一、http模块
1、http模块简介
http模块是Node提供的一个系统模块,使用该模块可以让我们根据自己的需求创建一个web服务器并定制服务器功能。
2、构建web服务器步骤
① 加载http模块
const http = require('http');
② 创建服务器实例
const server = http.createServer();
③ 开启服务器
参数一:监听的端口号,0——65535
参数二:启动成功后触发的回调函数
server.listen(3000, function () {
console.log('Server is running');
})
前三步都是固定的套路,记住就行了。
④ 注册服务器功能
参数一:事件类型,request代表监听浏览器请求
参数二:回调函数。参数一:请求对象;参数二:响应对像
server.on('request', function (req, res) {
//end能够将参数内容返回给浏览器
res.end('Hello Nodejs');
})
3、不同的url地址响应不同的内容
req.url内部保存了当前请求的url地址,该地址是一个没有域名的路径,判断url地址,通过不同的url地址来响应不同的内容。
const http = require('http');
const server = http.createServer();
server.listen(3000, function () {
console.log('Server is running');
})
server.on('request', function (req, res) {
const url = req.url;
if(url === '/' || url === '/index') {
res.setHeader('content-type', 'text/html;charset=utf-8');
res.end('前台首页');
} else if (url === '/admin/login') {
res.writeHeader(200, {
'content-type': 'text/html;charset=utf-8'
})
res.write('后台登录页');
res.end();
} else {
res.writeHeader(404);
res.end('404 not found');
}
})
每次代码改变就要重启服务器(Ctrl+shift+c)
4、req对象
① req.url:保存了当前请求的url地址
② req.method:保存了当前请求的方法
③ req.headers:保存了当前请求头的信息
5、res对象
① res.setHeader():设置响应头
② res.writeHeader():设置响应状态码和响应头,对象形式一次性可以设置多个响应头
③ res.write():设置响应主体
④ res.end():将响应行、响应头、响应体一次性返回给浏览器
6、http配合fs显示页面
//加载http模块
const http = require('http');
const fs = require('fs');
//创建服务器实例
const server = http.createServer();
//开启服务器
server.listen(3000, function () {
console.log('Server is running');
})
//注册服务器事件
server.on('request', function (req, res) {
const url = req.url;
//在view文件夹中新建了前台首页index.html,在view中新建了文件夹admin,在admin中新建了后台登录页面login.html
if(url === '/' || url === '/index') {
fs.readFile('./view/index.html', function (err,data) {
if (err) {
res.end('404 not found');
return console.log(err);
}
res.end(data);
});
} else if (url === '/admin/login') {
fs.readFile('./view/admin/login.html', function (err, data) {
if (err) {
res.end('404 not fount');
}
res.end(data);
});
} else {
res.writeHeader(404);
res.end('404 not found');
}
})
7、加载静态资源
分析url地址和要读取文件路径的关系
const http = require('http');
const fs = require('fs');
const server = http.createServer();
server.listen(3000, function () {
console.log('Server is running');
})
server.on('request', function (req, res) {
const url = req.url;
if(url === '/' || url === '/index') {
fs.readFile('./view/index.html', function (err,data) {
if (err) {
res.end('404 not found');
return console.log(err);
}
res.end(data);
});
} else if (url === '/admin/login') {
fs.readFile('./view/admin/login.html', function (err, data) {
if (err) {
res.end('404 not fount');
}
res.end(data);
});
//在view中创建了public文件夹,里面又分别创建了css、img、js三个文件夹,里面分别有a.css,1.png,b.js,这些都是要加载的静态资源
//为了加载静态资源,多加的一个分支
} else if (url.startsWith('/public')) {
fs.readFile('./view' + url, function (err,data) {
if (err) {
res.end('404 not found');
return console.log(err);
}
res.end(data);
});
} else {
res.writeHeader(404);
res.end('404 not found');
}
})
二、url模块
1、url模块简介
url也是node提供的系统模块,使用该模块能够将get地址分解,并提取出其中的参数。
get地址: /getData?id=10001&name=zs
核心方法: parse(var1, var2);
参数1: url地址
参数2: true(get参数转为对象) 、 false(get参数就是一个字符串)
扫描二维码关注公众号,回复:
4553168 查看本文章
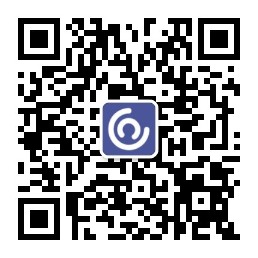
两个属性最重要:
pathname:当前请求的地址
query: get传递的参数
//1. 加载 http 模块
const http = require('http');
const url = require('url');
//2. 创建服务器实例
const server = http.createServer();
//3. 启动服务器
server.listen(3000, () => {
console.log('Server is running');
})
server.on('request', (req, res) => {
const urlObj = url.parse(req.url, true);
console.log(urlObj);
})