版权声明:希望各位多提意见多多互相交流哦~ https://blog.csdn.net/wait_for_taht_day5/article/details/82935226
文章目录
232. Implement Queue using Stacks
Implement the following operations of a queue using stacks.
- push(x) – Push element x to the back of queue.
- pop() – Removes the element from in front of queue.
- peek() – Get the front element.
- empty() – Return whether the queue is empty.
Example:
MyQueue queue = new MyQueue();
queue.push(1);
queue.push(2);
queue.peek(); // returns 1
queue.pop(); // returns 1
queue.empty(); // returns false
Notes:
- You must use only standard operations of a stack – which means only
push to top
,peek/pop from top
,size
, andis empty
operations are valid. - Depending on your language, stack may not be supported natively. You may simulate a stack by using a list or deque (double-ended queue), as long as you use only standard operations of a stack.
- You may assume that all operations are valid (for example, no pop or peek operations will be called on an empty queue).
Solution in C++:
关键点:
- queue FIFO stack FILO
思路:
- 开始打算用两个栈来实现一个队列的,后来想想还是一个栈一个数组比较方便,哈哈其实有点偷巧啦,其实实现原理差不多就不要纠结了,之后栈和队列的专题里面会有这方面的实现整理。我这里主要是每次push的时候将原先stack中的元素放入数组中,将push的元素压入栈底,然后将从栈中pop的元素按倒序再push回栈中就保持了原来的顺序。
class MyQueue {
public:
stack<int> mystack;
vector<int> backup;
/** Initialize your data structure here. */
MyQueue() {
}
/** Push element x to the back of queue. */
void push(int x) {
if (mystack.empty())
mystack.push(x);
else{
backup.clear();
while(!mystack.empty())
{
backup.push_back(mystack.top());
mystack.pop();
}
mystack.push(x);
size_t size = backup.size();
for ( int i = size - 1; i >= 0; --i)
{
mystack.push(backup[i]);
}
}
}
/** Removes the element from in front of queue and returns that element. */
int pop() {
int result = mystack.top();
mystack.pop();
return result;
}
/** Get the front element. */
int peek() {
return mystack.top();
}
/** Returns whether the queue is empty. */
bool empty() {
return mystack.empty();
}
};
/**
* Your MyQueue object will be instantiated and called as such:
* MyQueue obj = new MyQueue();
* obj.push(x);
* int param_2 = obj.pop();
* int param_3 = obj.peek();
* bool param_4 = obj.empty();
*/
234. Palindrome Linked List
Given a singly linked list, determine if it is a palindrome.
Example 1:
**Input**: 1->2
**Output**: false
Example 2:
**Input**: 1->2->2->1
**Output**: true
Follow up:
Could you do it in O(n) time and O(1) space?
Solution in C++:
扫描二维码关注公众号,回复:
3550287 查看本文章
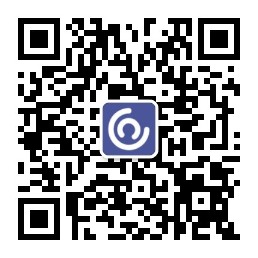
关键点:
- O(1) space
思路:
- 哎,渣渣的我只能想到用O(n)空间的方法,然后去看了一下leetcode中discuss的一些答案感觉有点差强人意,我觉得用递归方法实现的方法并不能算是O(1)space的方法,所以去网上搜了一些,感觉还不错。大致方法是首先找到链表的中心,然后根据元素的奇数偶数情况,将后半部分链表反转,然后从head和反转后的rehead开始一个一个判断值的情况。
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
ListNode *reverseList(ListNode *head){
if (head == nullptr || head->next == nullptr)
return head;
ListNode *p = reverseList(head->next);
head->next->next = head;
head->next = nullptr;
return p;
}
bool isPalindrome(ListNode* head) {
if (!head || !head->next)
return true;
ListNode *slow, *fast;
slow = fast = head;
// 找到链表中心,slow指向
while(fast && fast->next)
{
slow = slow->next;
fast = fast->next->next;
}
if (fast)
{
// 链表元素个数为奇数
slow->next = reverseList(slow->next);
slow = slow->next;
} else{
// 链表元素个数为偶数
slow = reverseList(slow);
}
while(slow)
{
if (head->val != slow->val)
return false;
slow = slow->next;
head = head->next;
}
return true;
}
};
237. Delete Node in a Linked List
Write a function to delete a node (except the tail) in a singly linked list, given only access to that node.
Given linked list – head = [4,5,1,9], which looks like following:
4 -> 5 -> 1 -> 9
Example 1:
**Input**: head = [4,5,1,9], node = 5
**Output**: [4,1,9]
**Explanation**: You are given the second node with value 5, the linked list
should become 4 -> 1 -> 9 after calling your function.
Example 2:
**Input**: head = [4,5,1,9], node = 1
**Output**: [4,5,9]
**Explanation**: You are given the third node with value 1, the linked list
should become 4 -> 5 -> 9 after calling your function.
Note:
- The linked list will have at least two elements.
- All of the nodes’ values will be unique.
- The given node will not be the tail and it will always be a valid node of the linked list.
- Do not return anything from your function.
Solution in C++:
关键点:
- 非常规思路
思路:
- 开始还以为是很简单的题目,还以为原来做过,但是发现提供的函数参数和原本想的有点不一样,这题可能就是用来锻炼大脑的思维,不要陷入常规的删除方法,这个提供的新思路对我来说也是很不错的,虽然看到有的人在解析下评论表示不看好,但我觉得还挺不错的~主要就是通过修改当前结点的值为下一个结点的值,然后删除下一个结点的方式来达到删除本结点的效果。
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
void deleteNode(ListNode* node) {
ListNode *next = node->next;
node->val = next->val;
node->next = next->next;
free(next);
}
};
小结
今天学习到了一些新思路,不错不错,同时也发现了一些可以总结的算法思路点,从现在开始会开始整理一些核心算法或数据结构的专题,再就是个人觉得思维有帮助的。
知识点
- stack implement queue
- 回文链表 反转链表
- 通过修改值删除当前结点