from {
transform: translateX(100%);
}
to {
transform: translateX(0px);
}
}
</style>
效果:
★★★ 欢迎批评指正!!!