Dice (III)
Given a dice with n sides, you have to find the expected number of times you have to throw that dice to see all its faces at least once. Assume that the dice is fair, that means when you throw the dice, the probability of occurring any face is equal.
For example, for a fair two sided coin, the result is 3. Because when you first throw the coin, you will definitely see a new face. If you throw the coin again, the chance of getting the opposite side is 0.5, and the chance of getting the same side is 0.5. So, the result is
1 + (1 + 0.5 * (1 + 0.5 * ...))
= 2 + 0.5 + 0.52 + 0.53 + ...
= 2 + 1 = 3
Input
Input starts with an integer T (≤ 100), denoting the number of test cases.
Each case starts with a line containing an integer n (1 ≤ n ≤ 105).
Output
For each case, print the case number and the expected number of times you have to throw the dice to see all its faces at least once. Errors less than 10-6 will be ignored.
Sample Input
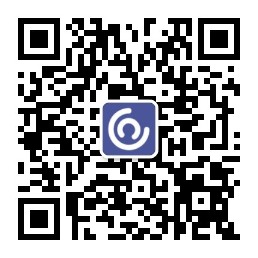
5
1
2
3
6
100
Sample Output
Case 1: 1
Case 2: 3
Case 3: 5.5
Case 4: 14.7
Case 5: 518.7377517640
假设已经转出了第i面,则转出第i+1面的概率是1/(n-i)
E=n* p
#include<cstdio>
#include<algorithm>
#include<iostream>
using namespace std;
int main()
{
int T,j,n,i;
double p;
scanf("%d",&T);
for(j=1;j<=T;j++)
{
scanf("%d",&n);
p=0;
for(i=1;i<=n;i++)
p+=1.0/i;
printf("Case %d: %.7lf\n",j,p*n);
}
return 0;
}
Island of Survival
You are in a reality show, and the show is way too real that they threw into an island. Only two kinds of animals are in the island, the tigers and the deer. Though unfortunate but the truth is that, each day exactly two animals meet each other. So, the outcomes are one of the following
a) If you and a tiger meet, the tiger will surely kill you.
b) If a tiger and a deer meet, the tiger will eat the deer.
c) If two deer meet, nothing happens.
d) If you meet a deer, you may or may not kill the deer (depends on you).
e) If two tigers meet, they will fight each other till death. So, both will be killed.
If in some day you are sure that you will not be killed, you leave the island immediately and thus win the reality show. And you can assume that two animals in each day are chosen uniformly at random from the set of living creatures in the island (including you).
Now you want to find the expected probability of you winning the game. Since in outcome (d), you can make your own decision, you want to maximize the probability.
Input
Input starts with an integer T (≤ 200), denoting the number of test cases.
Each case starts with a line containing two integers t (0 ≤ t ≤ 1000) and d (0 ≤ d ≤ 1000) where t denotes the number of tigers and d denotes the number of deer.
Output
For each case, print the case number and the expected probability. Errors less than 10-6 will be ignored.
Sample Input
4
0 0
1 7
2 0
0 10
Sample Output
Case 1: 1
Case 2: 0
Case 3: 0.3333333333
Case 4: 1
要想求出人活下来的概率,和鹿的数量没关系
老虎的数量为t
当老虎和老虎碰面时,两只老虎全死
如果t为奇数,则人一定不会存活
如果t为偶数,则人能活下来的碰面有C(t+1,1)种可能,从t+1个动物里选出一个,直到最后也不碰面,所以有1/(t+1)种可能
#include<cstdio>
#include<algorithm>
#include<iostream>
using namespace std;
int main()
{
int t,d,T,j;
double p;
scanf("%d",&T);
for(j=1;j<=T;j++)
{
scanf("%d %d",&t,&d);
if(t%2==0)
p=1.0/(t+1);
else
p=0;
printf("Case %d: %lf\n",j,p);
}
return 0;
}
Throwing Balls into the Baskets
You probably have played the game "Throwing Balls into the Basket". It is a simple game. You have to throw a ball into a basket from a certain distance. One day we (the AIUB ACMMER) were playing the game. But it was slightly different from the main game. In our game we were N people trying to throw balls into M identical Baskets. At each turn we all were selecting a basket and trying to throw a ball into it. After the game we saw exactly S balls were successful. Now you will be given the value of N and M. For each player probability of throwing a ball into any basket successfully is P. Assume that there are infinitely many balls and the probability of choosing a basket by any player is 1/M. If multiple people choose a common basket and throw their ball, you can assume that their balls will not conflict, and the probability remains same for getting inside a basket. You have to find the expected number of balls entered into the baskets after K turns.
Input
Input starts with an integer T (≤ 100), denoting the number of test cases.
Each case starts with a line containing three integers N (1 ≤ N ≤ 16), M (1 ≤ M ≤ 100) and K (0 ≤ K ≤ 100) and a real number P (0 ≤ P ≤ 1). P contains at most three places after the decimal point.
Output
For each case, print the case number and the expected number of balls. Errors less than 10-6 will be ignored.
Sample Input
2
1 1 1 0.5
1 1 2 0.5
Sample Output
Case 1: 0.5
Case 2: 1.000000
这题挺坑的,以为要跟着模板的思路,混乱//qwq
结果其实炒鸡简单,和投进哪个篮子的概率没有关系
有k轮,每轮n个人投一次,每个人投进的概率是p
那么总的能投进球的概率则是k*p*n
#include<cstdio>
#include<algorithm>
#include<iostream>
using namespace std;
int main()
{
int t,n,j,k,m;
double p;
scanf("%d",&t);
for(j=1;j<=t;j++)
{
scanf("%d %d %d %lf",&n,&m,&k,&p);
printf("Case %d: %lf\n",j,k*n*p);
}
return 0;
}
Discovering Gold
You are in a cave, a long cave! The cave can be represented by a 1 x N grid. Each cell of the cave can contain any amount of gold.
Initially you are in position 1. Now each turn you throw a perfect 6 sided dice. If you get X in the dice after throwing, you add X to your position and collect all the gold from the new position. If your new position is outside the cave, then you keep throwing again until you get a suitable result. When you reach the Nth position you stop your journey. Now you are given the information about the cave, you have to find out the expected number of gold you can collect using the given procedure.
Input
Input starts with an integer T (≤ 100), denoting the number of test cases.
Each case contains a blank line and an integer N (1 ≤ N ≤ 100) denoting the dimension of the cave. The next line contains N space separated integers. The ith integer of this line denotes the amount of gold you will get if you come to the ith cell. You may safely assume that all the given integers will be non-negative and no integer will be greater than 1000.
Output
For each case, print the case number and the expected number of gold you will collect. Errors less than 10-6 will be ignored.
Sample Input
3
1
101
2
10 3
3
3 6 9
Sample Output
Case 1: 101.0000000000
Case 2: 13.000
Case 3: 15
这题师哥讲课的时候讲过
emmm//记住:期望逆着推,概率正着推
#include<cstdio>
#include<algorithm>
#include<iostream>
using namespace std;
int main()
{
int t,n,i,j,k,a[1005];
double dp[1005];
scanf("%d",&t);
for(j=1;j<=t;j++)
{
scanf("\n");
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a[i]);
dp[i]=a[i];
}
for(i=n;i>=1;i--)
{
int x =min(6,n-i);
for(k=1;k<=x;k++)
{
dp[i]+=1.0/x*dp[i+k];
}
}
printf("Case %d: %lf\n",j,dp[1]);
}
return 0;
}