jQuery 是一个 JavaScript 函数库,兼容目前绝大部分的浏览器,概括来说包含如下功能:
- 元素选取
- 元素操作
- 样式设置
- 事件操作
- 过渡与动画
- AJAX
1、安装
(1)在 官网 中下载 jQuery,在项目中通过 <script> 标签引入
jQuery 提供两个版本可供下载,分别是 生产版本(用于实际上线) 和 开发版本(用于开发测试)
下载下来的 jQuery 是一个 JavaScript 文件,在项目中可以通过 <script> 标签引入
(2)直接通过 CDN 引入
也可以通过 CDN 引入 jQuery,这里以官方的 CDN 为例
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
2、语法
(1)基础语法
只要学习过 HTML、CSS、JavaScript 的朋友,一定会觉得 jQuery 的语法十分熟悉
一条独立的 jQuery 语句可以分为三个部分,$(selector).action()
$
:jQuery 的全局引用selector
:选择特定的 HTML 元素action
:对选择的元素进行操作
(2)执行入口
为了防止在文档完全加载之前运行 jQuery 代码,我们一般会把 jQuery 代码写在下面的回调函数中
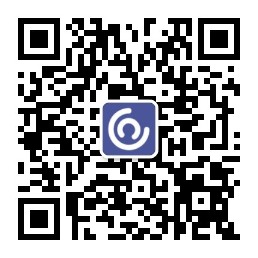
// 常规语法
$(document).ready(function(){
// 其它 jQuery 代码
})
// 简写语法
$(function(){
// 其它 jQuery 代码
})
3、元素选取
jQuery 可以用来选择特定的 HTML 元素,它与 CSS 选择器类似,但是对其做了一定的拓展
(1)CSS 选择器
这部分的语法与 CSS 选择器类似,常见的有 id 选择器、class 选择器等等,这里不多作介绍
<!DOCTYPE html>
<html>
<head>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
$(function(){
$("p").css("font-weight", "bold")
})
</script>
</head>
<body>
<p>Say Hello To Tomorrow</p>
<p>Say Goodbye To Yesterday</p>
</body>
</html>
(2)拓展
祖先元素
parent()
:返回选中元素的父亲元素parents()
:返回选中元素的所有祖先元素parentsUntil(<selector>)
:返回介于选中元素与给定元素之间的所有祖先元素
后代元素
children()
:返回选中元素的所有孩子子元素find()
:返回选中元素的所有后代元素
兄弟元素
siblings()
:返回选中元素的所有兄弟元素next()
/prev()
:返回选中元素的下 / 上一个兄弟元素nextAll()
/prevAll()
:返回选中元素之后 / 前的所有兄弟元素nextUntil(<selector>)
/prevUntil(<selector>)
:返回介于选中元素与给定元素之间的所有兄弟元素
过滤元素
first()
:返回选中元素的第一个元素last()
:返回选中元素的最后一个元素eq(<index>)
:返回选中元素中带有指定索引的元素filter(<selector>)
:返回符合条件的元素not(<selector>)
:返回不符合条件的元素
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>元素选取</title>
<style>
.relationship * {
display: block;
color: gray;
border: 1px solid gray;
padding: 5px;
margin: 15px;
}
</style>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
$(function(){
$("#selected").parentsUntil("body").css({
"color" : "red",
"border": "1px solid red"
})
})
</script>
</head>
<body class="relationship">
<div>
祖父元素
<div>
其它元素
<div>其它元素</div>
<div>其它元素</div>
</div>
<div>
父亲元素
<div>之前的兄弟元素</div>
<div>上一个兄弟元素</div>
<div id="selected">
选中元素
<div>
孩子元素
<div>孙子元素</div>
<div>孙子元素</div>
</div>
<div>
孩子元素
<div>孙子元素</div>
</div>
</div>
<div>下一个兄弟元素</div>
<div>之后的兄弟元素</div>
</div>
</div>
</body>
</html>
4、元素操作
jQuery 提供了一系列操作 DOM 的方法,用于访问和操作元素及其属性
(1)访问和操作元素
text([<text>] | [<function>])
:访问或操作选中元素的文本内容html([<html>] | [<function>])
:访问或操作选中元素的内容val([<value>] | [<function>])
:访问或操作表单字段的值
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>访问和操作元素</title>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
$(function(){
$("#set_text").click(function(){
$("#to_change").text("change into text")
})
$("#set_html").click(function(){
$("#to_change").html("<b>change into html</b>")
})
})
</script>
</head>
<body>
<p id="to_change">waiting to change</p>
<button id="set_text">set text</button>
<button id="set_html">set html</button>
</body>
</html>
(2)访问和操作属性
prop(<property>[, <value>] | { <property>: <value>, ... })
:访问和操作选中元素的属性如果存在指定的属性,返回相应的值;如果没有指定的属性,返回 空字符串
attr(<attribute>[, <value>] | { <attribute>: <value>, ... })
:访问和操作选中元素的属性如果存在指定的属性,返回相应的值;如果没有指定的属性,返回 undefined
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>访问和操作属性</title>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
function selectAll(checkbox) {
// 注意,这里不能使用 attr,否则会有错误
// 因为使用 attr 将会返回 undefined
let isAll = $(checkbox).attr("checked")
$("input[name='fruit']").prop("checked", isAll)
}
</script>
</head>
<body>
<p>What fruit do you like to eat?</p>
<p><input type="checkbox" name="fruit" value="all" onclick="selectAll(this)" />All</p>
<p><input type="checkbox" name="fruit" value="apple" />Apple</p>
<p><input type="checkbox" name="fruit" value="banana" />Banana</p>
<p><input type="checkbox" name="fruit" value="cherry" />Cherry</p>
</body>
</html>
(3)添加元素
append(<text>|<html>)
:在选中元素的结尾添加内容(仍属于该元素)prepend(<text>|<html>)
:在选中元素的开头添加内容(仍属于该元素)after(<text>|<html>)
:在选中元素的后面添加内容(不属于该元素)before(<text>|<html>)
:在选中元素的前面添加内容(不属于该元素)
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>添加元素</title>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
// 在添加文本后,请打开控制台看看 DOM 结构,比较它们之间的区别
$(function(){
$("#add_text").click(function(){
$("#origin_text").before("<p>before oringin text</p>")
$("#origin_text").prepend("<p>prepend to oringin text</p>")
$("#origin_text").append("<p>append to oringin text</p>")
$("#origin_text").after("<p>after oringin text</p>")
})
})
</script>
</head>
<body>
<p id="origin_text">origin text</p>
<button id="add_text">add text</button>
</body>
</html>
(4)删除元素
remove()
:删除选中元素及其后代元素empty()
:删除选中元素的后代元素
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>删除元素</title>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
// 在删除元素后,请打开控制台看看 DOM 结构,比较它们之间的区别
$(function(){
$("#remove_elem").click(function(){
$("#to_remove").remove()
})
$("#empty_elem").click(function(){
$("#to_empty").empty()
})
})
</script>
</head>
<body>
<div id="to_remove">
waiting to remove
<p>la la la</p>
<p>bla bla bla</p>
</div>
<button id="remove_elem">remove element</button>
<br/><br/>
<div id="to_empty">
waiting to empty
<p>la la la</p>
<p>bla bla bla</p>
</div>
<button id="empty_elem">empty element</button>
</body>
</html>
5、样式设置
jQuery 也提供了操作样式的方法,用于设置元素的 class 和 style
(1)class
addClass(<class>)
:向选中元素添加 class,可以添加一个或多个removeClass(<class>)
:从选中元素删除 class,可以删除一个或多个toggleClass(<class>)
:对选中元素进行 添加 / 删除 class 的切换操作
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>操作 class</title>
<style>
.rectangle {
width: 100px;
height: 80px;
cursor: pointer;
}
.red-color {
background-color: rgb(255, 0, 0);
}
.green-color {
background-color: rgb(0, 255, 0);
}
.blue-color {
background-color: rgb(0, 0, 255);
}
</style>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
$(function(){
let currColorIndex = 0
let nextColorIndex = 1
let indexColorMapping = {
0: "red-color",
1: "green-color",
2: "blue-color",
}
let colorNum = Object.keys(indexColorMapping).length
$("#primary_color").click(function(){
$("#primary_color").removeClass(indexColorMapping[currColorIndex])
$("#primary_color").addClass(indexColorMapping[nextColorIndex])
currColorIndex = nextColorIndex
nextColorIndex = (nextColorIndex + 1) % colorNum
})
})
</script>
</head>
<body>
<div id="primary_color" class="rectangle red-color"></div>
</body>
</html>
(2)style
css(<property>[, <value>] | { <property>: <value>, ... })
:访问或设置选中元素的 style
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>操作 style</title>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
$(function(){
let interval = 10
$("#larger").click(function(){
let font_size_string = $("#para").css("font-size").trim("px")
let font_size_number = parseInt(font_size_string)
let larger_font_size = (font_size_number + interval) + "px"
$("#para").css("font-size", larger_font_size)
})
})
</script>
</head>
<body>
<div id="para" style="font-size: 10px;">这是一段文字</div>
<br/><br/><button id="larger">larger</button>
</body>
</html>
(3)size
width()
:访问或设置元素的宽度(width)height()
:访问或设置元素的高度(height)innerWidth()
:访问或设置元素的内宽度(width + padding)innerHeight()
:访问或设置元素的内高度(height + padding)outerWidth()
:访问或设置元素的外宽度(width + padding + border)outerHeight()
:访问或设置元素的外高度(height + padding + border)outerWidth(true)
:访问或设置元素的外宽度(width + padding + border + margin)outerHeight(true)
:访问或设置元素的外高度(height + padding + border + margin)
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>操作 size</title>
<style>
.rectangle {
display: block;
width: 100px;
height: 80px;
margin: 20px 30px;
padding: 20px 30px;
border: 5px solid deeppink;
background-color: cornflowerblue;
}
</style>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
$(function(){
$("#rectangle_width").text(function(index, oldText){
return oldText + $(".rectangle").width() + "px"
})
$("#rectangle_height").text(function(index, oldText){
return oldText + $(".rectangle").height() + "px"
})
$("#rectangle_inner_width").text(function(index, oldText){
return oldText + $(".rectangle").innerWidth() + "px"
})
$("#rectangle_inner_height").text(function(index, oldText){
return oldText + $(".rectangle").innerHeight() + "px"
})
$("#rectangle_outer_width").text(function(index, oldText){
return oldText + $(".rectangle").outerWidth() + "px"
})
$("#rectangle_outer_height").text(function(index, oldText){
return oldText + $(".rectangle").outerHeight() + "px"
})
$("#rectangle_outer_width_true").text(function(index, oldText){
return oldText + $(".rectangle").outerWidth(true) + "px"
})
$("#rectangle_outer_height_true").text(function(index, oldText){
return oldText + $(".rectangle").outerHeight(true) + "px"
})
})
</script>
</head>
<body>
<div class="rectangle"></div>
<p id="rectangle_width">rectangle width: </p>
<p id="rectangle_height">rectangle height: </p>
<p id="rectangle_inner_width">rectangle inner width: </p>
<p id="rectangle_inner_height">rectangle inner height: </p>
<p id="rectangle_outer_width">rectangle outer width: </p>
<p id="rectangle_outer_height">rectangle outer height: </p>
<p id="rectangle_outer_width_true">rectangle outer width true: </p>
<p id="rectangle_outer_height_true">rectangle outer height true: </p>
</body>
</html>
6、事件操作
在 jQuery 中,对于大多数的事件操作都有一个等效的 jQuery 方法
(1)鼠标事件
click(<callback>)
:点击选中元素dbclick(<callback>)
:双击选中元素mouseenter(<callback>)
:鼠标移入选中元素mouseleave(<callback>)
:鼠标移出选中元素hover(<mouseenter>[, <mouseleave>])
:鼠标悬浮在选中元素
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>鼠标事件</title>
<style>
.wrapper {
width: 260px;
height: 80px;
background-color: black;
display: flex;
flex-direction: row;
justify-content: center;
align-items: center;
}
.content {
width: 240px;
height: 60px;
display: flex;
flex-direction: row;
justify-content: center;
align-items: center;
}
</style>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
$(function(){
$(".content").css({
"color": "ghostwhite",
"border": "1px solid rgba(255, 255, 255, 0.5)"
}).mouseenter(function(){
$(".content").css({
"color": "white",
"text-shadow": "1px 1px 2px ghostwhite",
"border": "1px solid rgba(255, 255, 255, 1)",
"box-shadow": "5px 5px 50px rgba(255, 255, 255, 0.4) inset"
})
}).mouseleave(function(){
$(".content").css({
"color": "ghostwhite",
"text-shadow": "none",
"border": "1px solid rgba(255, 255, 255, 0.5)",
"box-shadow": "none"
})
})
})
</script>
</head>
<body>
<div class="wrapper">
<div class="content">Hello World</div>
</div>
</body>
</html>
(2)键盘事件
keypress(<callback>)
:按下键盘的某一按键,并且产生一个字符时触发keydown(<callback>)
:按下键盘的某一按键时触发keyup(<callback>)
:松开键盘的某一按键时触发
(3)表单事件
submit(<callback>)
:提交表单change(<callback>)
:当选中元素的值发生改变focus(<callback>)
:当选中元素获得焦点blur(<callback>)
:当选中元素失去焦点
(4)文档 / 窗口事件
resize(<callback>)
:调整浏览器窗口大小scroll(<callback>)
:滚动指定元素
7、过渡与动画
在 jQuery 中,也可以处理过渡与动画效果
(1)显示隐藏
hide([<speed>[, <callback>]])
:隐藏选中元素show([<speed>[, <callback>]])
:显示选中元素toggle([<speed>[, <callback>]])
:切换hide()
和show()
方法隐藏或显示选中元素
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>显示隐藏</title>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
$(function(){
$("#show_or_hide").click(function(){
$("#para").toggle()
})
})
</script>
</head>
<body>
<p id="para">这是一段文本</p>
<button id="show_or_hide">Show / Hide</button>
</body>
</html>
(2)淡入淡出
fadeIn([<speed>[, <callback>]])
:淡入隐藏的选中元素fadeOut([<speed>[, <callback>]])
:淡出可见的选中元素fadeToggle([<speed>[, <callback>]])
:切换fadeIn()
和fadeOut()
方法淡入或淡出选中元素fadeTo(<speed>, <opacity>[, <callback>])
:为指定元素设置不透明度
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>淡入淡出</title>
<style>
.circle {
width: 100px;
height: 100px;
border-radius: 50px;
background-color: cornflowerblue;
}
</style>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
$(function(){
setInterval(function(){
$(".circle").fadeIn(1000).fadeOut(500)
}, 1500)
})
</script>
</head>
<body>
<div class="circle"></div>
</body>
</html>
(3)滑动
slideDown(<speed>[, <callback>])
:向下滑动选中元素slideUp(<speed>[, <callback>])
:向上滑动选中元素slideToggle(<speed>[, <callback>])
:切换slideDown()
和slideUp()
方法向下或向上滑动选中元素
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>滑动</title>
<style>
.announcement {
width: 200px;
height: 20px;
background-color: deepskyblue;
text-align: center;
cursor: pointer;
}
.content {
width: 200px;
height: 80px;
background-color: lightskyblue;
display: none;
}
</style>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
$(function(){
$(".announcement").click(function(){
$(".content").slideToggle(750)
})
})
</script>
</head>
<body>
<div class="announcement">announcement</div>
<div class="content"></div>
</body>
</html>
(4)动画
animate({<property>: <value>, ...}[, <speed>[, <callback>]])
:为选中元素设置自定义动画
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>动画</title>
<style>
.circle {
width: 200px;
height: 200px;
border-radius: 100px;
background-color: cornflowerblue;
font-size: 20px;
display: flex;
flex-direction: row;
justify-content: center;
align-items: center;
cursor: pointer;
}
</style>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
$(function(){
$("#announcement").click(function(){
$("#announcement").animate({
"width": "250px",
"height": "250px",
"border-radius": "125px"
}).animate({
"font-size": "25px"
})
})
})
</script>
</head>
<body>
<div id="announcement" class="circle">announcement</div>
</body>
</html>
8、AJAX
load(<URL>, <data>, <callback>)
:从服务器加载数据,并把返回的数据放入选中元素get(<URL>, <callback>)
:从指定资源请求数据post(<URL>, <data>, <callback>)
:向指定资源提交数据
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>AJAX</title>
<script src="http://code.jquery.com/jquery-3.4.1.min.js"></script>
<script>
$(function(){
$("#get_data").click(function(){
$.post("http://www.httpbin.org/post", {
"username": "admin",
"password": "123456"
}, function(data, status){
console.log(data)
$("#response_status").text(function(index, oldText){
return oldText + status
})
})
})
})
</script>
</head>
<body>
<button id="get_data">get data</button>
<p id="response_status">response status: </p>
</body>
</html>
【 阅读更多 JavaScript 系列文章,请看 JavaScript学习笔记 】