第6次全天课笔记 20180819
习题:
找到列表中第二大的数,可以用多种方法解决。
思路1:
找到最大的,删除掉,再找最大的
list1 = [5,8,9,34,214,54,22]
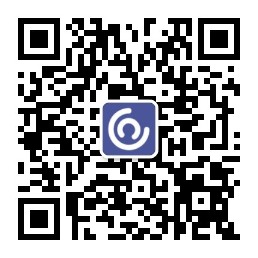
list1_max = max(list1)
print(list1_max)
list1.remove(list1_max)
list1_max = max(list1)
print(list1_max)
思路2:
排好序找倒数第二个
list1 = [5,8,9,34,214,54,22]
list1.sort() #原地排序 ; sorted(list1) 非原地排序,list1不改变
print(list1[-2])
思路3:
遍历,声明两个变量,一个存最大的
一个存第二大的,然后逐一比对。
s = [1,2,3,4,5,-1,-2]
max_num = s[0]
second_max_num = s[0]
for i in s:
if i> max_num:
second_max_num = max_num
max_num = i
print("第二大的元素是:",second_max_num)
习题2:
python代码得到2个列表的交集与差集
不许用set
交集思路:遍历list1,判断是否在list2中,
在的话,则存入一个列表中。
差集思路:分别遍历list1和2,如果不在对方的
list中,则存入一个列表中
s1 = [1,2,3,9,10]
s2=[2,3,9,11]
result = []
for i in s1:
if i in s2:
result.append(i)
print("交集:",result)
差集:
s1 = [1,2,3,9,10]
s2 = [2,3,9,11]
result = []
for i in s1:
if i not in s2:
result.append(i)
for i in s2:
if i not in s1:
result.append(i)
print("差集:",result)
差集2的实现方法: 2个集合的并集-交集=差集
s1 = [1,2,3,9,10]
s2=[2,3,9,11]
result1 = []
result2 = []
for i in s1:
if i in s2:
result1.append(i)
print("交集:",result1)
s1=list(set(s1+s2))
for i in s1:
if i not in result1:
result2.append(i)
print("差集:",result2)
冒泡排序:
s = [-1,1,-2,2,3,-3]
第一步:交换2个元素
交换的方法1:
a,b = b,a
交换2:
temp=b
b=a
a=temp
第二步:我要找到这个list中最大的元素,把它放到列表的最后位置
位置0和位置1比,如果大,交换,否则不换
位置1和位置2位置比,如果大,交换,否则不换
位置2和位置3位置比,如果大,交换,否则不换
...
位置4和和位置5位置比,如果大,交换,否则不换
1)基于坐标做遍历,最后一个元素不需要做遍历动作
遍历的反射:
1 把元素逐一取出来
2 基于坐标位置逐一取出来,i,i+1
s = [-1,1,-2,2,3,-3]
for i in range(len(s)-1):
if s[i] > s[i+1]:
temp=s[i]
s[i]=s[i+1]
s[i+1]=temp
print (s)
2)使用if当前元素和后一个元素做比对,如果大了,交换,否则啥也不干
把最后1个元素我不处理了,把之前的所有数,在做相同工作,第二大
s = [-1,1,-2,2,3,-3]
for i in range(len(s)-1-i):
if s[i] > s[i+1]:
temp=s[i]
s[i]=s[i+1]
s[i+1]=temp
print (s)
把最后2个元素我不处理了,把之前的所有数,在做相同工作,第三大
把最后3个元素我不处理了,把之前的所有数,在做相同工作,第四大
.....
把最后n-1个元素我不处理了,把之前的所有数,在做相同工作,第n大
s = [-1,1,-2,2,3,-3]
for j in range(len(s)-1):
for i in range(len(s)-1-j):
if s[i] > s[i+1]:
temp=s[i]
s[i]=s[i+1]
s[i+1]=temp
print(s)
dir(__builtins__) #查看内置的
help(round) #查看类或类似
不推荐使用关键字作为函数名,因为会覆盖已有函数
函数:类外面的函数定义叫做函数
方法:类内部定义的函数叫做方法
求一个字符串中的字母个数函数
需判断传入参数的类型。
isinstance(s,str)
加个要求,必须使用ascii来判断是否字母
def count_letter_num(s):
if not isinstance(s,str):
return None
result = 0
for i in s:
if (ord(i) >= ord("a") and ord(i) <= ord("z")) \
or (ord(i) >= ord("A") and ord(i) <= ord("Z")):
result +=1
return result
print("包含的字母数量:",count_letter_num("I am a boy!"))
>>> def print_sth(s):
... "此函数用于打印" #文档字符串
... print(s)
...
>>> print_sth("hi")
hi
>>> print_sth.__doc__
'此函数用于打印'
>>> help(print_sth)
Help on function print_sth in module __main__:
print_sth(s)
此函数用于打印
>>> import os
>>> os.getcwd()
'E:\\'
#时间戳 从1970-1-1 00:00
>>> import time
>>> time.time()
1534649995.2979999
写一个函数,这个函数要计算浮点数乘法的一万次相乘后的时间耗时,浮
点数可以使用随机小数
def count_float_compute_speed(n):
import random
import time
start_time = time.time()
for i in range(n):
result=random.random()**2
end_time = time.time()
return end_time-start_time
print(count_float_compute_speed(10000000))
不可变对象:所有的数字、字符串、元组
可变:set dict list 实例
参数传不可变对象的时候,函数内外变量值是独立的
参数传可变对象的时候,函数内外变量值是关联的
在函数内部,使用global变量,会对外部变量有影响。
a=100
def compute():
global a
a+=1
compute()
print (a)
#a改变了,是101
def add_end(L=[]):
L.append('END')
return L
print (add_end([1, 2, 3])) #调用正常
print (add_end(['x', 'y', 'z'])) #调用正常
print (add_end())#调用正常
print (add_end())#调用不正常
print (add_end())#调用不正常
修改成
def add_end(L=None):
if L is None:
L =[]
L.append('END')
return L
print (add_end([1, 2, 3])) #调用正常
print (add_end(['x', 'y', 'z'])) #调用正常
print (add_end())#调用正常
print (add_end())#调用正常
print (add_end())#调用正常
默认参数值,将推荐值作为默认值
>>> def add(a,b=1,c=2):
... return a+b+c
...
>>> print(add(1,2,3))
6
>>> print(add(1,2))
5
>>> print(add(1))
4
>>> "******abdc********".strip("*")
'abdc'
>>> "******abdc********".lstrip("*")
'abdc********'
>>> "******abdc********".rstrip("*")
'******abdc'
去除空白字符,包括 空格 /r /n /t 。
小练习:
add(a,b)
要求有个值是result来存结果
1 a,b 数字,相加
2 a,b 字符串,就当做字符串相加
3 a,b 如果list就当list相加
4 不满足条件,则返回None
def add(a,b):
if isinstance(a,(int,float)) and isinstance(b,(int,float)):
result = a+b
elif isinstance(a,str) and isinstance(b,str):
result = a+b
elif isinstance(a,list) and isinstance(b,list):
result = a+b
else:
return None
return result
print(add(2,3))
print(add("a","b"))
print(add([1,2],[3,4]))
print(add("a",3))
print(add([1,2],3))
命名参数
>>> def add(a,b,c):
... return a+b+c
...
>>> print(add(c=1,b=2,a=3))
6
>>> print(add(c=1,2,3))
File "<stdin>", line 1
SyntaxError: positional argument follows keyword argu
命名参数必须放在非命名参数的后面
>>> a,b = 1,2
>>> a
1
>>> b
2
>>> a,b = (1,2)
>>> a
1
>>> b
2
>>> def func(a,b):
... return a,b
...
func(1,2) ->返回一个元组 (1,2)
正向代理:
客户端和服务器之间的一个服务器或者是软件,负责转发用户的
请求到源服务器。
发给不同的公司服务器
在你公司的局域网,某第三方服务器。
反向代理:
在被访问服务器的局域网内,你的请求被它收到了之后,
会被反向代理服务器解析你的请求的url,然后将请求转发
给不同的服务器处理。
反向代理+你要访问的服务器是一组,是一个公司开发的。
外人不知道。
平台,接口层:
10台服务器,1万用户
1台1-1000
2台1001-2000
。。。。
10台9000-10000
{ip:“13.2.3.3”,userid:"1"}
解析你的url--->转发相应的服务器
Rewrite
练习:
输入5个字母,声明一个可变参数的函数,拼成一个单词。
元组作为函数参数
def word(*arg):
result=""
for i in arg:
result += i
return result
print(word("h","e","l","l","o"))
print(word("h","eddd","le","l2","o3"))
字典作为函数参数
def add(**kw):
print(type(kw))
for i in kw:
print(i)
for j in kw.values():
print(j)
print(add(a="1",b=2,c=3))
题目:使用必填参数、默认参数、可变元组参数、可变字典参数计算一下单词的长度之和。
def count_letter_num(a,b="abc",*arg,**kw):
result = 0
result += len(a)
result += len(b)
print(arg)
print(kw)
for i in arg:
result+=len(i)
for i in kw.values():
result+=len(i)
return result
#"a"赋值给参数a了,"b"给参数b了,cde给arg,x和y给kw做key
#f和g作为kw的value
print(count_letter_num("a","b","c","d","e",x="f",y="g"))
print(count_letter_num("a"))
print(count_letter_num("a",c="x"))
lambda函数
六剑客解决一行代码:
Lambda
切片
Map
Filter
Reduce
推倒列表
Repr方法
>>> s=repr([1,2,3])
>>> eval(s)
[1, 2, 3]
>>> s=repr((1,2,3))
>>> eval(s)
(1, 2, 3)
>>> class P():
... def __str__(self):
... return "hi"
... def __repr__(self):
... return "ggggg"
...
>>> p=P()
>>> p
ggggg
>>> str(p)
'hi'
>>> type(1)
<class 'int'>
>>> type(1.1)
<class 'float'>
>>> type(1+1j)
<class 'complex'>
>>> type("a")
<class 'str'>
>>> type([1])
<class 'list'>
>>> type((1,2))
<class 'tuple'>
>>> type({1:1})
<class 'dict'>
>>> type(set([1])) #集合
<class 'set'>
>>> type({1,2})
<class 'set'>
>>>
Map
大写变小写
list(map(lambda x:x+1,[1,2,3]))
list(map(lambda x:chr(ord(x)+32),["A","B","C"]))
list(map(lambda x:x.lower(),["A","B","C"]))
>>> def l(s):return s.lower()
...
>>> list(map(l,"ABCabc"))
['a', 'b', 'c', 'a', 'b', 'c']
Filter
#过滤偶数
>>> def f(n):
... if n % 2 ==0:
... return n
...
>>> list(filter(f,[1,2,3,4]))
[2, 4]
#过滤小写字母
>>> def f(n):
... if n >="a" and n <="z":
... return n
...
>>> list(filter(f,"AAaaBBbb"))
['a', 'a', 'b', 'b']
Reduce:
from functools import reduce
>>> reduce(lambda x,y:x+y,[1,2,3,4])
10
第一次计算:x=1,y=2
第二次计算:x=3,y =3
第三次计算:x=6,y=4
6+4=10
递归
1) 调用自己
2) 有结束的判断
累加:1+2+3+4+5
result =0
for i in range(1,6):
result +=i
def add(n):
if n ==1:
return 1
return add(n-1)+n
n=3:第一次调用:add(3-1)+3--->add(2)+3=3+3=6
n=2:第二次调用:add(2-1)+2--->add(1)+2=1+2=3
n=1:第三次调用:add(1)--->return 1