492. Construct the Rectangle
For a web developer, it is very important to know how to design a web page’s size. So, given a specific rectangular web page’s area, your job by now is to design a rectangular web page, whose length L and width W satisfy the following requirements:
1. The area of the rectangular web page you designed must equal to the given target area.
2. The width W should not be larger than the length L, which means L >= W.
3. The difference between length L and width W should be as small as possible.
You need to output the length L and the width W of the web page you designed in sequence.
Example:
Input: 4
Output: [2, 2]
Explanation: The target area is 4, and all the possible ways to construct it are [1,4], [2,2], [4,1].
But according to requirement 2, [1,4] is illegal; according to requirement 3, [4,1] is not optimal compared to [2,2]. So the length L is 2, and the width W is 2.
Note:
- The given area won’t exceed 10,000,000 and is a positive integer
- The web page’s width and length you designed must be positive integers.
Solution in C++:
关键点:
- 折半
思路:
- 因为看到例子里面有完全平方数的例子,让我觉得可以从这里下手,首先是如果是完全平方数的话那就返回两个相等的即可,这里我之前犯了个错误,我认为 area % i == 0即可代表完全平方数,在测试6的时候出现了错误,所以这里换回了
== area的判断条件。然后就是下面的逻辑,还是拿6和5来举例,正确答案分别应该是3 * 2和5*1这里一个可以看出来
l
的最大值应该要么是可以完全平方求根号之后的结果要么是比它大的,然后再就是w
一定是大于1的,所以就出现了下面的循环操作。
vector<int> constructRectangle(int area) {
vector<int> result;
int l, m;
l = sqrt(area);
if ( l* l == area){ // 是完全平方数
result.push_back(l);
result.push_back(l);
} else{
l = ceil(area*1.0/l);
while(l >= 1){
if (area % l == 0)
{
int tmp = max(l, area/l);
m = area / tmp;
l = tmp;
break;
}
--l;
}
result.push_back(l);
result.push_back(m);
}
return result;
}
496. Next Greater Element I
You are given two arrays (without duplicates) nums1
and nums2
where nums1
’s elements are subset of nums2
. Find all the next greater numbers for nums1
's elements in the corresponding places of nums2
.
The Next Greater Number of a number x in nums1
is the first greater number to its right in nums2
. If it does not exist, output -1 for this number.
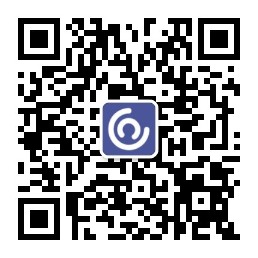
Example 1:
Input: nums1 = [4,1,2], nums2 = [1,3,4,2].
Output: [-1,3,-1]
Explanation:
For number 4 in the first array, you cannot find the next greater number for it in the second array, so output -1.
For number 1 in the first array, the next greater number for it in the second array is 3.
For number 2 in the first array, there is no next greater number for it in the second array, so output -1.
Example 2:
Input: nums1 = [2,4], nums2 = [1,2,3,4].
Output: [3,-1]
Explanation:
For number 2 in the first array, the next greater number for it in the second array is 3.
For number 4 in the first array, there is no next greater number for it in the second array, so output -1.
Note:
- All elements in
nums1
andnums2
are unique. - The length of both
nums1
andnums2
would not exceed 1000.
Solution in C++:
易错点:
- 本位置第一个大的数不一定是右边最大的数
思路:
- 最开始我的想法很naive,使用
map
记录nums
里面每个位置对应的第一个大的数的值就可以了,然后为了简化,我还认为从后到前的方式只用比较后一个和其对应的value即可,但是后来发现这里的逻辑bug就是我上面提到的易错点,然后我修改了这个比较逻辑,改成了从当前位置一直扫描到比自己大的数,(其实这里可以优化到扫描到value为-1的数,然后比较和那个数的大小情况即可)。
vector<int> nextGreaterElement(vector<int>& findNums, vector<int>& nums) {
map<int, int> maps;
vector<int> result;
for(int i = nums.size() - 1; i >= 0; --i){
if (i == nums.size() - 1){
maps[nums[i]] = -1;
} else{
if (nums[i+1] > nums[i])
maps[nums[i]] = nums[i+1];
else if (maps[nums[i+1]] > nums[i])
maps[nums[i]] = maps[nums[i+1]];
else if (maps[nums[i+1]] != -1){
int j = i + 1;
while(j < nums.size()){
if (nums[j] > nums[i]){
maps[nums[i]] = nums[j];
break;
}
++j;
}
if (j == nums.size())
maps[nums[i]] = -1;
}
else
maps[nums[i]] = -1;
}
}
for(auto num : findNums){
result.push_back(maps[num]);
}
return result;
}
500. Keyboard Row
Given a List of words, return the words that can be typed using letters of alphabet on only one row’s of American keyboard like the image below.
Example:
Input: ["Hello", "Alaska", "Dad", "Peace"]
Output: ["Alaska", "Dad"]
Solution in C++:
关键点:
- 字母对应关系
思路:
- 这里也是一个很简单的想法,就是在键盘中一行的字母对应同一个value值,然后构造一下查找的数组表即可。
vector<string> findWords(vector<string>& words) {
vector<string> result;
vector<int> maps(26, 0);
// 初始化表
maps['Q' - 'A'] = 1;
maps['W' - 'A'] = 1;
maps['E' - 'A'] = 1;
maps['R' - 'A'] = 1;
maps['T' - 'A'] = 1;
maps['Y' - 'A'] = 1;
maps['U' - 'A'] = 1;
maps['I' - 'A'] = 1;
maps['O' - 'A'] = 1;
maps['P' - 'A'] = 1;
maps['A' - 'A'] = 2;
maps['S' - 'A'] = 2;
maps['D' - 'A'] = 2;
maps['F' - 'A'] = 2;
maps['G' - 'A'] = 2;
maps['H' - 'A'] = 2;
maps['J' - 'A'] = 2;
maps['K' - 'A'] = 2;
maps['L' - 'A'] = 2;
maps['Z' - 'A'] = 3;
maps['X' - 'A'] = 3;
maps['C' - 'A'] = 3;
maps['V' - 'A'] = 3;
maps['B' - 'A'] = 3;
maps['N' - 'A'] = 3;
maps['M' - 'A'] = 3;
for(auto word : words){
int i = 0;
int tmp = maps[toupper(word[i]) - 'A'];
bool flag = true;
for(; i < word.size(); ++i){
if (maps[toupper(word[i]) - 'A'] != tmp){
flag = false;
break;
}
}
if(flag)
result.push_back(word);
}
return result;
}
小结
今天leetcode界面换新了,我很不习惯啊,熟悉花了一点时间,在别扭中实在习惯不了,就切回来了。哈哈。不过今天也是练练手。找找自己的逻辑bug。之前有提到感觉没什么进步,今天走在路上突然想通了,我缺少一些逻辑上的锻炼,刷题也是一个不错的锻炼。(自己可以想到一些解决的途径但是无法code出来,大问题!!!)