Android中实现拍照有两种方法,一种是调用系统自带的相机,然后使用其返回的照片数据。 还有一种是自己用Camera类和其他相关类实现相机功能,这种方法定制度比较高,洗染也比较复杂,一般平常的应用只需使用第一种即可。
用Intent启动相机的代码:
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); startActivityForResult(intent, 1);
要将图像存储到SD卡上之前,最好检查一下SD卡是否可用
String sdStatus = Environment.getExternalStorageState(); if (!sdStatus.equals(Environment.MEDIA_MOUNTED)) { // 检测sd是否可用 Log.v("TestFile", "SD card is not avaiable/writeable right now."); return; }
另外,要注意的是读写SD卡必须在Mainifest.xml文件中配置权限:
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> <uses-permission android:name="android.permission.MOUNT_UNMOUNT_FILESYSTEMS" />
一个示例如下:
扫描二维码关注公众号,回复:
702713 查看本文章
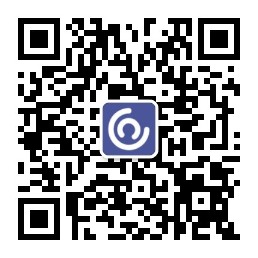
MainActivity.java:
package com.example.testand; import java.io.File; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import android.os.Bundle; import android.os.Environment; import android.provider.MediaStore; import android.app.Activity; import android.content.Intent; import android.graphics.Bitmap; import android.util.Log; import android.view.Menu; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.ImageView; public class MainActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button button=(Button) findViewById(R.id.button); button.setOnClickListener(new OnClickListener(){ @Override public void onClick(View v) { Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); startActivityForResult(intent, 1); } }); } protected void onActivityResult(int requestCode, int resultCode, Intent data){ super.onActivityResult(requestCode, resultCode, data); String sdStatus = Environment.getExternalStorageState(); if (!sdStatus.equals(Environment.MEDIA_MOUNTED)) { // 检测sd是否可用 Log.v("TestFile","SD card is not avaiable/writeable right now."); return; } Bundle bundle = data.getExtras(); Bitmap bitmap = (Bitmap) bundle.get("data");// 获取相机返回的数据,并转换为Bitmap图片格式 File file = new File("/sdcard/myImage/"); FileOutputStream bout = null; file.mkdirs();// 创建文件夹 String fileName = "/sdcard/myImage/111.jpg"; try { bout = new FileOutputStream(fileName); bitmap.compress(Bitmap.CompressFormat.JPEG, 100, bout);// 把数据写入文件 } catch (FileNotFoundException e) { e.printStackTrace(); } finally { try { bout.flush(); bout.close(); } catch (IOException e) { e.printStackTrace(); } } ((ImageView) findViewById(R.id.imageView)).setImageBitmap(bitmap);// 将图片显示在ImageView里 } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.main, menu); return true; } }
activity_main.xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".MainActivity" > <Button android:id="@+id/button" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="点击启动相机" /> <ImageView android:id="@+id/imageView" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_alignLeft="@+id/button" android:layout_alignParentBottom="true" android:layout_below="@+id/button" android:background="#999999" /> </RelativeLayout>
AndroidManifest.xml:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.testand" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" android:targetSdkVersion="17" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.example.testand.MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> <uses-permission android:name="android.permission.MOUNT_UNMOUNT_FILESYSTEMS" /> </manifest>