题目链接:https://leetcode.com/problems/binary-tree-zigzag-level-order-traversal/
思路:
仍然用队列,只不过在每一层遍历时,通过flag来确定是插入LinkedList尾部还是头部。
队列的遍历仍然是从左向右。这次用的是LinkedList而不是ArrayList保存每一层。
LinkedList是双向链表
比如:[1,2,3,4,null,null,5]
对于节点1,入队,然后从左向右(插入到LinkedList尾部),2,3入队。
到下一层,访问队列节点的顺序仍然是先2在3,但是在LinkedList中依次在头部插入,
先2在3,形成了[3,2]依次类推。更新flag,完成锯齿形遍历。
AC 0ms 100% Java:
扫描二维码关注公众号,回复:
5794629 查看本文章
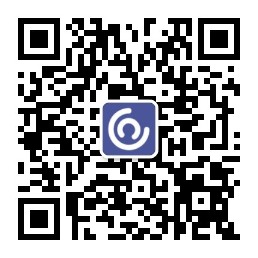
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
public List<List<Integer>> zigzagLevelOrder(TreeNode root) {
List<List<Integer>> ans=new ArrayList();
if(root==null)
return ans;
Queue<TreeNode> queue=new LinkedList();
queue.offer(root);
boolean flag=false;
while(!queue.isEmpty()){
LinkedList<Integer> list=new LinkedList();
int len=queue.size();
for(int i=0;i<len;i++){
TreeNode temp=queue.poll();
if(!flag)
list.add(temp.val);
else
list.addFirst(temp.val);
if(temp.left!=null)
queue.offer(temp.left);
if(temp.right!=null)
queue.offer(temp.right);
}
ans.add(list);
flag=!flag;
}
return ans;
}
}