版权声明:本文为博主原创文章,转载请注明出处 https://blog.csdn.net/qq_23934475/article/details/83008529
设计模式之享元模式(Flyweight)
本篇为https://github.com/iluwatar/java-design-patterns/tree/master/flyweight阅读笔记
场景
炼金术士的商店里摆满了魔法药水。许多药水是相同的,因此不需要为每个药水创建新的对象。相反,一个对象实例可以表示多个货架项目,因此内存占用量仍然很小
它用于通过尽可能多地与类似对象共享来最小化内存使用或计算开销。
药水接口
public interface Potion {
void drink();
}
解药
public class HealPotion implements Potion {
@Override
public void drink() {
System.out.println("drink heal potion. @" + System.identityHashCode(this));
}
}
毒药
public class PoisonPotion implements Potion {
@Override
public void drink() {
System.out.println("drink poison potion. @" + System.identityHashCode(this));
}
}
圣水
public class HolyWaterPotion implements Potion {
@Override
public void drink() {
System.out.println("drink holy water potion. @" + System.identityHashCode(this));
}
}
药厂
public class PotionFactory {
enum PotionType {
POISON, HEAL, HOLY_WATER;
}
public PotionFactory() {
this.potions = new EnumMap<>(PotionType.class);
}
private final Map<PotionType, Potion> potions;
public Potion createPotion(PotionType type) {
Potion potion = potions.get(type);
if (potion == null) {
switch (type) {
case HEAL:
potion = new HealPotion();
potions.put(type,potion);
break;
case POISON:
potion = new PoisonPotion();
potions.put(type,potion);
break;
case HOLY_WATER:
potion = new HolyWaterPotion();
potions.put(type,potion);
break;
default:
break;
}
}
return potion;
}
}
药房
public class Shop {
private List<Potion> topShelf;
private List<Potion> bottomShelf;
public Shop() {
topShelf = new ArrayList<>();
bottomShelf = new ArrayList<>();
fillShelf();
}
private void fillShelf() {
PotionFactory factory = new PotionFactory();
topShelf.add(factory.createPotion(PotionFactory.PotionType.HEAL));
topShelf.add(factory.createPotion(PotionFactory.PotionType.HEAL));
topShelf.add(factory.createPotion(PotionFactory.PotionType.POISON));
topShelf.add(factory.createPotion(PotionFactory.PotionType.POISON));
topShelf.add(factory.createPotion(PotionFactory.PotionType.HOLY_WATER));
topShelf.add(factory.createPotion(PotionFactory.PotionType.HOLY_WATER));
bottomShelf.add(factory.createPotion(PotionFactory.PotionType.HEAL));
bottomShelf.add(factory.createPotion(PotionFactory.PotionType.HEAL));
bottomShelf.add(factory.createPotion(PotionFactory.PotionType.POISON));
bottomShelf.add(factory.createPotion(PotionFactory.PotionType.POISON));
bottomShelf.add(factory.createPotion(PotionFactory.PotionType.HOLY_WATER));
bottomShelf.add(factory.createPotion(PotionFactory.PotionType.HOLY_WATER));
}
public void enumerate() {
System.out.println("===top===");
for (Potion potion : topShelf) {
potion.drink();
}
System.out.println("===bottom===");
for (Potion potion : bottomShelf) {
potion.drink();
}
}
}
测试
public class App {
@Test
public void flyweightTest(){
Shop shop = new Shop();
shop.enumerate();
}
}
输出
扫描二维码关注公众号,回复:
3614519 查看本文章
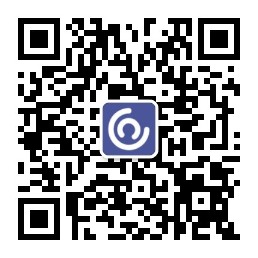
===top===
drink heal potion. @399573350
drink heal potion. @399573350
drink poison potion. @463345942
drink poison potion. @463345942
drink holy water potion. @195600860
drink holy water potion. @195600860
===bottom===
drink heal potion. @399573350
drink heal potion. @399573350
drink poison potion. @463345942
drink poison potion. @463345942
drink holy water potion. @195600860
drink holy water potion. @195600860
可以看到一共创建了三个对象
类图
flyweight是一个通过与其他类似对象共享尽可能多的数据来最小化内存使用的对象; 当简单的重复表示使用不可接受的内存量时,它是一种大量使用对象的方法。