手机下载串口助手,定义协议,前后左右几个按键编辑发送的字符串
USART2接蓝牙,蓝牙接收到数据后透传给串口2,从蓝牙得到数据分析后进行处理。控制四个电机,还可以扩展
USART2配置
/*
uasrt2接受数据,并且分析数据
*/
void NVIC_Configuration_2(void)
{
NVIC_InitTypeDef NVIC_InitStructure;
/* 閰嶇疆 NVIC 涓轰紭鍏堢骇缁?1 */
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2);
/* 閰嶇疆涓柇婧愶細鎸夐敭 1 */
NVIC_InitStructure.NVIC_IRQChannel = USART2_IRQn;
/* 閰嶇疆鎶㈠崰浼樺厛绾э細 1 */
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 1;
/* 閰嶇疆瀛愪紭鍏堢骇锛?1 */
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1;
/* 浣胯兘涓柇閫氶亾 */
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
}
void USART2_Config(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
/* config USART1 clock */
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
/* USART1 GPIO config */
/* Configure USART1 Tx (PA.09) as alternate function push-pull */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
/* Configure USART1 Rx (PA.10) as input floating */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
/* USART1 mode config */
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No ;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART2, &USART_InitStructure);
// 涓插彛涓柇浼樺厛绾ч厤缃?
NVIC_Configuration_2();
// 閰嶇疆瀹?NVIC 涔嬪悗璋冪敤 USART_ITConfig 鍑芥暟浣胯兘 USART 鎺ユ敹涓柇銆?
USART_ITConfig(USART2, USART_IT_RXNE, ENABLE);
USART_Cmd(USART2, ENABLE);
}
void USART_Config(void)
{
USART1_Config();
USART2_Config();
}
char get_usart2_buf[4];
void gRxHostBufferFlush(u32 BufferSize,char *buf){
for(u32 counter=0; counter<BufferSize; counter++) buf[counter] = 0;
}
char get_usart2_i=0;
bool get_usart2_buffer = FALSE;
void USART2_IRQHandler_FUN(void)
{
u8 data;
(void)USART2->SR; //Error clear;
data = (u8)(USART2->DR & (u16)0x01FF);
USART_ClearITPendingBit(USART2, USART_IT_RXNE);
get_usart2_buffer = FALSE;
get_usart2_buf[get_usart2_i] = data;
USART_SendData(USART1, get_usart2_buf[get_usart2_i]);
get_usart2_i++;
if(get_usart2_buf[0] != 0xFF)
{
get_usart2_i=0;
}
if(get_usart2_i==4)
{ get_usart2_buffer = TRUE;
AnalizingBuf();
get_usart2_i = 0;
gRxHostBufferFlush(4,get_usart2_buf);
}
}
void AnalizingBuf(void)
{
if(get_usart2_buffer == FALSE)
return;
switch (get_usart2_buf[2])
{
case 1://涓?
switch (get_usart2_buf[3])
{
case 1://鎸変笅
car_front;
printf("forward down\n");
break;
case 0://鎸夋姮璧?
car_stop;
printf("forward up\n");
break;
default :
break;
}
break;
case 2://涓?
switch (get_usart2_buf[3])
{
case 1://鎸変笅
car_rear;
printf("back on\n");
break;
case 0://鎸夋姮璧?
car_stop;
printf("back off\n");
break;
default :
break;
}
break;
case 3://涓棿
switch (get_usart2_buf[3])
{
case 1://鎸変笅
printf("centre on\n");
break;
case 0://鎸夋姮璧?
printf("centre off\n");
break;
default :
break;
}
break;
case 4://宸?
switch (get_usart2_buf[3])
{
case 1://鎸変笅
car_left;
printf("left on\n");
break;
case 0://鎸夋姮璧?
car_stop;
printf("left off \n");
break;
default :
break;
}
break;
case 5://鍙?
switch (get_usart2_buf[3])
{
case 1://鎸変笅
car_right;
printf("right on \n");
break;
case 0://鎸夋姮璧?
car_stop;
printf("right off\n");
break;
default :
break;
}
break;
default :
break;
}
}
GPIO配置
#define front_ch1(BitVal) GPIO_WriteBit( GPIOB, GPIO_Pin_12,(BitAction) BitVal)
#define front_ch2(BitVal) GPIO_WriteBit( GPIOB, GPIO_Pin_13, (BitAction)BitVal)
#define front_ch3(BitVal) GPIO_WriteBit( GPIOB, GPIO_Pin_14, (BitAction)BitVal)
#define front_ch4(BitVal) GPIO_WriteBit( GPIOB, GPIO_Pin_15, (BitAction)BitVal)
#define rear_ch1(BitVal) GPIO_WriteBit( GPIOC, GPIO_Pin_2, (BitAction)BitVal)
#define rear_ch2(BitVal) GPIO_WriteBit( GPIOC, GPIO_Pin_3,(BitAction) BitVal)
#define rear_ch3(BitVal) GPIO_WriteBit( GPIOC, GPIO_Pin_4,(BitAction) BitVal)
#define rear_ch4(BitVal) GPIO_WriteBit( GPIOC, GPIO_Pin_5, (BitAction)BitVal)
#define front_stop front_ch1(1);front_ch2(1);front_ch3(1);front_ch4(1)
#define rear_stop rear_ch1(1);rear_ch2(1);rear_ch3(1);rear_ch4(1)
#define front_start_z front_ch1(0);front_ch2(1);front_ch3(0);front_ch4(1)
#define front_start_f front_ch1(1);front_ch2(0);front_ch3(1);front_ch4(0)
#define rear_start_z rear_ch1(0);rear_ch2(1);rear_ch3(0);rear_ch4(1)
#define rear_start_f rear_ch1(1);rear_ch2(0);rear_ch3(1);rear_ch4(0)
#define front_start_left front_ch1(1);front_ch2(0);front_ch3(0);front_ch4(1)
#define front_start_right front_ch1(0);front_ch2(1);front_ch3(1);front_ch4(0)
#define rear_start_left rear_ch1(1);rear_ch2(0);rear_ch3(0);rear_ch4(1)
#define rear_start_right rear_ch1(0);rear_ch2(1);rear_ch3(1);rear_ch4(0)
#define car_front front_start_z;rear_start_z
#define car_rear front_start_f;rear_start_f
#define car_left front_start_left;rear_start_left
#define car_right front_start_right;rear_start_right
#define car_stop front_stop;rear_stop
单片机最小系统
轮子X4
电机控制模块X2
蓝牙模块
小车底板
扫描二维码关注公众号,回复:
2945474 查看本文章
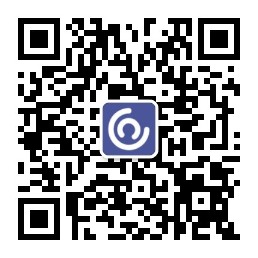
最小系统