版权声明:版权所有,转载请注明原网址链接。 https://blog.csdn.net/qq_41231926/article/details/81937355
原题链接:https://leetcode-cn.com/problems/palindrome-number/description/
题目描述:
知识点:字符串、数学
思路一:将整数转为字符串
注意判断字符串是否是回文序列只需要遍历字符串一半的长度即可。
时间复杂度是O(n),其中n为整数的位数。空间复杂度也为O(n)。
JAVA代码:
public class Solution {
public boolean isPalindrome(int x) {
String string = Integer.toString(x);
int n = string.length();
for (int i = 0; i <= n / 2; i++) {
if(string.charAt(i) != string.charAt(n - i - 1)) {
return false;
}
}
return true;
}
}
LeetCode解题报告:
思路二:利用一个ArrayList存储数字中每一位的值
此方法的时间复杂度是O(n)级别的,其中n为整数的位数。空间复杂度也是O(n)。
JAVA代码:
扫描二维码关注公众号,回复:
2895710 查看本文章
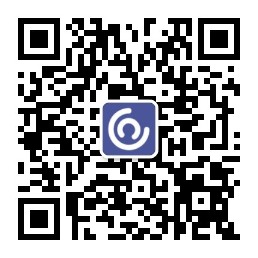
public class Solution {
public boolean isPalindrome(int x) {
if(x < 0) {
return false;
}
if(x == 0) {
return true;
}
ArrayList<Character> arrayList = new ArrayList<>();
while(x > 0) {
arrayList.add((char)(x % 10));
x /= 10;
}
for (int i = 0; i <= arrayList.size() / 2; i++) {
if(arrayList.get(i) != arrayList.get(arrayList.size() - 1 - i)) {
return false;
}
}
return true;
}
}
LeetCode解题报告:
思路三:依次比较数字的最高位和最低位是否相等
此方法的时间复杂度是O(n)级别的,其中n为整数的位数。空间复杂度是O(1)。
JAVA代码:
public class Solution {
public boolean isPalindrome(int x) {
if(x < 0) {
return false;
}
if(x <= 9) {
return true;
}
int num = 0;
int temp = x;
while(temp > 0) {
temp /= 10;
num++;
}
while(num > 1) {
int left = x / (int)(Math.pow(10, num - 1));
int right = x % 10;
if(left != right) {
return false;
}
x = (x - right - left * (int)(Math.pow(10, num - 1))) / 10;
num -= 2;
}
return true;
}
}
LeetCode解题报告:
思路四:翻转数字,判断是否和原数字相等
翻转过程中注意越界的情况,如果越界,直接返回false。事实上如果翻转之后越界了,肯定就不会和原数字相等了,我们在代码实现时无需考虑这种情况。
翻转过程:
设置一个int类型的reverse,其初值为0,只要x > 0,就继续循环, 每一轮循环执行reverse = reverse * 10 + x % 10;和x /= 10;的操作。当然一开始我们要先设一个变量记录x的原值。
此方法的时间复杂度是O(n)级别的,其中n为整数的位数。空间复杂度是O(1)。
JAVA代码:
public class Solution {
public boolean isPalindrome(int x) {
if(x < 0) {
return false;
}
if(x <= 9) {
return true;
}
int reverse = 0;
int temp = x;
while(temp > 0) {
reverse = reverse * 10 + temp % 10;
temp = temp / 10;
}
return reverse == x;
}
}
LeetCode解题报告: